How to Convert Number to Words in Java
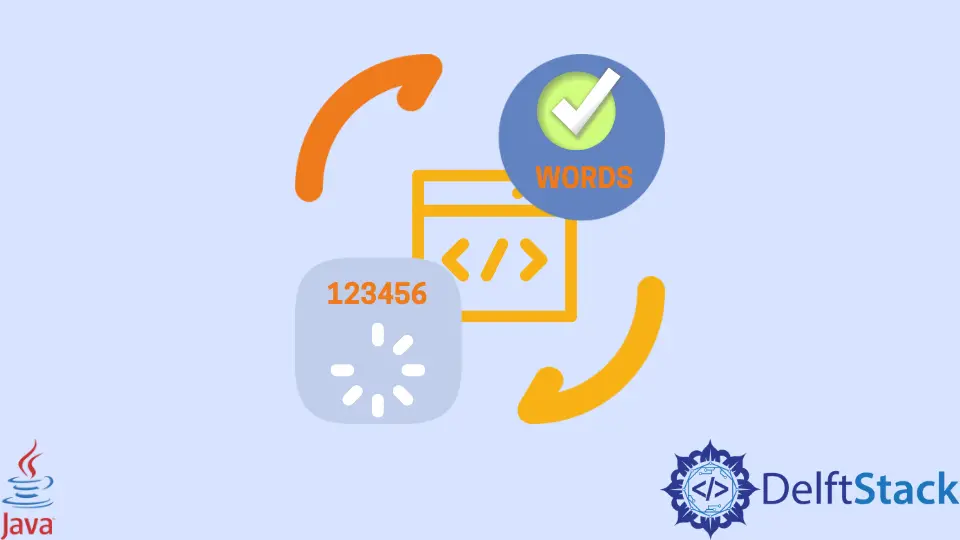
This tutorial demonstrates how to convert numbers to words in Java.
Sometimes we need to convert numbers to words; for example, 123
should convert to one hundred twenty-three
. This can be achieved in Java with small or large numbers.
Convert Four-Digit Numbers to Words in Java
First, let’s try converting a four-digit number to words in Java. For example, if the number is 4444
, it should be converted to four thousand four hundred forty-four
.
See the example:
package delftstack;
public class Example {
// function to convert numbers to words
static void ConvertFourDigittoWords(char[] InputNumber) {
// Get the length of a number
int NumberLength = InputNumber.length;
// Basic cases
if (NumberLength == 0) {
System.out.println("The input is an empty string.");
return;
}
if (NumberLength > 4) {
System.out.println("The Length of the input number is more than 4 digits.");
return;
}
// create an array for numbers in words; the first string will not be used.
String[] Single_Numbers = new String[] {
"Zero", "One", "Two", "Three", "Four", "Five", "Six", "Seven", "Eight", "Nine"};
// create an array for numbers in word; the first string will not be used.
String[] Two_Numbers = new String[] {"", "Ten", "Eleven", "Twelve", "Thirteen", "Fourteen",
"Fifteen", "Sixteen", "Seventeen", "Eighteen", "Nineteen"};
// create arrays for numbers in words; the first two strings will not be used.
String[] Tens_Numbers = new String[] {
"", "", "Twenty", "Thirty", "Forty", "Fifty", "Sixty", "Seventy", "Eighty", "Ninety"};
String[] Hundred_Thousand = new String[] {"Hundred", "Thousand"};
// this is used for debugging purposes only
System.out.print(String.valueOf(InputNumber) + " = ");
// for a single digit number
if (NumberLength == 1) {
System.out.println(Single_Numbers[InputNumber[0] - '0']);
return;
}
// the while loop will be used if the number length is more than 0
int i = 0;
while (i < InputNumber.length) {
// for the first two digits
if (NumberLength >= 3) {
if (InputNumber[i] - '0' != 0) {
System.out.print(Single_Numbers[InputNumber[i] - '0'] + " ");
System.out.print(Hundred_Thousand[NumberLength - 3] + " ");
}
--NumberLength;
}
// for the last two digits
else {
// 10-19 will be explicitly handled.
if (InputNumber[i] - '0' == 1) {
int NumebrSum = InputNumber[i] - '0' + InputNumber[i + 1] - '0';
System.out.println(Two_Numbers[NumebrSum]);
return;
}
// the 20 will be explicitly handled
else if (InputNumber[i] - '0' == 2 && InputNumber[i + 1] - '0' == 0) {
System.out.println("twenty");
return;
}
// for the rest of two digit numbers from 20 to 99
else {
int a = (InputNumber[i] - '0');
if (a > 0)
System.out.print(Tens_Numbers[a] + " ");
else
System.out.print("");
++i;
if (InputNumber[i] - '0' != 0)
System.out.println(Single_Numbers[InputNumber[i] - '0']);
}
}
++i;
}
}
// main method
public static void main(String[] args) {
ConvertFourDigittoWords("6542".toCharArray());
ConvertFourDigittoWords("876".toCharArray());
ConvertFourDigittoWords("34".toCharArray());
ConvertFourDigittoWords("9".toCharArray());
ConvertFourDigittoWords("76544".toCharArray());
ConvertFourDigittoWords("".toCharArray());
}
}
The code above will convert any number with up to four digits into words. See output:
6542 = Six Thousand Five Hundred Forty Two
876 = Eight Hundred Seventy Six
34 = Thirty Four
9 = Nine
The Length of the input number is more than 4 digits.
