How to Generate a Random Number Between 1 and 10 in Java
-
Use
java.util.Random
to Generate a Random Number Between1
and10
-
Use
Math.random()
to Generate Random Numbers Between1
to10
-
Use
ThreadLocalRandom
to Generate Random Numbers Between1
to10
- Conclusion
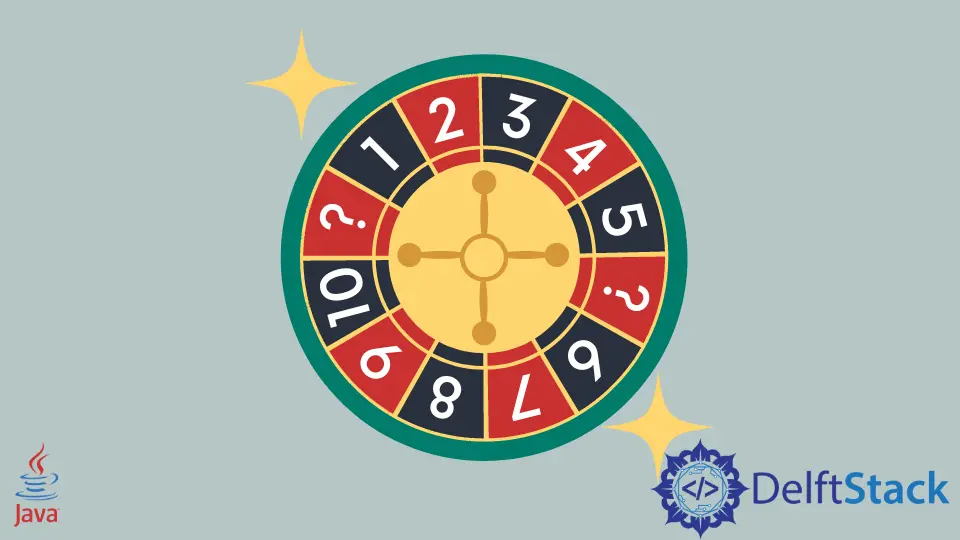
Random number generation is a fundamental aspect of programming, essential for applications ranging from game development to simulations. In Java, developers have multiple tools at their disposal to introduce randomness into their code.
This article explores three distinct methods for generating random numbers between 1
and 10
in Java, each offering unique advantages.
Use java.util.Random
to Generate a Random Number Between 1
and 10
The java.util.Random
package in Java equips developers with a powerful tool for generating random numbers within specified ranges.
Here, we’ll focus on using the Random
class from this package to generate random numbers between 1
and 10
. This class provides flexibility by supporting various numeric types, such as int
or float
.
The Random
class is initialized by creating an instance named random
. This instance becomes our gateway to the world of randomness.
To generate a random number within our desired range of 1
to 10
, the nextInt
method is employed. This method takes an argument representing the upper bound of the random numbers to be generated.
By adjusting this bound, we control the range of potential outcomes.
Here’s an example:
import java.util.Random;
public class RandomNumberGenerator {
public static void main(String[] args) {
int min = 1;
int max = 10;
Random random = new Random();
int value = random.nextInt(max - min + 1) + min;
System.out.println("Random Number is " + value);
}
}
Output:
Random Number is 10
In the provided code, we establish a range by defining min
and max
variables. The Random
instance, named random
, is then created.
The crucial part is the random.nextInt(max - min + 1) + min;
expression. Here, (max - min + 1)
determines the range of possible values (inclusive), and by adding min
, we shift the range to start from 1
.
The result is a random number between 1
and 10
, inclusive.
Now, to reinforce the effectiveness of this technique, a loop is introduced in the following example. This loop iterates 10
times, generating and displaying a new random number with each iteration.
import java.util.Random;
public class RandomNumberGenerator {
public static void main(String[] args) {
Random random = new Random();
// Generate and display 10 random numbers between 1 and 10
for (int i = 1; i <= 10; i++) {
int value = random.nextInt((10 - 1) + 1) + 1;
System.out.println("Random Number " + i + " is " + value);
}
}
}
Output:
Random Number 1 is 1
Random Number 2 is 8
Random Number 3 is 8
Random Number 4 is 3
Random Number 5 is 1
Random Number 6 is 7
Random Number 7 is 2
Random Number 8 is 2
Random Number 9 is 8
Random Number 10 is 4
As evident from the output, the random numbers exhibit variability and may repeat due to the limited range.
Use Math.random()
to Generate Random Numbers Between 1
to 10
While the java.util.Random
class provides a robust solution for generating random numbers in Java, another approach involves using the Math.random()
method. This method is part of the Math
class in Java and is particularly useful when a more straightforward mechanism for generating random floating-point numbers is required.
