在 Java 中處理 FileNotFoundException
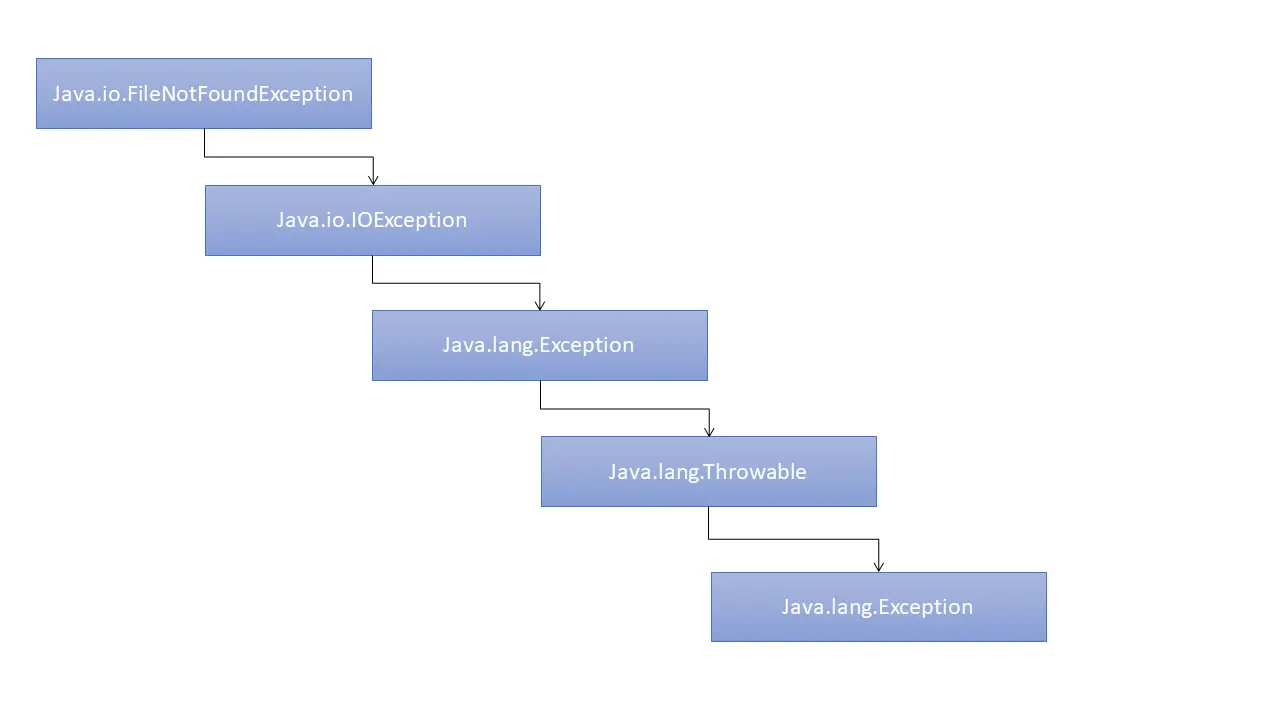
當我們嘗試訪問檔案時會發生 FileNotFoundException。它是 FileOutputStream、FileInputStream 和 RandomAccessFile 丟擲的 IO 異常的一部分,我們可以使用 try-catch
塊來處理這些異常。
本教程描述 FileNotFoundException 並演示如何處理 FileNotFoundException。
Java IO FileNotFoundException
當具有特定路徑名的檔案不存在或具有路徑名的檔案存在但我們可以出於某種原因訪問它時,會發生 FileNotFoundException。FileNotFoundException 擴充套件了 java.io.IOException,後者擴充套件了 java.lang.Exception,處理 try-catch
塊。
下圖展示了 FileNotFoundException
的結構。
讓我們執行一個示例來檢視 FileNotFoundException
:
package delftstack;
import java.io.File;
import java.util.*;
public class File_Not_Found_Exception {
public static void main(String[] args) throws Exception {
Scanner demo_file = new Scanner(new File("demo.txt"));
String demo_content = "";
demo_content = demo_file.nextLine();
}
}
檔案 demo.txt
不存在;程式碼將丟擲 FileNotFoundException。
輸出:
Exception in thread "main" java.io.FileNotFoundException: demo.txt (The system cannot find the file specified)
at java.base/java.io.FileInputStream.open0(Native Method)
at java.base/java.io.FileInputStream.open(FileInputStream.java:216)
at java.base/java.io.FileInputStream.<init>(FileInputStream.java:157)
at java.base/java.util.Scanner.<init>(Scanner.java:639)
at delftstack.File_Not_Found_Exception.main(File_Not_Found_Exception.java:8)
在 Java 中處理 FileNotFoundException
我們可以使用 try-catch
塊來處理 Java 中的 FileNotFoundException。下面的例子演示了 FileNotFoundException 的處理:
package delftstack;
import java.io.BufferedReader;
import java.io.File;
import java.io.FileReader;
import java.io.IOException;
public class File_Not_Found_Exception {
private static final String demo_file = "demo.txt";
public static void main(String[] args) {
BufferedReader file_read = null;
try {
// Open the file to read the content
file_read = new BufferedReader(new FileReader(new File(demo_file)));
// Reading the file content
String file_line = null;
while ((file_line = file_read.readLine()) != null) System.out.println(file_line);
} catch (IOException e) {
System.err.println("FileNotFoundException was caught!");
e.printStackTrace();
} finally {
try {
file_read.close();
} catch (IOException e) {
System.err.println("FileNotFoundException was caught!");
e.printStackTrace();
}
}
}
}
上面的程式碼處理異常。首先,它會嘗試開啟和讀取檔案,如果檔案不存在或不允許開啟和讀取,則會丟擲 FileNotFoundException。
輸出:
FileNotFoundException was caught!
java.io.FileNotFoundException: demo.txt (The system cannot find the file specified)
at java.base/java.io.FileInputStream.open0(Native Method)
at java.base/java.io.FileInputStream.open(FileInputStream.java:216)
at java.base/java.io.FileInputStream.<init>(FileInputStream.java:157)
at java.base/java.io.FileReader.<init>(FileReader.java:75)
at delftstack.File_Not_Found_Exception.main(File_Not_Found_Exception.java:16)
Exception in thread "main" java.lang.NullPointerException: Cannot invoke "java.io.BufferedReader.close()" because "file_read" is null
at delftstack.File_Not_Found_Exception.main(File_Not_Found_Exception.java:29)
Sheeraz is a Doctorate fellow in Computer Science at Northwestern Polytechnical University, Xian, China. He has 7 years of Software Development experience in AI, Web, Database, and Desktop technologies. He writes tutorials in Java, PHP, Python, GoLang, R, etc., to help beginners learn the field of Computer Science.
LinkedIn Facebook