Array Range in Java
- Use Another Array to Get a Range of Elements From an Array in Java
-
Use the
copyOfRange()
Method to Get a Range of Elements From an Array in Java -
Use the
stream
Object to Get a Range of Elements From an Array in Java
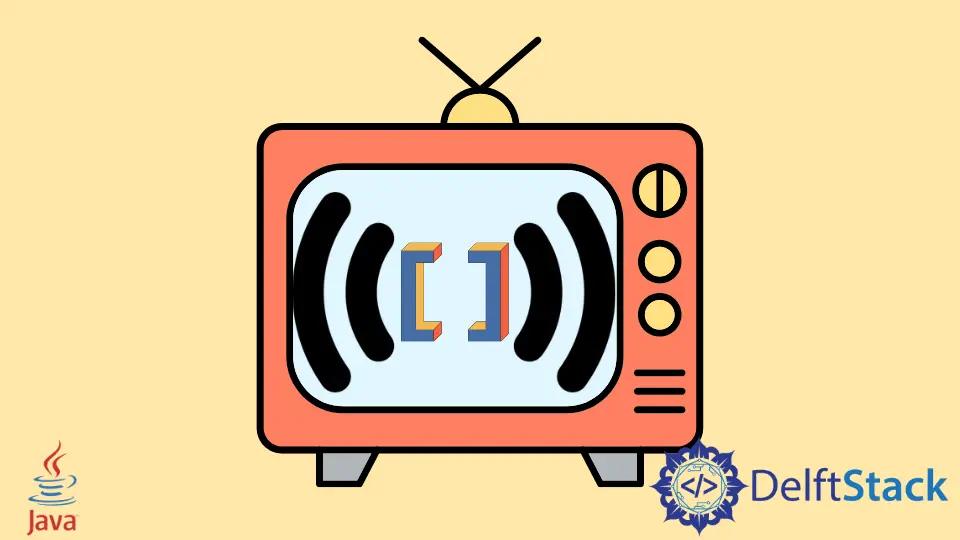
Array slicing is done to get a range of elements from the existing array. It is like extracting a sub-array from a given array.
This tutorial demonstrates how to get a range of elements from an existing array.
Use Another Array to Get a Range of Elements From an Array in Java
We will copy the required elements to a new array in this method. First, we have to find the start and end index of the array based on the given range. Then, we have to create an empty array of size (endIndex
- startIndex
). Finally, we copy the elements from the given array to the sliced array and print it.
See the code given below.
import java.util.Arrays;
public class Main {
public static int[] getSlice(int[] array, int startIndex, int endIndex) {
int[] slicedArray = new int[endIndex - startIndex];
for (int i = 0; i < slicedArray.length; i++) {
slicedArray[i] = array[startIndex + i];
}
return slicedArray;
}
public static void main(String args[]) {
int[] array = {87, 56, 29, 11, 45, 33, 84, 4, 67, 73};
int startIndex = 4, endIndex = 9;
int[] slicedArray = getSlice(array, startIndex, endIndex + 1);
System.out.println("Slice of Array: " + Arrays.toString(slicedArray));
}
}
Output:
Slice of Array: [45, 33, 84, 4, 67, 73]
It is advisable to use this method when only dealing with an array of small sizes. Iterating over an array and copying to another array requires a lot of memory and time.
Use the copyOfRange()
Method to Get a Range of Elements From an Array in Java
This method is defined in java.util.Arrays
Class. This method copies the specific range of elements from the given original array to a new array and then returns this array.
For example,
import java.util.Arrays;
public class Main {
public static int[] slice(int[] array, int startIndex, int endIndex) {
int[] slicedArray = Arrays.copyOfRange(array, startIndex, endIndex);
return slicedArray;
}
public static void main(String args[]) {
int[] array = {87, 56, 29, 11, 45, 33, 84, 4, 67, 73};
int startIndex = 4, endIndex = 9;
int[] sliceArray = slice(array, startIndex, endIndex + 1);
System.out.println("Slice of Array: " + Arrays.toString(sliceArray));
}
}
Output:
Slice of Array: [45, 33, 84, 4, 67, 73]
Use the stream
Object to Get a Range of Elements From an Array in Java
We start with finding the startIndex
and endIndex
of the range in this method. Then, we have to convert the element in the required range into Primitive Stream using the range()
method. Next, the map()
method is used to map the required elements for the range from the original array. Finally, the toArray()
method converts the mapped array into an array, and we print it.
See the code given below.
import java.util.Arrays;
import java.util.stream.IntStream;
public class Main {
public static int[] findSlice(int[] array, int startIndex, int endIndex) {
int[] slcarray = IntStream.range(startIndex, endIndex).map(i -> array[i]).toArray();
return slcarray;
}
public static void main(String args[]) {
int[] array = {87, 56, 29, 11, 45, 33, 84, 4, 67, 73};
int startIndex = 4, endIndex = 9;
int[] slcarray = findSlice(array, startIndex, endIndex + 1);
System.out.println("Slice of array for the specified range is: " + Arrays.toString(slcarray));
}
}
Output:
Slice of Array: [45, 33, 84, 4, 67, 73]