How to Get the Subset of an Array in Java
-
Get the Subset of an Array in Java Using
Arrays.copyOf()
-
Get the Subset of an Array in Java Using
Arrays.copyOfRange()
-
Get the Subset of an Array in Java Using
Stream.IntStream
-
Get the Subset of an Array in Java Using
System.arraycopy
- Get the Subset of an Array in Java Using Apache Commons Lang
- Get the Subset of an Array in Java Using List Conversion
- Get the Subset of an Array in Java Using a Custom Method
- Conclusion
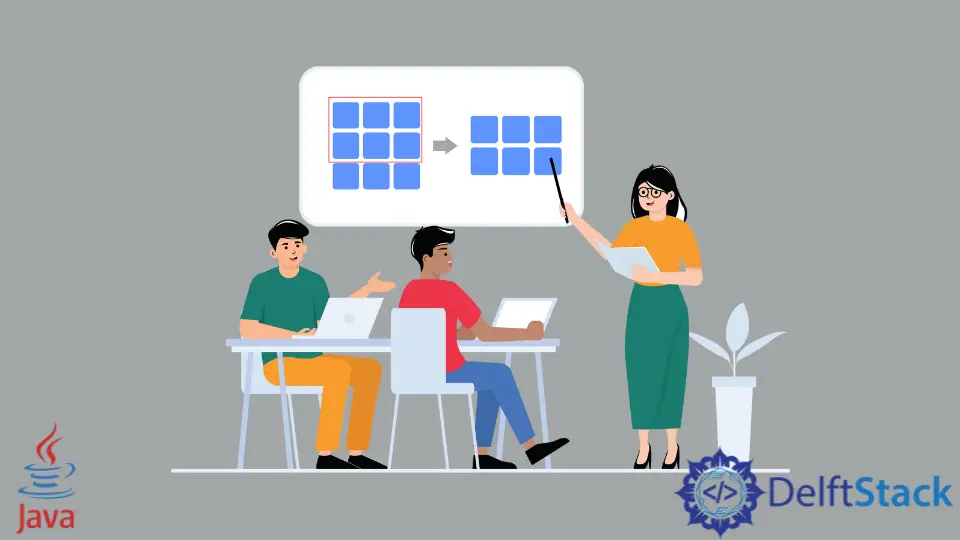
Manipulating arrays is a fundamental aspect of Java programming, and developers often encounter scenarios where extracting a subset of an array becomes crucial. Whether it’s for filtering specific elements, performing operations on a distinct portion, or optimizing memory usage, Java offers multiple approaches to efficiently obtain subsets of arrays.
In this article, we’ll explore various methods, from utilizing built-in functions like Arrays.copyOf()
to leveraging Java 8 streams and employing custom logic.
Get the Subset of an Array in Java Using Arrays.copyOf()
One way to extract a subset of elements based on specific criteria in Java is by using the Arrays.copyOf()
method, a convenient utility method provided by the java.util
package. This method allows you to create a new array containing elements from the original array within a specified range.
The syntax for the Arrays.copyOf()
method is as follows:
public static <T, U> T[] copyOf(U[] original, int newLength, Class<? extends T[]> newType)
It takes three parameters:
original
: The array to be copied.newLength
: The length of the copy.newType
: The class of the new array.
Let’s consider a practical example where we have an array of strings, and we want to obtain a subset of elements up to a certain index using the Arrays.copyOf()
method.
import java.util.Arrays;
public class ArraySubsetExample {
public static void main(String[] args) {
String[] originalArray = {"Apple", "Banana", "Orange", "Grapes", "Mango", "Pineapple"};
int endIndex = 3;
String[] subsetArray = Arrays.copyOf(originalArray, endIndex + 1, String[].class);
System.out.println("Original Array: " + Arrays.toString(originalArray));
System.out.println("Subset Array: " + Arrays.toString(subsetArray));
}
}
In this example, we start by importing the Arrays
class from the java.util
package. We declare an array named originalArray
containing strings. The variable endIndex
determines the endpoint of our subset, and we want to include elements up to this index.
The critical line of code is:
String[] subsetArray = Arrays.copyOf(originalArray, endIndex + 1, String[].class);
Here, Arrays.copyOf()
is called with three arguments: the original array (originalArray
), the new length (endIndex + 1
), and the class of the new array (String[].class
). This creates a new array containing elements from the original array up to the specified endpoint.
Finally, we print both the original and subset arrays to observe the result.
Code Output:
Original Array: [Apple, Banana, Orange, Grapes, Mango, Pineapple]
Subset Array: [Apple, Banana, Orange, Grapes]
In this output, the subset array successfully contains elements up to the specified index, demonstrating the effective use of the Arrays.copyOf()
method to obtain a subset of an array in Java.
