Java에서 사용자 정의 사용자 정의 예외 생성
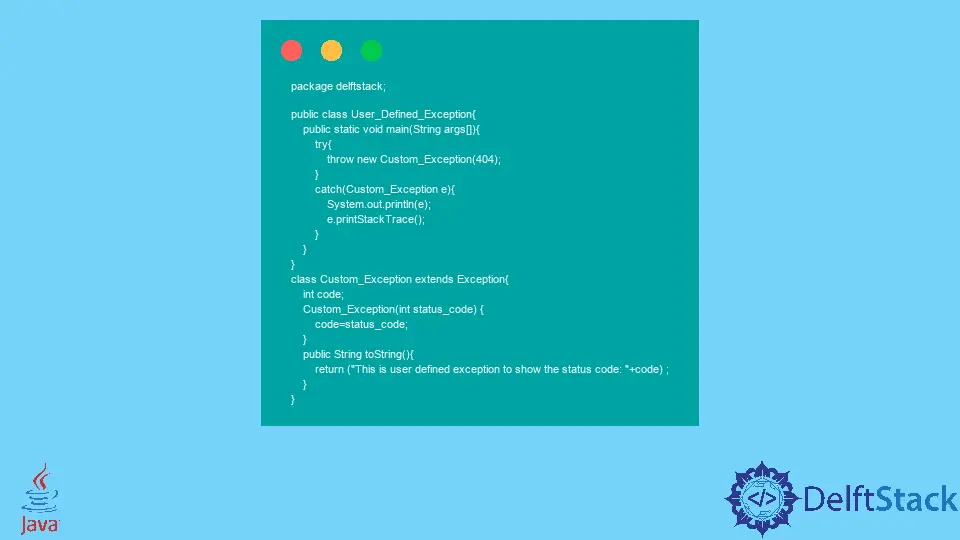
NullPointerException 또는 ArithmeticException과 같은 미리 정의된 예외 외에 Java에서 사용자 정의 사용자 정의 예외를 생성할 수 있습니다. Throw
키워드와 try-catch
블록은 사용자 정의 예외를 만듭니다.
이 자습서는 Java에서 사용자 정의 사용자 정의 예외를 생성하는 방법을 보여줍니다.
Java의 사용자 정의 예외
사전 정의된 Java 예외는 프로그램의 거의 모든 예외를 다루지만 때때로 예외를 생성해야 합니다. 사용자 정의 예외는 사전 정의된 Java 예외의 하위 집합을 포착하고 특정 처리를 제공합니다.
이 예외는 워크플로 및 비즈니스 논리와 관련된 비즈니스 논리 예외일 수도 있습니다. 사용자 정의 예외를 생성하려면 먼저 정확한 문제를 이해해야 합니다.
사용자 정의 예외에 대한 예를 시도해 보겠습니다. 사용자 정의 예외를 생성하려면 먼저 Java.lang에서 Exception 클래스를 확장해야 합니다.
예시:
package delftstack;
public class User_Defined_Exception {
public static void main(String args[]) {
try {
throw new Custom_Exception(404);
} catch (Custom_Exception e) {
System.out.println(e);
e.printStackTrace();
}
}
}
class Custom_Exception extends Exception {
int code;
Custom_Exception(int status_code) {
code = status_code;
}
public String toString() {
return ("This is user defined exception to show the status code: " + code);
}
}
이 코드는 사용자 정의 문자열 예외를 발생시킵니다.
출력:
This is user defined exception to show the status code: 404
This is user defined exception to show the status code: 404
at delftstack.User_Defined_Exception.main(User_Defined_Exception.java:6)
Java에서 ID의 유효성을 확인하기 위한 사용자 정의 예외
ID의 유효성을 확인하는 것과 같이 좀 더 문제 중심적인 다른 예를 시도해 보겠습니다.
사용자가 ID를 입력하면 예외를 생성합니다. 데이터베이스에 없으면 잘못된 ID 예외가 발생합니다.
예시:
package delftstack;
import java.util.*;
class InValid_ID extends Exception {
public InValid_ID(String ID) {
super(ID);
}
}
public class User_Defined_Exception {
// Method to find ID
static void find_ID(int input_array[], int ID) throws InValid_ID {
boolean condition = false;
for (int i = 0; i < input_array.length; i++) {
if (ID == input_array[i]) {
condition = true;
}
}
if (!condition) {
throw new InValid_ID("The ID is you Entered is InValid!");
} else {
System.out.println("The ID is you Entered is Valid!");
}
}
public static void main(String[] args) {
Scanner new_id = new Scanner(System.in);
System.out.print("Enter the ID number: ");
int ID = new_id.nextInt();
try {
int Input_Array[] = new int[] {123, 124, 134, 135, 145, 146};
find_ID(Input_Array, ID);
} catch (InValid_ID e) {
System.out.println(e);
e.printStackTrace();
}
}
}
위의 코드는 잘못된 ID 예외를 생성합니다. 사용자가 잘못된 ID를 입력하면 예외가 발생합니다.
잘못된 출력:
Enter the ID number: 133
delftstack.InValid_ID: The ID is you Entered is InValid!
delftstack.InValid_ID: The ID is you Entered is InValid!
at delftstack.User_Defined_Exception.find_ID(User_Defined_Exception.java:19)
at delftstack.User_Defined_Exception.main(User_Defined_Exception.java:32)
유효한 출력:
Enter the ID number: 145
The ID is you Entered is Valid!
Sheeraz is a Doctorate fellow in Computer Science at Northwestern Polytechnical University, Xian, China. He has 7 years of Software Development experience in AI, Web, Database, and Desktop technologies. He writes tutorials in Java, PHP, Python, GoLang, R, etc., to help beginners learn the field of Computer Science.
LinkedIn Facebook