Java で文字列を繰り返す
-
Java で
regex
を使用して文字列を繰り返す -
Java で
.repeat()
を使用して文字列を繰り返す -
Apache Commons
StringUtils
クラスを使用して文字列を繰り返す
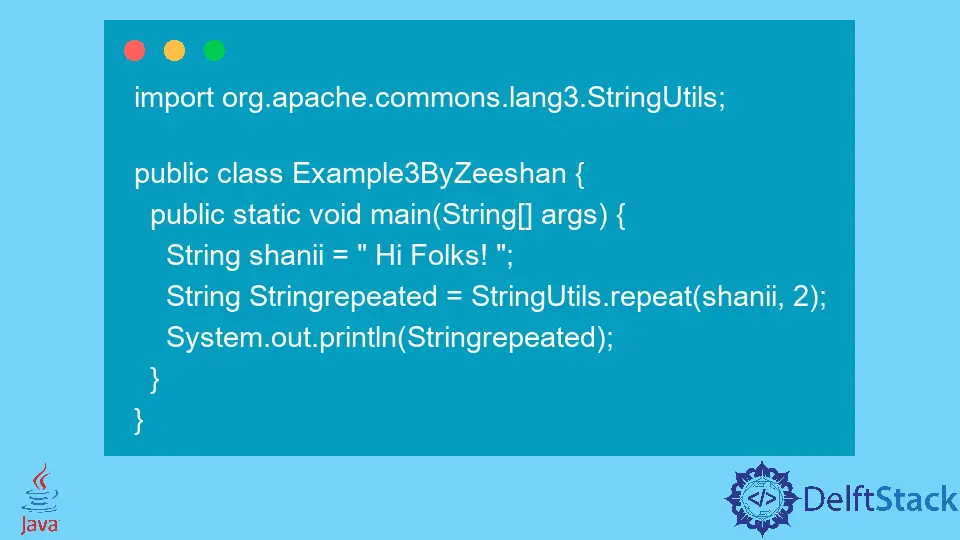
この記事では、Java で文字列を繰り返す簡単な方法をいくつか紹介します。
Java で regex
を使用して文字列を繰り返す
regex
の使用は、文字列を繰り返すためのオプションです。 以下に示すように、基本的な例でそれを分解してみましょう。
以下で説明する例では、以下の文字列を 5 回繰り返します。
String shanii = " Hey! Muhammad Zeeshan here ";
変数名 Stringrepeated
は繰り返される文字列を格納するために使用され、その変数の出力に進みます。
String Stringrepeated = new String(new char[5]).replace("\0", shanii);
System.out.println(Stringrepeated);
コード例:
public class Example1ByZeeshan {
public static void main(String[] args) {
String shanii = " Hey! Muhammad Zeeshan here ";
String Stringrepeated = new String(new char[5]).replace("\0", shanii);
System.out.println(Stringrepeated);
}
}
出力:
Hey! Muhammad Zeeshan here Hey! Muhammad Zeeshan here Hey! Muhammad Zeeshan here Hey! Muhammad Zeeshan here Hey! Muhammad Zeeshan here
Java で .repeat()
を使用して文字列を繰り返す
このメソッドによって返される文字列の値は、提供された文字列を指定された回数だけ連結したものです。 以下の例でそれを理解しましょう。
次の例では、.repeat()
関数のみを使用して、指定された文字列を 5 回繰り返します。 文字列に文字が含まれていない場合、またはカウントが 0
の場合は、空白の文字列が返されます。
コード例:
public class Example2ByZeeshan {
public static void main(String[] args) {
String shanii = " Let's Code ";
System.out.println(shanii.repeat(5));
}
}
出力:
Let's Code Let's Code Let's Code Let's Code Let's Code
プロジェクトが Java ライブラリを使用している場合、別の可能性があります: Apache Commons の StringUtils
関数です。
Apache Commons StringUtils
クラスを使用して文字列を繰り返す
Apache Common の StringUtils
クラスとその .repeat()
関数を利用できます。 以下は、StringUtils
クラスをコードに実装する例です。
次のコード スニペットでは、StringUtils
関数を 2つのパラメータ (文字列と目的の繰り返し回数) と共に使用します。
コード例:
import org.apache.commons.lang3.StringUtils;
public class Example3ByZeeshan {
public static void main(String[] args) {
String shanii = " Hi Folks! ";
String Stringrepeated = StringUtils.repeat(shanii, 2);
System.out.println(Stringrepeated);
}
}
出力:
Hi Folks! Hi Folks!
I have been working as a Flutter app developer for a year now. Firebase and SQLite have been crucial in the development of my android apps. I have experience with C#, Windows Form Based C#, C, Java, PHP on WampServer, and HTML/CSS on MYSQL, and I have authored articles on their theory and issue solving. I'm a senior in an undergraduate program for a bachelor's degree in Information Technology.
LinkedIn