Java에서 1과 10 사이의 난수를 생성하는 방법
-
random.nextInt()
: 1과 10 사이의 난수 생성 -
Math.random()
을 사용하여 1에서 10 사이의 난수 생성 -
ThreadLocalRandom.current.nextInt()
를 사용하여 1-10 사이의 난수 생성
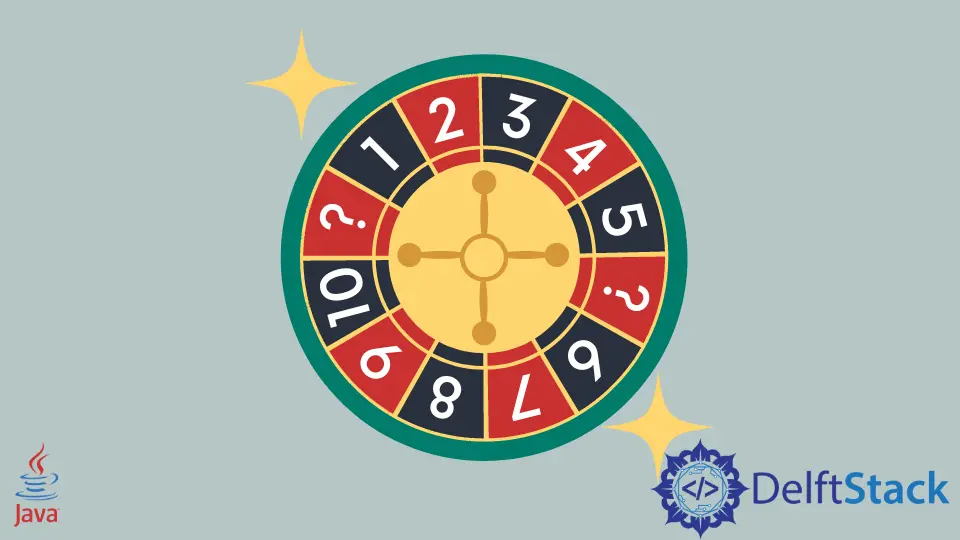
Java에서 임의로 1에서 10 사이의 난수를 생성하는 단계를 살펴 보겠습니다. 1에서 10 사이의 난수를 생성 할 수있는 세 개의 Java 패키지 또는 클래스와 그중 어느 것이 사용하기에 가장 적합한 지 확인할 수 있습니다.
random.nextInt()
: 1과 10 사이의 난수 생성
java.util.Random
은 Java와 함께 제공되는 패키지로 범위 사이에 임의의 숫자를 생성하는 데 사용할 수 있습니다. 우리의 경우 범위는 1에서 10입니다.
이 패키지에는int
든float
든 여러 유형의 숫자를 생성 할 수있는Random
클래스가 있습니다. 더 잘 이해하려면 예제를 확인하십시오.
import java.util.Random;
public class Main {
public static void main(String[] args) {
int min = 1;
int max = 10;
Random random = new Random();
int value = random.nextInt(max + min) + min;
System.out.println(value);
}
}
출력:
6
위의 기술이 작동하고 매번 난수를 생성한다는 것을 보여주기 위해 루프를 사용하여 완료 될 때까지 새로운 난수를 생성 할 수 있습니다. 숫자의 범위가 크지 않기 때문에 임의의 숫자가 반복 될 수 있습니다.
import java.util.Random;
public class Main {
public static void main(String[] args) {
Random random = new Random();
for (int i = 1; i <= 10; i++) {
int value = random.nextInt((10 - 1) + 1) + 1;
System.out.println(value);
}
}
출력:
10
7
2
9
2
7
6
4
9
Math.random()
을 사용하여 1에서 10 사이의 난수 생성
우리의 목표를 달성하는 데 도움이되는 또 다른 클래스는 숫자를 무작위 화하는 여러 정적 함수가있는 수학입니다. random()
메서드를 사용할 것입니다. float
유형의 임의 값을 반환합니다. 이것이 우리가 그것을int
로 캐스트해야하는 이유입니다.
public class Main {
public static void main(String[] args) {
int min = 1;
int max = 10;
for (int i = min; i <= max; i++) {
int getRandomValue = (int) (Math.random() * (max - min)) + min;
System.out.println(getRandomValue);
}
}
출력:
5
5
2
1
6
9
3
6
5
7
ThreadLocalRandom.current.nextInt()
를 사용하여 1-10 사이의 난수 생성
1에서 10 사이의 난수를 얻는 마지막 방법은 다중 스레드 프로그램을 위해 JDK 7에 도입 된ThreadLocalRandom
클래스를 사용하는 것입니다.
현재 스레드에서 난수를 생성하기를 원하기 때문에 클래스의current()
메서드를 호출해야한다는 것을 아래에서 볼 수 있습니다.
import java.util.concurrent.ThreadLocalRandom;
public class Main {
public static void main(String[] args) {
int min = 1;
int max = 10;
for (int i = 1; i <= 10; i++) {
int getRandomValue = ThreadLocalRandom.current().nextInt(min, max) + min;
System.out.println(getRandomValue);
}
}
}
출력:
3
4
5
8
6
2
6
10
6
2
Rupam Saini is an android developer, who also works sometimes as a web developer., He likes to read books and write about various things.
LinkedIn