Get the Subset of an Array in Java Using Arrays.copyOfRange()
In Java, another efficient way to obtain a subset of an array is by using the Arrays.copyOfRange()
method. This method, available in the java.util
package, allows you to copy a specified range of elements from the original array into a new array.
This is particularly useful when you want to extract a portion of the array based on specified start and end indexes.
The syntax for the Arrays.copyOfRange()
method is as follows:
public static <T> T[] copyOfRange(T[] original, int from, int to)
Parameters:
original
: The array to be copied.from
: The initial index (inclusive).to
: The final index (exclusive).
Let’s consider an example where we have an array of integers, and we want to extract a subset from index 2 to index 5 using the Arrays.copyOfRange()
method.
import java.util.Arrays;
public class ArraySubsetRangeExample {
public static void main(String[] args) {
int[] originalArray = {10, 20, 30, 40, 50, 60, 70};
int startIndex = 2;
int endIndex = 6;
int[] subsetArray = Arrays.copyOfRange(originalArray, startIndex, endIndex);
System.out.println("Original Array: " + Arrays.toString(originalArray));
System.out.println("Subset Array: " + Arrays.toString(subsetArray));
}
}
In this example, we import the Arrays
class and declare an array named originalArray
containing integers. We set the startIndex
to 2 and the endIndex
to 6, indicating that we want to include elements from index 2 up to, but not including, index 6 in our subset.
The key line of code is:
int[] subsetArray = Arrays.copyOfRange(originalArray, startIndex, endIndex);
Here, Arrays.copyOfRange()
is called with three arguments: the original array (originalArray
), the starting index (startIndex
), and the ending index (endIndex
). This creates a new array containing elements within the specified range.
Finally, we print both the original and subset arrays to observe the result.
Code Output:
Original Array: [10, 20, 30, 40, 50, 60, 70]
Subset Array: [30, 40, 50, 60]
In this output, the subset array successfully contains elements from index 2 to index 5, demonstrating the effective use of the Arrays.copyOfRange()
method to obtain a subset of an array in Java.
Get the Subset of an Array in Java Using Stream.IntStream
In Java 8 and later, the introduction of streams provides a concise and expressive way to obtain a subset of an array. The IntStream
interface, part of the java.util.stream
package, allows us to create a stream of integers within a specified range.
We can then convert this stream into an array, effectively extracting a subset of elements from the original array.
The syntax for obtaining a subset using Stream.IntStream
is as follows:
IntStream.range(int startInclusive, int endExclusive)
Parameters:
startInclusive
: The starting index (inclusive).endExclusive
: The ending index (exclusive).
Let’s consider an example where we have an array of doubles, and we want to extract a subset from index 1 to index 4 using the Stream.IntStream
method.
import java.util.Arrays;
import java.util.stream.IntStream;
public class ArraySubsetStreamExample {
public static void main(String[] args) {
double[] originalArray = {3.14, 2.71, 1.61, 4.13, 2.82, 0.77};
int startIndex = 1;
int endIndex = 5;
double[] subsetArray =
IntStream.range(startIndex, endIndex).mapToDouble(i -> originalArray[i]).toArray();
System.out.println("Original Array: " + Arrays.toString(originalArray));
System.out.println("Subset Array: " + Arrays.toString(subsetArray));
}
}
In this example, we import both the Arrays
class and IntStream
from java.util.stream
. We declare an array named originalArray
containing double values. The startIndex
is set to 1, and the endIndex
is set to 5, indicating that we want to include elements from index 1 up to, but not including, index 5 in our subset.
The crucial lines of code are:
double[] subsetArray =
IntStream.range(startIndex, endIndex).mapToDouble(i -> originalArray[i]).toArray();
Here, IntStream.range(startIndex, endIndex)
creates a stream of integer values within the specified range. The mapToDouble(i -> originalArray[i])
operation then maps each integer to the corresponding element in the original array, converting the stream to an IntStream
. Finally, toArray()
converts the IntStream
into an array of doubles.
Code Output:
Original Array: [3.14, 2.71, 1.61, 4.13, 2.82, 0.77]
Subset Array: [2.71, 1.61, 4.13, 2.82]
In this output, the subset array successfully contains elements from index 1 to index 4, demonstrating the effective use of the Stream.IntStream
method to obtain a subset of an array in Java.
Get the Subset of an Array in Java Using System.arraycopy
The System.arraycopy
method provides a low-level but efficient way to copy a subset of elements from one array to another. This method allows for greater control over the copying process by specifying the source and destination arrays along with the starting indexes and length of the copy.
The syntax for the System.arraycopy
method is as follows:
public static void arraycopy(Object src, int srcPos, Object dest, int destPos, int length)
Parameters:
src
: The source array.srcPos
: The starting position in the source array.dest
: The destination array.destPos
: The starting position in the destination array.length
: The number of array elements to copy.
