在 Java 中建立使用者定義的自定義異常
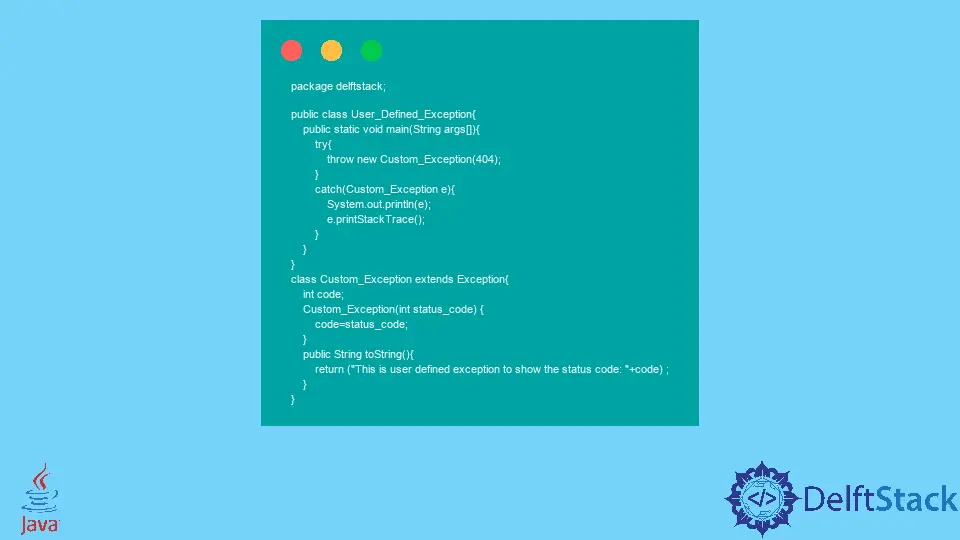
除了 NullPointerException 或 ArithmeticException 之類的預定義異常,我們還可以在 Java 中建立使用者定義的自定義異常。Throw
關鍵字和 try-catch
塊生成自定義使用者定義的異常。
本教程演示瞭如何在 Java 中建立自定義的使用者定義異常。
Java 中的使用者定義異常
預定義的 Java 異常幾乎涵蓋了程式中的所有異常,但有時需要建立我們的異常。使用者定義的異常捕獲併為預定義的 Java 異常的子集提供特定的處理。
該異常也可以是與工作流和業務邏輯相關的業務邏輯異常。要建立自定義的使用者定義異常,我們必須首先了解確切的問題。
讓我們嘗試一個使用者定義異常的示例。要建立使用者定義的異常,首先,我們需要從 Java.lang 擴充套件 Exception 類。
例子:
package delftstack;
public class User_Defined_Exception {
public static void main(String args[]) {
try {
throw new Custom_Exception(404);
} catch (Custom_Exception e) {
System.out.println(e);
e.printStackTrace();
}
}
}
class Custom_Exception extends Exception {
int code;
Custom_Exception(int status_code) {
code = status_code;
}
public String toString() {
return ("This is user defined exception to show the status code: " + code);
}
}
此程式碼將引發使用者定義的字串異常。
輸出:
This is user defined exception to show the status code: 404
This is user defined exception to show the status code: 404
at delftstack.User_Defined_Exception.main(User_Defined_Exception.java:6)
用於檢查 Java 中 ID 有效性的使用者定義異常
讓我們嘗試另一個更以問題為中心的示例,例如檢查 ID 的有效性。
如果使用者輸入 ID,我們將建立一個異常。如果資料庫中不存在,則丟擲無效 ID 異常。
例子:
package delftstack;
import java.util.*;
class InValid_ID extends Exception {
public InValid_ID(String ID) {
super(ID);
}
}
public class User_Defined_Exception {
// Method to find ID
static void find_ID(int input_array[], int ID) throws InValid_ID {
boolean condition = false;
for (int i = 0; i < input_array.length; i++) {
if (ID == input_array[i]) {
condition = true;
}
}
if (!condition) {
throw new InValid_ID("The ID is you Entered is InValid!");
} else {
System.out.println("The ID is you Entered is Valid!");
}
}
public static void main(String[] args) {
Scanner new_id = new Scanner(System.in);
System.out.print("Enter the ID number: ");
int ID = new_id.nextInt();
try {
int Input_Array[] = new int[] {123, 124, 134, 135, 145, 146};
find_ID(Input_Array, ID);
} catch (InValid_ID e) {
System.out.println(e);
e.printStackTrace();
}
}
}
上面的程式碼建立了一個無效的 ID 異常。如果使用者輸入了錯誤的 ID,它將引發異常。
無效的輸出:
Enter the ID number: 133
delftstack.InValid_ID: The ID is you Entered is InValid!
delftstack.InValid_ID: The ID is you Entered is InValid!
at delftstack.User_Defined_Exception.find_ID(User_Defined_Exception.java:19)
at delftstack.User_Defined_Exception.main(User_Defined_Exception.java:32)
有效輸出:
Enter the ID number: 145
The ID is you Entered is Valid!
Sheeraz is a Doctorate fellow in Computer Science at Northwestern Polytechnical University, Xian, China. He has 7 years of Software Development experience in AI, Web, Database, and Desktop technologies. He writes tutorials in Java, PHP, Python, GoLang, R, etc., to help beginners learn the field of Computer Science.
LinkedIn Facebook