在 Java 中格式化字符串
Mohammad Irfan
2023年10月12日
-
使用
+
运算符的 Java 字符串插值(连词) -
使用
format()
方法进行 Java 字符串插值 -
使用 Java 中的
MessageFormat
类进行 Java 字符串插值 -
使用 Java 中的
StringBuilder
类进行 Java 字符串插值 -
使用 Java 15 中的
formatted()
方法进行 Java 字符串插值
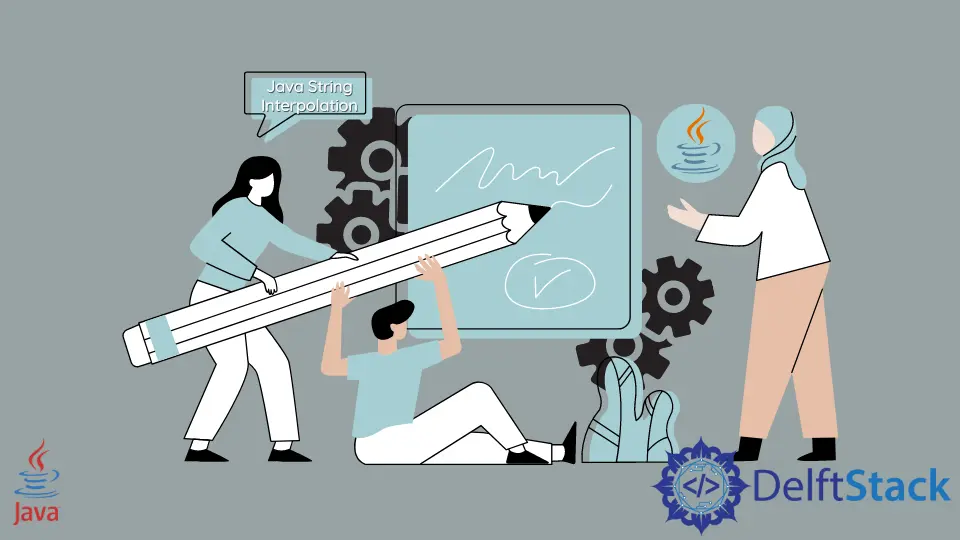
本教程介绍了如何在 Java 中进行格式化字符串,并列举了一些示例代码来理解这个主题。
字符串插值是将占位符替换为字符串文字中的值的过程。在 Java 中进行字符串插值,我们可以使用几种方法,如 String
类的 format()
方法、MessageFormat
类的 format()
方法、StringBuilder
类、String
类的 formatted()
方法等。
使用+
运算符的 Java 字符串插值(连词)
这是最简单的方法。我们可以使用+
来进行字符串插值。Java 使用+
操作符来连接变量与字符串。所以我们也可以用它来进行字符串插值。下面我们在字符串中放入两个变量,并得到一个字符串。
public class SimpleTesting {
public static void main(String[] args) {
String site_name = "DelfStack";
String type = "'How to Guide'";
String message = site_name + " is a " + type + " Portal";
System.out.println(message);
}
}
输出:
DelfStack is a 'How to Guide' Portal
使用 format()
方法进行 Java 字符串插值
我们可以使用 String
类的 format()
方法,通过插值变量来格式化一个字符串。format()
方法需要两个参数,第一个是字符串格式,第二个是参数。
public class SimpleTesting {
public static void main(String[] args) {
String site_name = "DelfStack";
String type = "'How to Guide'";
String message = String.format("%s is a %s portal", site_name, type);
System.out.println(message);
}
}
输出:
DelfStack is a 'How to Guide' portal
使用 Java 中的 MessageFormat
类进行 Java 字符串插值
MessageFormat
类提供了一个 format()
方法,可以用来执行字符串插值。在这里,我们用占位符 ({0}, {1} ...)
替换字符串中的变量。请看下面的例子。
import java.text.MessageFormat;
public class SimpleTesting {
public static void main(String[] args) {
String site_name = "DelfStack";
String type = "'How to Guide'";
String message = MessageFormat.format("{0} is a {1} Portal", site_name, type);
System.out.println(message);
}
}
输出:
DelfStack is a 'How to Guide' Portal
使用 Java 中的 StringBuilder
类进行 Java 字符串插值
我们可以使用 StringBuilder
类的 append()
方法将变量追加到字符串中。StringBuilder
是 String
的可变版本,可以用变量进行修改。在这里,我们通过插值变量来创建一个字符串。
public class SimpleTesting {
public static void main(String[] args) {
String site_name = "DelfStack";
String type = "'How to Guide'";
StringBuilder message = new StringBuilder(site_name)
.append(" is a ")
.append(String.valueOf(type))
.append(" Portal");
System.out.println(message);
}
}
输出:
DelfStack is a 'How to Guide' Portal
使用 Java 15 中的 formatted()
方法进行 Java 字符串插值
如果你正在使用 Java 15 或更高版本,你可以使用 formatted()
方法。Java 在 Java 15 版本中加入了这个方法,以实现字符串格式化。这个方法需要一个 Object[]
类型的单一参数。请看下面的例子。
public class SimpleTesting {
public static void main(String[] args) {
String site_name[] = {"DelfStack", "How to Guide"};
String message = "%s is a '%s' Portal".formatted(site_name);
System.out.println(message);
}
}
输出:
DelfStack is a 'How to Guide' Portal