Let’s consider an example where we have an array of characters, and we want to extract a subset from index 2 to index 6 using the System.arraycopy
method.
import java.util.Arrays;
public class ArraySubsetCopyExample {
public static void main(String[] args) {
char[] originalArray = {'a', 'b', 'c', 'd', 'e', 'f', 'g'};
int startIndex = 2;
int endIndex = 7;
int length = endIndex - startIndex;
char[] subsetArray = new char[length];
System.arraycopy(originalArray, startIndex, subsetArray, 0, length);
System.out.println("Original Array: " + Arrays.toString(originalArray));
System.out.println("Subset Array: " + Arrays.toString(subsetArray));
}
}
In this example, we have an array named originalArray
containing characters. We set the startIndex
to 2 and the endIndex
to 7, indicating that we want to include elements from index 2 up to, but not including, index 7 in our subset.
The crucial lines of code are:
int length = endIndex - startIndex;
char[] subsetArray = new char[length];
System.arraycopy(originalArray, startIndex, subsetArray, 0, length);
Here, we calculate the length of the subset (length
) by subtracting the startIndex
from the endIndex
. We then create a new array, subsetArray
, with the calculated length.
The System.arraycopy
method is called to copy elements from the originalArray
starting at the startIndex
to the subsetArray
starting at position 0, with the specified length.
Code Output:
Original Array: [a, b, c, d, e, f, g]
Subset Array: [c, d, e, f, g]
In this output, the subset array successfully contains elements from index 2 to index 6, demonstrating the effective use of the System.arraycopy
method to obtain a subset of an array in Java.
Get the Subset of an Array in Java Using Apache Commons Lang
Apache Commons Lang provides a convenient utility class, ArrayUtils
, which includes a method called subarray
that allows you to easily obtain a subset of an array. This method simplifies the process of extracting a portion of an array by taking care of the details.
The syntax for using ArrayUtils.subarray
is straightforward:
public static <T> T[] subarray(T[] array, int startIndexInclusive, int endIndexExclusive)
Parameters:
array
: The source array.startIndexInclusive
: The starting index (inclusive).endIndexExclusive
: The ending index (exclusive).
Let’s consider an example where we have an array of integers, and we want to extract a subset from index 1 to index 4 using ArrayUtils.subarray
.
import java.util.Arrays;
import org.apache.commons.lang3.ArrayUtils;
public class ArraySubsetApacheCommonsExample {
public static void main(String[] args) {
Integer[] originalArray = {10, 20, 30, 40, 50, 60, 70};
int startIndex = 1;
int endIndex = 5;
Integer[] subsetArray = ArrayUtils.subarray(originalArray, startIndex, endIndex);
System.out.println("Original Array: " + Arrays.toString(originalArray));
System.out.println("Subset Array: " + Arrays.toString(subsetArray));
}
}
In this example, we import ArrayUtils
from the org.apache.commons.lang3
package.
We declare an array named originalArray
containing integers. The startIndex
is set to 1, and the endIndex
is set to 5, indicating that we want to include elements from index 1 up to, but not including, index 5 in our subset.
The key line of code is:
Integer[] subsetArray = ArrayUtils.subarray(originalArray, startIndex, endIndex);
Here, we simply call the subarray
method with our originalArray
, startIndex
, and endIndex
. The method takes care of creating a new array containing the specified subset.
Code Output:
Original Array: [10, 20, 30, 40, 50, 60, 70]
Subset Array: [20, 30, 40, 50]
In this output, the subset array successfully contains elements from index 1 to index 4, demonstrating the effective use of ArrayUtils.subarray
from Apache Commons Lang to obtain a subset of an array in Java.
Get the Subset of an Array in Java Using List Conversion
Converting an array to a list in Java and utilizing the subList()
method provides an alternative approach for obtaining a subset of an array. This method involves converting the array into a list, extracting the desired subset using the subList()
method, and then converting it back into an array.
Although this approach involves additional steps, it can be a practical solution when working with more complex operations on the subset.
The syntax for using list conversion involves the Arrays.asList()
method and the subList()
method:
List<T> list = Arrays.asList(array);
List<T> subsetList = list.subList(startIndex, endIndex);
T[] subsetArray = subsetList.toArray(new T[0]);
Parameters:
array
: The source array.startIndex
: The starting index (inclusive).endIndex
: The ending index (exclusive).
