How to Repeat a String in Java
-
Repeat a String Using
regex
in Java -
Repeat a String Using
.repeat()
in Java -
Repeat a String Using Apache Commons
StringUtils
Class
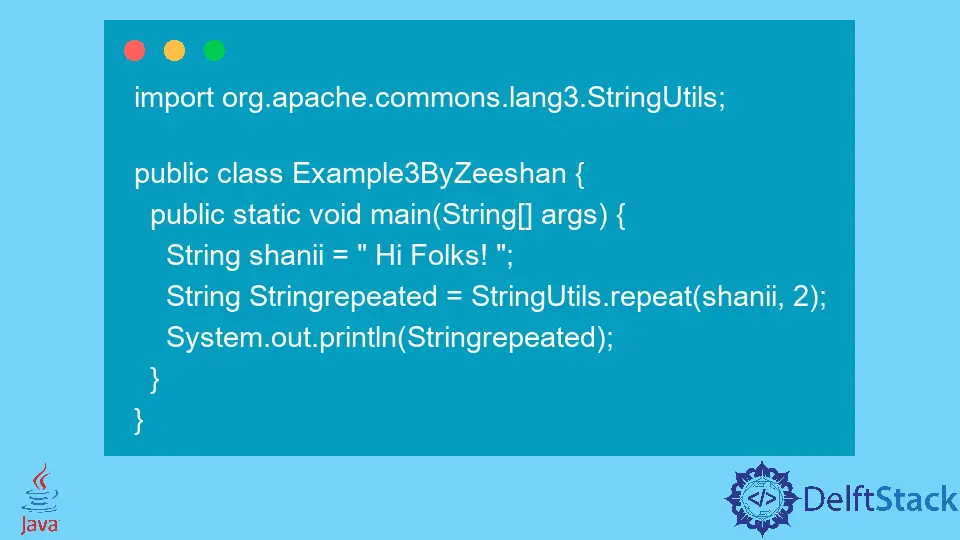
This article will go through several simple approaches to repeating a string in Java.
Repeat a String Using regex
in Java
The use of regex
can be an option for repeating a string. Let’s break it down with a basic example, as shown below.
In the below-discussed example, we’ll repeat the below string 5 times:
String shanii = " Hey! Muhammad Zeeshan here ";
The variable name Stringrepeated
will be used to store the repeated string, and we will proceed to print that variable.
String Stringrepeated = new String(new char[5]).replace("\0", shanii);
System.out.println(Stringrepeated);
Example Code:
public class Example1ByZeeshan {
public static void main(String[] args) {
String shanii = " Hey! Muhammad Zeeshan here ";
String Stringrepeated = new String(new char[5]).replace("\0", shanii);
System.out.println(Stringrepeated);
}
}
Output:
Hey! Muhammad Zeeshan here Hey! Muhammad Zeeshan here Hey! Muhammad Zeeshan here Hey! Muhammad Zeeshan here Hey! Muhammad Zeeshan here
Repeat a String Using .repeat()
in Java
The value of the string returned by this method is the concatenation of the provided string repeated the specified number of times. Let’s understand it with an example below.
In the following example, we will only use the .repeat()
function to repeat the specified string 5 times. A blank string will be returned when the string does not include any characters or the count is 0
.
Example Code:
public class Example2ByZeeshan {
public static void main(String[] args) {
String shanii = " Let's Code ";
System.out.println(shanii.repeat(5));
}
}
Output:
Let's Code Let's Code Let's Code Let's Code Let's Code
There are other possibilities if your project uses Java libraries: the StringUtils
function in Apache Commons.
Repeat a String Using Apache Commons StringUtils
Class
We can utilize the Apache Common’s StringUtils
class and its .repeat()
function. The following is an example of implementing the StringUtils
class into your code.
In the following code snippet, we’ll use the StringUtils
function with two parameters: the string and the desired repetition count.
Example Code:
import org.apache.commons.lang3.StringUtils;
public class Example3ByZeeshan {
public static void main(String[] args) {
String shanii = " Hi Folks! ";
String Stringrepeated = StringUtils.repeat(shanii, 2);
System.out.println(Stringrepeated);
}
}
Output:
Hi Folks! Hi Folks!
I have been working as a Flutter app developer for a year now. Firebase and SQLite have been crucial in the development of my android apps. I have experience with C#, Windows Form Based C#, C, Java, PHP on WampServer, and HTML/CSS on MYSQL, and I have authored articles on their theory and issue solving. I'm a senior in an undergraduate program for a bachelor's degree in Information Technology.
LinkedIn