The input is an empty string.
But what if the number is more than four digits? We can also create a program that accepts numbers with up to 15 digits.
Convert Fifteen-Digit Numbers to Words in Java
In the above example, we used Hundred
and Thousand
for four-digit numbers. In this example, we need to use Trillion
, Billion
, and Million
; let’s try to implement an example that converts a number of up to 15 digits into words.
package delftstack;
public class Example {
static String LongNumberstoWords(long Long_Number) {
long Number_Limit = 1000000000000L;
long Current_Limit = 0;
long M = 0;
// If the number is zero, return zero
if (Long_Number == 0)
return ("Zero");
// Array for the powers of 10
String Multiplier_Array[] = {"", "Trillion", "Billion", "Million", "Thousand"};
// Array for 20 numbers
String FirstTwenty_Numbers[] = {"", "One", "Two", "Three", "Four", "Five", "Six", "Seven",
"Eight", "Nine", "Ten", "Eleven", "Twelve", "Thirteen", "Fourteen", "Fifteen", "Sixteen",
"Seventeen", "Eighteen", "Nineteen"};
// Array for multiples of ten
String Tens_Numbers[] = {
"", "Twenty", "Thirty", "Forty", "Fifty", "Sixty", "Seventy", "Eighty", "Ninety"};
// If the number is less than 20.
if (Long_Number < 20L)
return (FirstTwenty_Numbers[(int) Long_Number]);
String Result = "";
for (long x = Long_Number; x > 0; x %= Number_Limit, Number_Limit /= 1000) {
// the current accessible limit
Current_Limit = x / Number_Limit;
// the current multiplier may be bigger than your number
while (Current_Limit == 0) {
// Set x as the remainder obtained when the number was divided by the limit
x %= Number_Limit;
// Shift the multiplier by dividing the limit by 1000
Number_Limit /= 1000;
// Get the current value in hundreds.
Current_Limit = x / Number_Limit;
// multiplier shifting
++M;
}
// Add the hundreds' place if the current hundred is greater than 99,
if (Current_Limit > 99)
Result += (FirstTwenty_Numbers[(int) Current_Limit / 100] + " Hundred ");
// Bring the current hundred to tens
Current_Limit = Current_Limit % 100;
// If the value in tens belongs to 1 to 19, add using the FirstTwenty_Numbers
if (Current_Limit > 0 && Current_Limit < 20)
Result += (FirstTwenty_Numbers[(int) Current_Limit] + " ");
// If the current limit is now a multiple of 10, pass the tens
else if (Current_Limit % 10 == 0 && Current_Limit != 0)
Result += (Tens_Numbers[(int) Current_Limit / 10 - 1] + " ");
// If the value is between 20 to 99, print using the FirstTwenty_Numbers
else if (Current_Limit > 20 && Current_Limit < 100)
Result += (Tens_Numbers[(int) Current_Limit / 10 - 1] + " "
+ FirstTwenty_Numbers[(int) Current_Limit % 10] + " ");
// shift the multiplier is it has not become less than 1000
if (M < 4)
Result += (Multiplier_Array[(int) ++M] + " ");
}
return (Result);
}
public static void main(String args[]) {
long InputNumber = 70000000000121L;
System.out.println(InputNumber + " = " + LongNumberstoWords(InputNumber) + "\n");
InputNumber = 987654321;
System.out.println(InputNumber + " = " + LongNumberstoWords(InputNumber) + "\n");
InputNumber = 90807060540001L;
System.out.println(InputNumber + " = " + LongNumberstoWords(InputNumber) + "\n");
InputNumber = 909090909090909L;
System.out.println(InputNumber + " = " + LongNumberstoWords(InputNumber) + "\n");
}
}
The code above will convert the long number to 15 digits into words. See the output:
70000000000121 = Seventy Trillion One Hundred Twenty One
987654321 = Nine Hundred Eighty Seven Million Six Hundred Fifty Four Thousand Three Hundred Twenty One
90807060540001 = Ninety Trillion Eight Hundred Seven Billion Sixty Million Five Hundred Forty Thousand One
909090909090909 = Nine Hundred Nine Trillion Ninety Billion Nine Hundred Nine Million Ninety Thousand Nine Hundred Nine
Sheeraz is a Doctorate fellow in Computer Science at Northwestern Polytechnical University, Xian, China. He has 7 years of Software Development experience in AI, Web, Database, and Desktop technologies. He writes tutorials in Java, PHP, Python, GoLang, R, etc., to help beginners learn the field of Computer Science.
LinkedIn Facebook