Unlike the Random
class, Math.random()
directly returns a random double value between 0
(inclusive) and 1
(exclusive). To achieve a random number within a specific range, we utilize a combination of mathematical operations.
The formula Math.random() * (max - min + 1) + min;
generates a random double between min
and max
. By casting the result to an integer, we obtain a final random integer within the desired range.
See the example below:
public class RandomNumberGenerator {
public static void main(String[] args) {
int min = 1;
int max = 10;
double randomDouble = Math.random();
int value = (int) (randomDouble * (max - min + 1)) + min;
System.out.println("Random Number is " + value);
}
}
Output:
Random Number is 5
Here, we set min
to 1
and max
to 10
, defining the range for our random numbers.
The heart of the operation lies in randomDouble * (max - min + 1) + min;
. This expression multiplies the random double value by the range length and adds the minimum value, effectively shifting the range to 1
(inclusive) through 10
.
By casting the result to an integer, we obtain a random whole number within the specified range.
Now, to showcase the effectiveness and variability of the random number generation using Math.random()
, we can utilize a loop:
public class RandomNumberGenerator {
public static void main(String[] args) {
for (int i = 1; i <= 10; i++) {
double randomDouble = Math.random();
int randomNumber = (int) (randomDouble * 10) + 1;
System.out.println("Random Number " + i + " is " + randomNumber);
}
}
}
Output:
Random Number 1 is 5
Random Number 2 is 9
Random Number 3 is 3
Random Number 4 is 3
Random Number 5 is 6
Random Number 6 is 10
Random Number 7 is 1
Random Number 8 is 1
Random Number 9 is 4
Random Number 10 is 6
This loop generates and prints a new random number in each iteration. As evident from the output, the numbers vary and may repeat due to the limited range.
While both methods achieve the goal of generating random numbers, the Math.random()
approach is more straightforward and suitable when floating-point precision is not a concern. It provides a quick and concise means of introducing randomness into Java applications.
Use ThreadLocalRandom
to Generate Random Numbers Between 1
to 10
For developers working with Java 7 and later, the ThreadLocalRandom
class provides a thread-safe and efficient way to generate random numbers. It is particularly useful in multi-threaded environments, ensuring that each thread obtains its own random number generator instance.
The ThreadLocalRandom
class is part of the java.util.concurrent
package, and its usage involves invoking the current()
method to obtain a thread-local instance of the random number generator. The nextInt(int origin, int bound)
method is then utilized to generate a random integer within the specified range.
The following example illustrates this approach:
import java.util.concurrent.ThreadLocalRandom;
public class RandomNumberGenerator {
public static void main(String[] args) {
int randomNumber = ThreadLocalRandom.current().nextInt(1, 11);
System.out.println("Random Number is " + randomNumber);
}
}
Output:
Random Number is 7
In the provided code, we first import the ThreadLocalRandom
class and use the current()
method to obtain a thread-local instance of the random number generator.
The expression nextInt(1, 11)
generates a random integer between 1
(inclusive) and 11
(exclusive). This method call ensures that the random number generation is thread-safe and avoids contention between multiple threads.
To emphasize the reliability and thread safety of ThreadLocalRandom
, we can employ a loop to generate multiple random values:
import java.util.concurrent.ThreadLocalRandom;
public class RandomNumberGenerator {
public static void main(String[] args) {
for (int i = 1; i <= 10; i++) {
int randomNumber = ThreadLocalRandom.current().nextInt(1, 11);
System.out.println("Random Number " + i + " is " + randomNumber);
}
}
}
Output:
Random Number 1 is 9
Random Number 2 is 9
Random Number 3 is 2
Random Number 4 is 4
Random Number 5 is 6
Random Number 6 is 8
Random Number 7 is 5
Random Number 8 is 5
Random Number 9 is 3
Random Number 10 is 10
This loop iterates ten times, generating and printing a new random number in each iteration. The use of ThreadLocalRandom
ensures that each thread operates independently, resulting in reliable and secure random number generation.
Conclusion
Java provides developers with a range of options for generating random numbers, each tailored to different scenarios. The java.util.Random
class offers flexibility and ease of use, Math.random()
provides a concise approach, and ThreadLocalRandom
ensures thread safety in concurrent environments.
By understanding the syntax and applications of these methods, Java developers can choose the approach that best fits their programming needs, adding a touch of randomness to enhance the dynamism of their applications.
Rupam Saini is an android developer, who also works sometimes as a web developer., He likes to read books and write about various things.
LinkedIn