How to Generate Random Number Within a Specified Range in Java
-
Generate Random Number Using the
ThreadLocalRandom
Class in Java -
Generate Random Number Using the
Math
Class in Java -
Generate Random Number Using the
Random
Class in Java -
Generate Random Number Using the
Random
Class in Java -
Generate Random Number Using the
Random
Class andIntStream
in Java -
Generate Random Number Using the
nextFloat
Method in Java -
Generate Random Number Using the
RandomData
Class in Java -
Generate Random Number Using
SecureRandom
Class in Java -
Generate Random Number Using the
RandomUtils
Class in Java
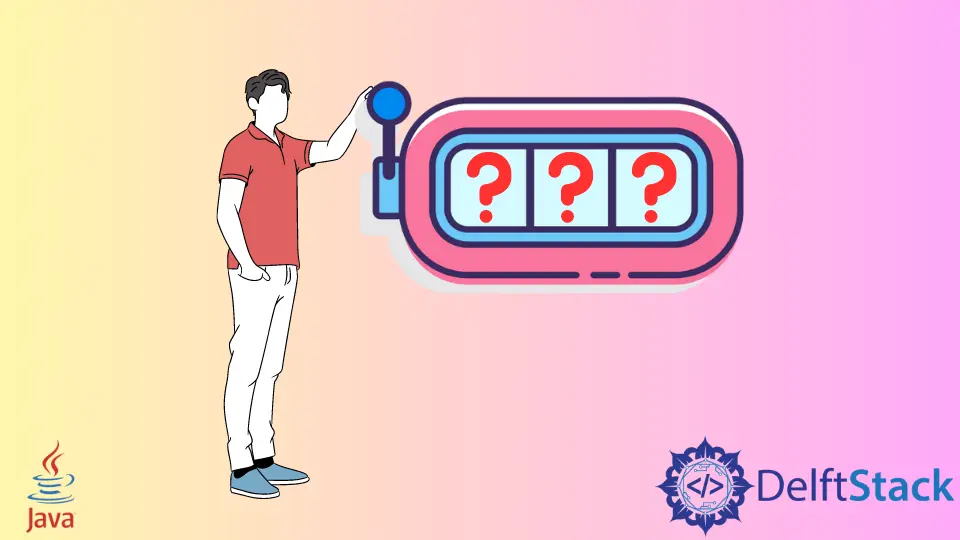
This tutorial introduces how to generate a random number within a specified range in Java.
There are several ways to generate random number in Java, such as the nextInt()
method of the ThreadLocalRandom
class, the random()
method of the Math
class, the nextInt()
method of the Random
class, the ints()
method of the Random
class, the nextFloat()
method of the Random
class and the RandomUtil
class, etc.
Generate Random Number Using the ThreadLocalRandom
Class in Java
Java concurrent package provides a class ThreadLocalRandom
that has the nextInt()
method. This method can generate a random integer within the specified range. See the below example.
import java.util.concurrent.ThreadLocalRandom;
public class SimpleTesting {
public static void main(String[] args) {
int min_val = 10;
int max_val = 100;
ThreadLocalRandom tlr = ThreadLocalRandom.current();
int randomNum = tlr.nextInt(min_val, max_val + 1);
System.out.println("Random Number: " + randomNum);
}
}
Output:
Value in double: 12.9
Value in int: 12
Generate Random Number Using the Math
Class in Java
Java Math
class can be used to generate a random number within the specified range. Here, we use the random()
method of the Math
class to get a random number. See the example below.
public class SimpleTesting {
public static void main(String[] args) {
int min_val = 10;
int max_val = 100;
double randomNum = Math.random() * (max_val - min_val);
System.out.println("Random Number: " + randomNum);
}
}
Output:
Id: 1212
Name: Samre
Generate Random Number Using the Random
Class in Java
The Random
class of Java can generate a random integer within the specified range by using the nextInt()
method, which returns an integer value. See the example below.
import java.util.Random;
public class SimpleTesting {
public static void main(String[] args) {
int min_val = 10;
int max_val = 100;
Random ran = new Random();
int x = ran.nextInt(max_val) + min_val;
System.out.println("Random Number: " + x);
}
}
Output:
Id: 1212
Name: Samre
Generate Random Number Using the Random
Class in Java
This is another solution for getting a random integer within the specified range. Here, we use the nextInt()
method of the Random
class with different parameters. See the example below.
import java.util.Random;
public class SimpleTesting {
public static void main(String[] args) {
int min_val = 10;
int max_val = 100;
Random rand = new Random();
int randomNum = min_val + rand.nextInt((max_val - min_val) + 1);
System.out.println("Random Number: " + randomNum);
}
}
Output:
Random Number: 75
Generate Random Number Using the Random
Class and IntStream
in Java
Here, we use the ints()
method of the Random
class that returns a stream of random numbers. We use the forEach()
method to print all the random integers generated by the ints()
method. See the below example.
import java.util.Random;
import java.util.stream.IntStream;
public class SimpleTesting {
public static void main(String[] args) {
int min_val = 10;
int max_val = 100;
Random rand = new Random();
IntStream stream = rand.ints(5, min_val, max_val);
stream.forEach(System.out::println);
}
}
Output:
94
35
90
74
47
Generate Random Number Using the nextFloat
Method in Java
The Random
class provides a method nextFloat()
to generate a float type random number. If we want to get a float-type random number, then this method is useful. See the below example.
import java.util.Random;
public class SimpleTesting {
public static void main(String[] args) {
int min_val = 10;
int max_val = 100;
Random rand = new Random();
float rand_val = rand.nextFloat() * (max_val - min_val);
System.out.println(rand_val);
}
}
Output:
71.88764
Generate Random Number Using the RandomData
Class in Java
If you are working with the apache commons library, then use the RandomData
class. This class provides a method nextInt()
that returns an integer value. We can use this method to generate random integers within the specified range. See the example below.
import org.apache.commons.math.random.RandomData;
import org.apache.commons.math.random.RandomDataImpl;
public class SimpleTesting {
public static void main(String[] args) {
int min_val = 10;
int max_val = 100;
RandomData randomData randomData = new RandomDataImpl();
int number = randomData.nextInt(min_val, max_val);
System.out.println(number);
}
}
Output:
72
Generate Random Number Using SecureRandom
Class in Java
The SecureRandom class belongs to the security
package of Java that is used to create a secure random number. Here, we use the Date
class to generate a seed and then the nextInt()
method to generate a random integer. See the below example.
import java.security.SecureRandom;
import java.util.Date;
public class SimpleTesting {
public static void main(String[] args) {
int min_val = 10;
int max_val = 100;
SecureRandom rand = new SecureRandom();
rand.setSeed(new Date().getTime());
int randomNum = rand.nextInt((max_val - min_val) + 1) + min_val;
System.out.println(randomNum);
}
}
Output:
65
Generate Random Number Using the RandomUtils
Class in Java
We can use the RandomUtil
class of the apache commons library to generate random integers. The nextInt()
method of this class returns an integer class. See the below example.
import org.apache.commons.lang3.RandomUtils;
public class SimpleTesting {
public static void main(String[] args) {
int min_val = 10;
int max_val = 100;
RandomUtils random = new RandomUtils();
int randomNum = random.nextInt(min_val, max_val);
System.out.println(randomNum);
}
}
Output:
66
Related Article - Java Math
- How to Calculate Probability in Java
- How to Find Factors of a Given Number in Java
- How to Evaluate a Mathematical Expression in Java
- How to Calculate the Euclidean Distance in Java
- How to Calculate Distance Between Two Points in Java
- How to Simplify or Reduce Fractions in Java