Let’s consider an example where we have an array of strings, and we want to extract a subset from index 2 to index 6 using list conversion.
import java.util.Arrays;
import java.util.List;
public class ArraySubsetListConversionExample {
public static void main(String[] args) {
String[] originalArray = {"apple", "banana", "orange", "grape", "mango", "kiwi", "pear"};
int startIndex = 2;
int endIndex = 7;
String[] subsetArray =
Arrays.asList(originalArray).subList(startIndex, endIndex).toArray(new String[0]);
System.out.println("Original Array: " + Arrays.toString(originalArray));
System.out.println("Subset Array: " + Arrays.toString(subsetArray));
}
}
In this example, we use Arrays.asList(originalArray)
to convert the original array of strings into a list. We then apply the subList(startIndex, endIndex)
method to the list to extract the desired subset.
Finally, we use toArray(new String[0])
to convert the subset list back into an array of strings.
The key line of code is:
String[] subsetArray =
Arrays.asList(originalArray).subList(startIndex, endIndex).toArray(new String[0]);
Here, Arrays.asList(originalArray)
creates a list from the original array, and subList(startIndex, endIndex)
extracts the subset. The toArray(new String[0])
part converts the subset list into a new array of strings.
Code Output:
Original Array: [apple, banana, orange, grape, mango, kiwi, pear]
Subset Array: [orange, grape, mango, kiwi, pear]
In this output, the subset array successfully contains elements from index 2 to index 6, showcasing the effective use of List Conversion to obtain a subset of an array in Java.
Get the Subset of an Array in Java Using a Custom Method
Creating a custom method to extract a subset of an array allows for a more tailored approach to array manipulation. By utilizing a custom method, you have the flexibility to define specific conditions or criteria for extracting elements from the original array.
This method is particularly useful when you need to implement a custom logic for subset extraction that is not covered by standard utility methods.
The syntax for a custom method involves defining a method that takes the original array, starting index, and ending index as parameters and returns the desired subset:
public static <T> T[] getSubset(T[] array, int startIndex, int endIndex) {
// Custom logic to extract the subset
}
Where:
array
: The source array.startIndex
: The starting index (inclusive).endIndex
: The ending index (exclusive).
Let’s consider an example where we have an array of integers, and we want to extract a subset containing only even numbers.
import java.util.ArrayList;
import java.util.Arrays;
import java.util.List;
public class ArraySubsetCustomMethodExample {
public static void main(String[] args) {
Integer[] originalArray = {1, 2, 3, 4, 5, 6, 7, 8, 9, 10};
Integer[] subsetArray = getEvenSubset(originalArray, 1, 8);
System.out.println("Original Array: " + Arrays.toString(originalArray));
System.out.println("Even Subset Array: " + Arrays.toString(subsetArray));
}
public static <T> T[] getEvenSubset(T[] array, int startIndex, int endIndex) {
List<T> subsetList = new ArrayList<>();
for (int i = startIndex; i < endIndex; i++) {
if ((Integer) array[i] % 2 == 0) {
subsetList.add(array[i]);
}
}
return subsetList.toArray(Arrays.copyOf(array, 0));
}
}
In this example, we define a custom method, getEvenSubset
, that takes the original array, starting index, and ending index as parameters.
Inside the method, we iterate through the specified range and add only even numbers to a List
named subsetList
. Finally, we convert the subsetList
back to an array using toArray()
.
The crucial lines of code are:
Integer[] subsetArray = getEvenSubset(originalArray, 1, 8);
Here, getEvenSubset
is called with the original array and the range (from index 1 to index 8).
Code Output:
Original Array: [1, 2, 3, 4, 5, 6, 7, 8, 9, 10]
Even Subset Array: [2, 4, 6, 8]
In this output, the custom method successfully extracts a subset containing only even numbers from index 1 to index 8, demonstrating the flexibility of using a custom method to obtain a subset of an array in Java.
Conclusion
Obtaining a subset of an array in Java can be approached through various methods, each offering its own advantages and use cases. From using built-in methods like Arrays.copyOf()
and Arrays.copyOfRange()
to leveraging Java 8 streams with IntStream
, and employing utility classes such as Apache Commons Lang’s ArrayUtils
, each method provides a unique solution based on the specific requirements.
Additionally, we discussed list conversion as an alternative, which involves converting the array to a list, using the subList()
method, and then converting it back to an array. This approach is particularly useful when more complex operations on the subset are needed.
Finally, we demonstrated the flexibility of creating a custom method, allowing developers to implement specific logic for subset extraction based on their unique needs.
Choosing the most suitable method depends on the specific use case, readability, and performance requirements. By understanding these array manipulation techniques, you can enhance your ability to work with arrays efficiently and tailor your approach to the demands of your projects.
Sheeraz is a Doctorate fellow in Computer Science at Northwestern Polytechnical University, Xian, China. He has 7 years of Software Development experience in AI, Web, Database, and Desktop technologies. He writes tutorials in Java, PHP, Python, GoLang, R, etc., to help beginners learn the field of Computer Science.
LinkedIn Facebook