How to Do String Interpolation in Java
-
Java String Interpolation Using the
+
Operator (Concatenation) -
Java String Interpolation Using the
format()
Method -
Java String Interpolation Using the
MessageFormat
Class in Java -
Java String Interpolation Using the
StringBuilder
Class in Java -
Java String Interpolation Using the
formatted()
Method in Java 15
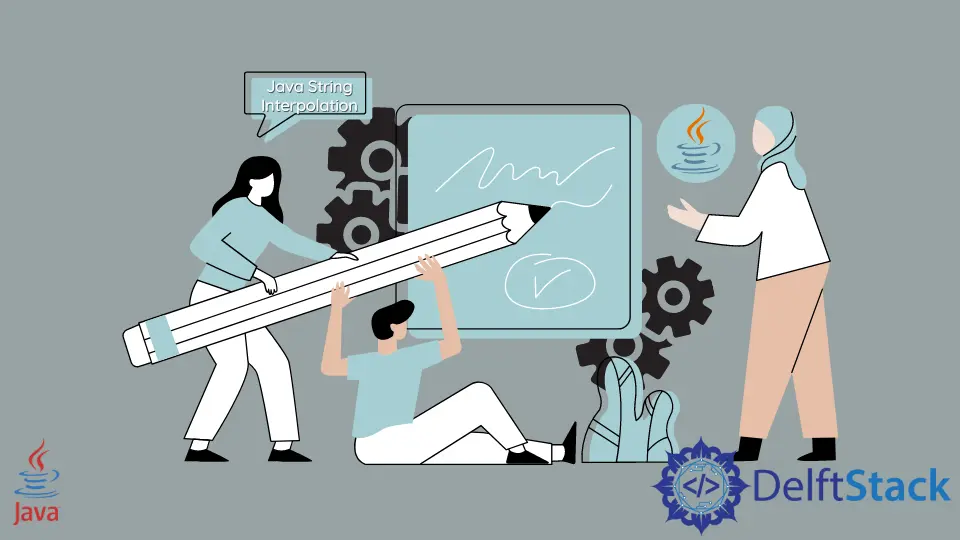
This tutorial introduces how to do string interpolation in Java and lists some example codes to understand the topic.
String interpolation is a process of replacing the placeholders with the values in a string literal. To do the string interpolation in Java, we can use several methods such as the format()
method of String
class, the format()
method of MessageFormat
class, the StringBuilder
class, and the formatted()
method of the String
class, etc.
Java String Interpolation Using the +
Operator (Concatenation)
It is the simplest approach. We can use +
to perform the string interpolation. Java uses the +
operator to concatenate variables with the string. So we can use it for string interpolation as well. Below we put two variables in the string and get a string as a result.
public class SimpleTesting {
public static void main(String[] args) {
String site_name = "DelfStack";
String type = "'How to Guide'";
String message = site_name + " is a " + type + " Portal";
System.out.println(message);
}
}
Output:
DelfStack is a 'How to Guide' Portal
Java String Interpolation Using the format()
Method
We can use the format()
method of the String
class to format a string by interpolating variables. The format()
method takes two arguments, the first is string format, and the second is the list of arguments.
public class SimpleTesting {
public static void main(String[] args) {
String site_name = "DelfStack";
String type = "'How to Guide'";
String message = String.format("%s is a %s portal", site_name, type);
System.out.println(message);
}
}
Output:
DelfStack is a 'How to Guide' portal
Java String Interpolation Using the MessageFormat
Class in Java
The MessageFormat
class provides a method format()
that can be used to perform string interpolation. Here, we replace the variables into the string with the placeholders ({0}, {1}, etc)
. See the example below.
import java.text.MessageFormat;
public class SimpleTesting {
public static void main(String[] args) {
String site_name = "DelfStack";
String type = "'How to Guide'";
String message = MessageFormat.format("{0} is a {1} Portal", site_name, type);
System.out.println(message);
}
}
Output:
DelfStack is a 'How to Guide' Portal
Java String Interpolation Using the StringBuilder
Class in Java
We can use the append()
method of the StringBuilder
class to append the variables into the string. The StringBuilder
is a mutable version of String
and can be modified with variables. Here, we created a string by interpolating variables.
public class SimpleTesting {
public static void main(String[] args) {
String site_name = "DelfStack";
String type = "'How to Guide'";
StringBuilder message = new StringBuilder(site_name)
.append(" is a ")
.append(String.valueOf(type))
.append(" Portal");
System.out.println(message);
}
}
Output:
DelfStack is a 'How to Guide' Portal
Java String Interpolation Using the formatted()
Method in Java 15
If you are working with Java 15 or higher version, you can use the formatted()
method. Java added this method to Java 15 version to enable string formatting. This method takes a single argument of Object[]
type. See the example below.
public class SimpleTesting {
public static void main(String[] args) {
String site_name[] = {"DelfStack", "How to Guide"};
String message = "%s is a '%s' Portal".formatted(site_name);
System.out.println(message);
}
}
Output:
DelfStack is a 'How to Guide' Portal