How to Create User-Defined Custom Exception in Java
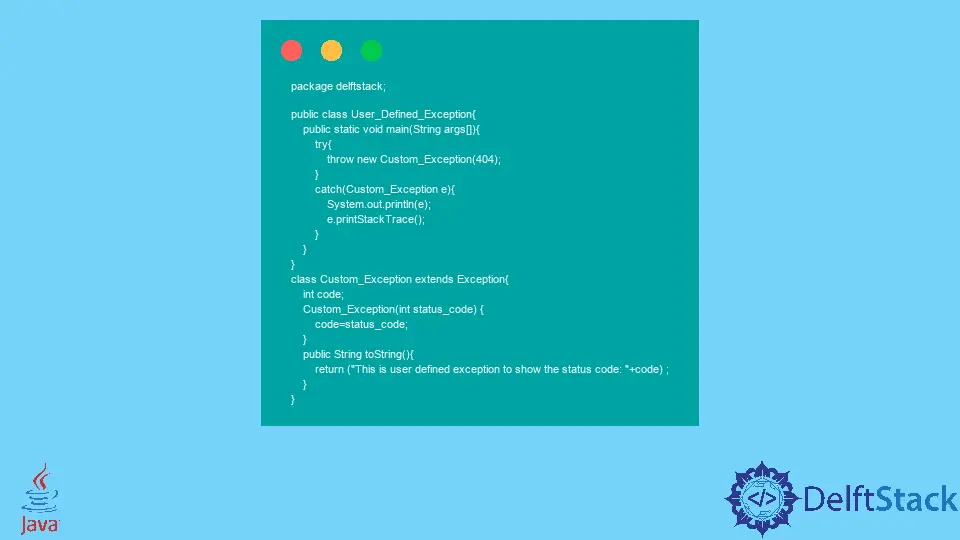
Other than pre-defined exceptions like NullPointerException or ArithmeticException, we can create our user-defined custom exceptions in Java. The Throw
keyword and try-catch
blocks make custom user-defined exceptions.
This tutorial demonstrates how to create custom user-defined exceptions in Java.
User-Defined Exception in Java
The pre-defined Java exception covers almost all the exceptions in the programs, but sometimes it is required to create our exception. The user-defined exception catches and provides specific treatment to a subset of pre-defined Java exceptions.
This exception can also be the business logic exceptions related to the workflow and business logic. To create custom user-defined exceptions, we must understand the exact problem first.
Let us try an example for user-defined exceptions. To create the user-defined exception, first, we need to extend the Exception class from Java.lang.
Example:
package delftstack;
public class User_Defined_Exception {
public static void main(String args[]) {
try {
throw new Custom_Exception(404);
} catch (Custom_Exception e) {
System.out.println(e);
e.printStackTrace();
}
}
}
class Custom_Exception extends Exception {
int code;
Custom_Exception(int status_code) {
code = status_code;
}
public String toString() {
return ("This is user defined exception to show the status code: " + code);
}
}
This code will throw a user-defined string exception.
Output:
This is user defined exception to show the status code: 404
This is user defined exception to show the status code: 404
at delftstack.User_Defined_Exception.main(User_Defined_Exception.java:6)
User-Defined Exception to Check the Validity of an ID in Java
Let us try another example that will be more problem-centric, such as checking the validity of an ID.
We will create an exception if the user enters an ID. If it is not present in the database, the invalid ID exception is thrown.
Example:
package delftstack;
import java.util.*;
class InValid_ID extends Exception {
public InValid_ID(String ID) {
super(ID);
}
}
public class User_Defined_Exception {
// Method to find ID
static void find_ID(int input_array[], int ID) throws InValid_ID {
boolean condition = false;
for (int i = 0; i < input_array.length; i++) {
if (ID == input_array[i]) {
condition = true;
}
}
if (!condition) {
throw new InValid_ID("The ID is you Entered is InValid!");
} else {
System.out.println("The ID is you Entered is Valid!");
}
}
public static void main(String[] args) {
Scanner new_id = new Scanner(System.in);
System.out.print("Enter the ID number: ");
int ID = new_id.nextInt();
try {
int Input_Array[] = new int[] {123, 124, 134, 135, 145, 146};
find_ID(Input_Array, ID);
} catch (InValid_ID e) {
System.out.println(e);
e.printStackTrace();
}
}
}
The code above creates an invalid ID exception. It will throw an exception if the user inputs the wrong ID.
Invalid output:
Enter the ID number: 133
delftstack.InValid_ID: The ID is you Entered is InValid!
delftstack.InValid_ID: The ID is you Entered is InValid!
at delftstack.User_Defined_Exception.find_ID(User_Defined_Exception.java:19)
at delftstack.User_Defined_Exception.main(User_Defined_Exception.java:32)
Valid output:
Enter the ID number: 145
The ID is you Entered is Valid!
Sheeraz is a Doctorate fellow in Computer Science at Northwestern Polytechnical University, Xian, China. He has 7 years of Software Development experience in AI, Web, Database, and Desktop technologies. He writes tutorials in Java, PHP, Python, GoLang, R, etc., to help beginners learn the field of Computer Science.
LinkedIn Facebook