Java.Lang.IllegalMonitorStateException
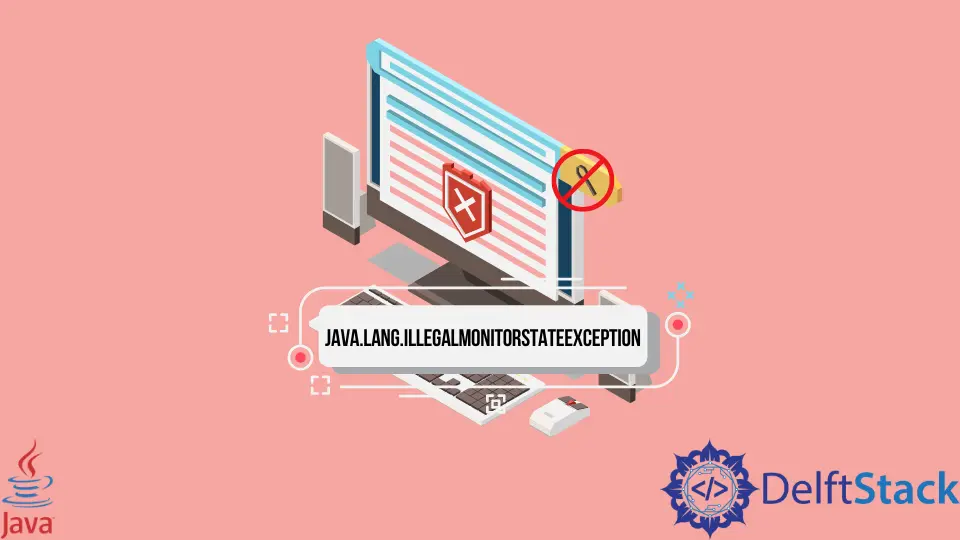
IllegalMonitorStateException
은 멀티스레딩 프로그래밍과 관련이 있습니다. 이 튜토리얼은 Java의 IllegalMonitorStateException
을 설명하고 시연합니다.
Java의 java.lang.IllegalMonitorStateException
IllegalMonitorStateException
은 Java에서 다중 스레딩 프로그래밍으로 작업할 때 발생합니다. 모니터에서 동기화하고 스레드가 해당 시간을 소유하지 않고 모니터에서 대기 중인 다른 스레드를 기다리거나 알리려고 하면 IllegalMonitorStateException
이 발생합니다.
synchronized
블록에 없는 object
클래스에서 wait()
, notify()
또는 notifyAll()
메서드를 호출하면 이 예외가 발생합니다. 이 시나리오에서 예를 들어 보겠습니다.
package delftstack;
class DemoClass implements Runnable {
public void run() {
try {
// The wait method is called outside the synchronized block
this.wait(100);
System.out.println("Thread can successfully run.");
} catch (InterruptedException ex) {
ex.printStackTrace();
}
}
}
public class Example {
public static void main(String[] args) {
DemoClass DemoRunnable = new DemoClass();
Thread DemoThread = new Thread(DemoRunnable);
DemoThread.start();
}
}
위의 코드는 Runnable
클래스를 구현하는 클래스를 생성한 다음 synchronized
블록 외부에서 wait
메소드를 호출합니다. Example
은 DemoClass
인스턴스에서 스레드를 생성합니다.
wait
메서드가 synchronized
블록 외부에서 호출되고 wait()
메서드를 호출하기 전에 스레드가 모니터에 대한 잠금을 소유해야 하기 때문에 IllegalMonitorStateException
이 발생합니다. 출력 참조:
Exception in thread "Thread-0" java.lang.IllegalMonitorStateException: current thread is not owner
at java.base/java.lang.Object.wait(Native Method)
at delftstack.DemoClass.run(Example.java:7)
at java.base/java.lang.Thread.run(Thread.java:833)
이 예외를 수정하려면 object
잠금을 획득한 후 wait()
, notify()
또는 notifyAll()
메서드를 호출해야 합니다. 이 잠금은 synchronized
블록에 있게 됩니다.
이제 wait()
메서드를 synchronized
블록에 넣은 다음 위의 코드를 오류 없이 만듭니다. 예를 참조하십시오:
package delftstack;
class DemoClass implements Runnable {
public void run() {
synchronized (this) {
try {
// The wait method is called outside the synchronized block
this.wait(100);
System.out.println("Thread can successfully run.");
} catch (InterruptedException ex) {
ex.printStackTrace();
}
}
}
}
public class Example {
public static void main(String[] args) {
DemoClass DemoRunnable = new DemoClass();
Thread DemoThread = new Thread(DemoRunnable);
DemoThread.start();
}
}
이제 wait()
메서드가 synchronized
블록 안에 있고 개체 모니터에 대한 잠금이 획득되면 코드가 성공적으로 실행됩니다. 출력 참조:
Thread can successfully run.
Sheeraz is a Doctorate fellow in Computer Science at Northwestern Polytechnical University, Xian, China. He has 7 years of Software Development experience in AI, Web, Database, and Desktop technologies. He writes tutorials in Java, PHP, Python, GoLang, R, etc., to help beginners learn the field of Computer Science.
LinkedIn Facebook