在 Java 中处理 FileNotFoundException
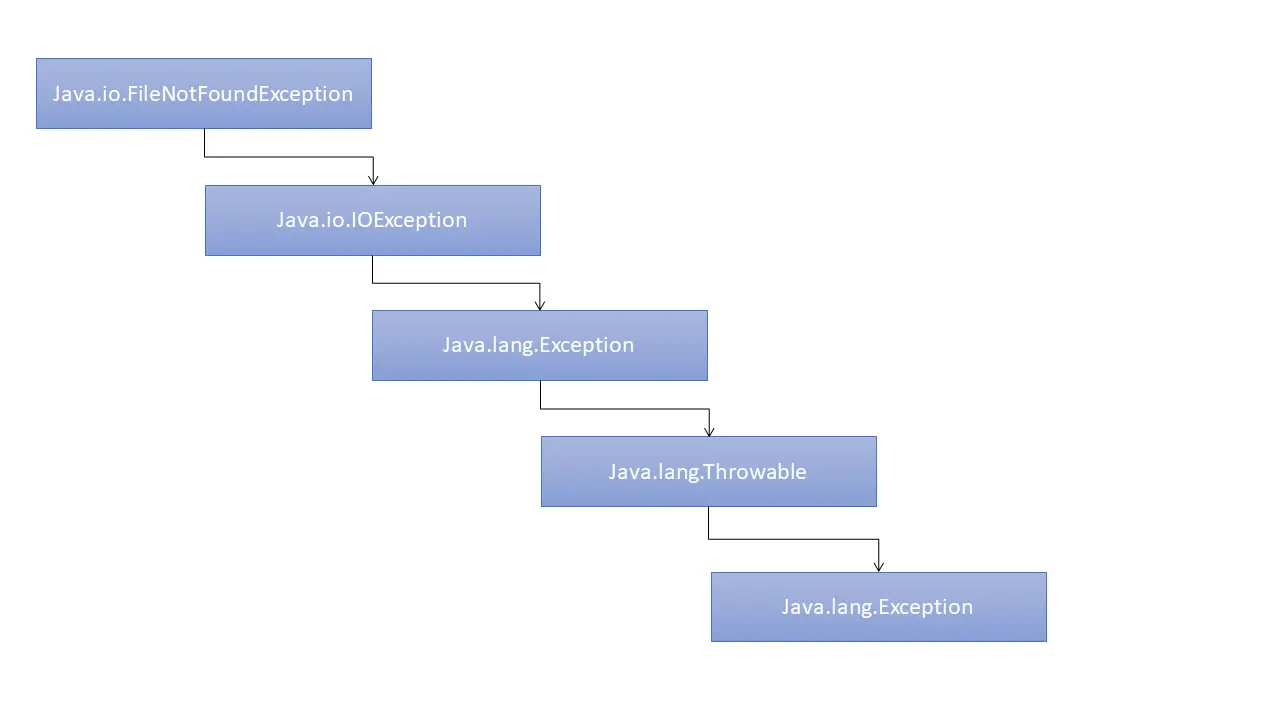
当我们尝试访问文件时会发生 FileNotFoundException。它是 FileOutputStream、FileInputStream 和 RandomAccessFile 抛出的 IO 异常的一部分,我们可以使用 try-catch
块来处理这些异常。
本教程描述 FileNotFoundException 并演示如何处理 FileNotFoundException。
Java IO FileNotFoundException
当具有特定路径名的文件不存在或具有路径名的文件存在但我们可以出于某种原因访问它时,会发生 FileNotFoundException。FileNotFoundException 扩展了 java.io.IOException,后者扩展了 java.lang.Exception,处理 try-catch
块。
下图展示了 FileNotFoundException
的结构。
让我们运行一个示例来查看 FileNotFoundException
:
package delftstack;
import java.io.File;
import java.util.*;
public class File_Not_Found_Exception {
public static void main(String[] args) throws Exception {
Scanner demo_file = new Scanner(new File("demo.txt"));
String demo_content = "";
demo_content = demo_file.nextLine();
}
}
文件 demo.txt
不存在;代码将抛出 FileNotFoundException。
输出:
Exception in thread "main" java.io.FileNotFoundException: demo.txt (The system cannot find the file specified)
at java.base/java.io.FileInputStream.open0(Native Method)
at java.base/java.io.FileInputStream.open(FileInputStream.java:216)
at java.base/java.io.FileInputStream.<init>(FileInputStream.java:157)
at java.base/java.util.Scanner.<init>(Scanner.java:639)
at delftstack.File_Not_Found_Exception.main(File_Not_Found_Exception.java:8)
在 Java 中处理 FileNotFoundException
我们可以使用 try-catch
块来处理 Java 中的 FileNotFoundException。下面的例子演示了 FileNotFoundException 的处理:
package delftstack;
import java.io.BufferedReader;
import java.io.File;
import java.io.FileReader;
import java.io.IOException;
public class File_Not_Found_Exception {
private static final String demo_file = "demo.txt";
public static void main(String[] args) {
BufferedReader file_read = null;
try {
// Open the file to read the content
file_read = new BufferedReader(new FileReader(new File(demo_file)));
// Reading the file content
String file_line = null;
while ((file_line = file_read.readLine()) != null) System.out.println(file_line);
} catch (IOException e) {
System.err.println("FileNotFoundException was caught!");
e.printStackTrace();
} finally {
try {
file_read.close();
} catch (IOException e) {
System.err.println("FileNotFoundException was caught!");
e.printStackTrace();
}
}
}
}
上面的代码处理异常。首先,它会尝试打开和读取文件,如果文件不存在或不允许打开和读取,则会抛出 FileNotFoundException。
输出:
FileNotFoundException was caught!
java.io.FileNotFoundException: demo.txt (The system cannot find the file specified)
at java.base/java.io.FileInputStream.open0(Native Method)
at java.base/java.io.FileInputStream.open(FileInputStream.java:216)
at java.base/java.io.FileInputStream.<init>(FileInputStream.java:157)
at java.base/java.io.FileReader.<init>(FileReader.java:75)
at delftstack.File_Not_Found_Exception.main(File_Not_Found_Exception.java:16)
Exception in thread "main" java.lang.NullPointerException: Cannot invoke "java.io.BufferedReader.close()" because "file_read" is null
at delftstack.File_Not_Found_Exception.main(File_Not_Found_Exception.java:29)
Sheeraz is a Doctorate fellow in Computer Science at Northwestern Polytechnical University, Xian, China. He has 7 years of Software Development experience in AI, Web, Database, and Desktop technologies. He writes tutorials in Java, PHP, Python, GoLang, R, etc., to help beginners learn the field of Computer Science.
LinkedIn Facebook