在 Java 中格式化字串
Mohammad Irfan
2023年10月12日
-
使用
+
運算子的 Java 字串插值(連詞) -
使用
format()
方法進行 Java 字串插值 -
使用 Java 中的
MessageFormat
類進行 Java 字串插值 -
使用 Java 中的
StringBuilder
類進行 Java 字串插值 -
使用 Java 15 中的
formatted()
方法進行 Java 字串插值
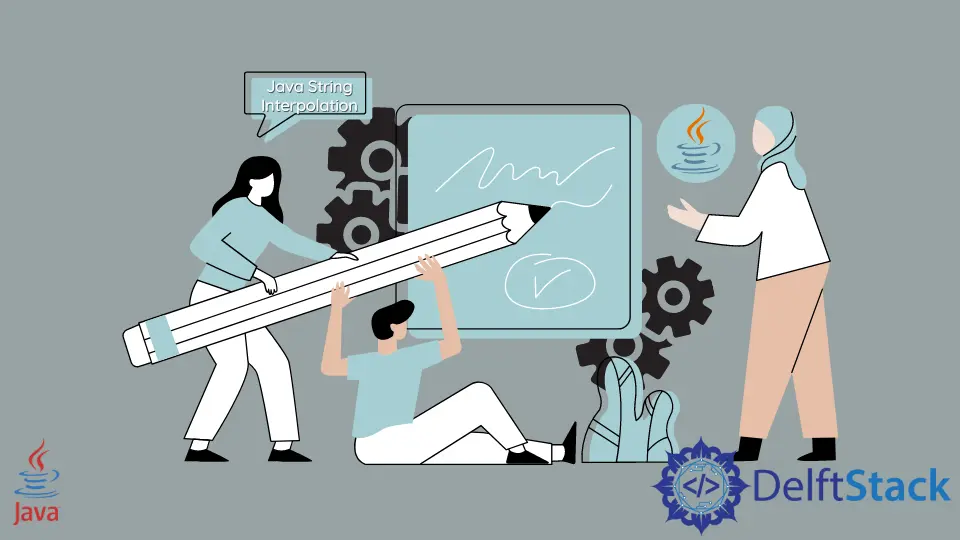
本教程介紹瞭如何在 Java 中進行格式化字串,並列舉了一些示例程式碼來理解這個主題。
字串插值是將佔位符替換為字串文字中的值的過程。在 Java 中進行字串插值,我們可以使用幾種方法,如 String
類的 format()
方法、MessageFormat
類的 format()
方法、StringBuilder
類、String
類的 formatted()
方法等。
使用+
運算子的 Java 字串插值(連詞)
這是最簡單的方法。我們可以使用+
來進行字串插值。Java 使用+
操作符來連線變數與字串。所以我們也可以用它來進行字串插值。下面我們在字串中放入兩個變數,並得到一個字串。
public class SimpleTesting {
public static void main(String[] args) {
String site_name = "DelfStack";
String type = "'How to Guide'";
String message = site_name + " is a " + type + " Portal";
System.out.println(message);
}
}
輸出:
DelfStack is a 'How to Guide' Portal
使用 format()
方法進行 Java 字串插值
我們可以使用 String
類的 format()
方法,通過插值變數來格式化一個字串。format()
方法需要兩個引數,第一個是字串格式,第二個是引數。
public class SimpleTesting {
public static void main(String[] args) {
String site_name = "DelfStack";
String type = "'How to Guide'";
String message = String.format("%s is a %s portal", site_name, type);
System.out.println(message);
}
}
輸出:
DelfStack is a 'How to Guide' portal
使用 Java 中的 MessageFormat
類進行 Java 字串插值
MessageFormat
類提供了一個 format()
方法,可以用來執行字串插值。在這裡,我們用佔位符 ({0}, {1} ...)
替換字串中的變數。請看下面的例子。
import java.text.MessageFormat;
public class SimpleTesting {
public static void main(String[] args) {
String site_name = "DelfStack";
String type = "'How to Guide'";
String message = MessageFormat.format("{0} is a {1} Portal", site_name, type);
System.out.println(message);
}
}
輸出:
DelfStack is a 'How to Guide' Portal
使用 Java 中的 StringBuilder
類進行 Java 字串插值
我們可以使用 StringBuilder
類的 append()
方法將變數追加到字串中。StringBuilder
是 String
的可變版本,可以用變數進行修改。在這裡,我們通過插值變數來建立一個字串。
public class SimpleTesting {
public static void main(String[] args) {
String site_name = "DelfStack";
String type = "'How to Guide'";
StringBuilder message = new StringBuilder(site_name)
.append(" is a ")
.append(String.valueOf(type))
.append(" Portal");
System.out.println(message);
}
}
輸出:
DelfStack is a 'How to Guide' Portal
使用 Java 15 中的 formatted()
方法進行 Java 字串插值
如果你正在使用 Java 15 或更高版本,你可以使用 formatted()
方法。Java 在 Java 15 版本中加入了這個方法,以實現字串格式化。這個方法需要一個 Object[]
型別的單一引數。請看下面的例子。
public class SimpleTesting {
public static void main(String[] args) {
String site_name[] = {"DelfStack", "How to Guide"};
String message = "%s is a '%s' Portal".formatted(site_name);
System.out.println(message);
}
}
輸出:
DelfStack is a 'How to Guide' Portal