How to Generate Random Character in Java
-
Generate Random Character Using
random.nextInt()
in Java -
Generate Random Character From a String Using
random.nextInt()
andcharAt()
-
Generate Random Character Using
RandomStringUtils
of Apache Commons
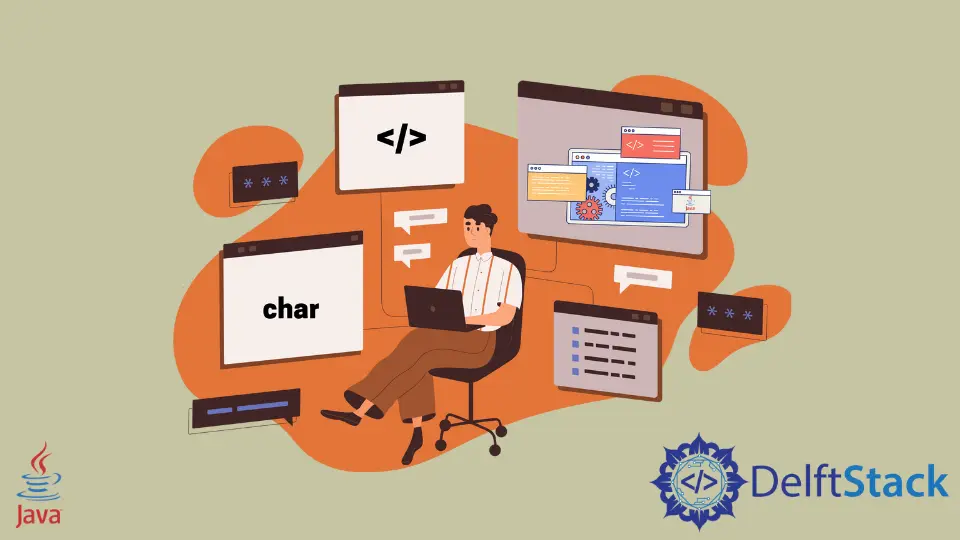
In this tutorial, we will introduce how we can generate a random character using several methods. We will introduce three methods with examples to understand the topic better.
Generate Random Character Using random.nextInt()
in Java
Random
is the most commonly used class in Java to generate a random value, but it cannot generate characters. To randomize characters using the Random
class, we can use random.nextInt()
to generate random integers. Every character corresponds to a number.
We can use a character as a bound in the nextInt()
function. In the following example, we can see that an object of the Random
class is created and then (random.nextInt(26) + 'a')
is used. Here, the character 'a'
corresponds to the number 97, using which we can generate 26 random integers that correspond to the lower-case alphabet.
At last, we have to cast the generated integer to a char.
import java.util.Random;
public class RandomChar {
public static void main(String[] args) {
Random random = new Random();
char randomizedCharacter = (char) (random.nextInt(26) + 'a');
System.out.println("Generated Random Character: " + randomizedCharacter);
}
}
Output:
Generated Random Character: p
Generate Random Character From a String Using random.nextInt()
and charAt()
In the next example, we will again use the Random
class, and its nextInt()
method but generate a random character from an already defined set of characters.
Below we can see that setOfCharacters
is a string with several characters, including alphabets, numbers, and symbols. To generate a random character from this string, we will use the length of setOfCharacters
as the argument of random.nextInt()
. Once a random integer is generated, we use it to get a character at a random index or position using charAt()
. It will return a random char
from setOfCharacters
.
import java.util.Random;
public class RandomChar {
public static void main(String[] args) {
Random random = new Random();
String setOfCharacters = "abcdefghxyz1234567-/@";
int randomInt = random.nextInt(setOfCharacters.length());
char randomChar = setOfCharacters.charAt(randomInt);
System.out.println("Random character from string: " + randomChar);
}
}
Output:
Random character from string: 4
Generate Random Character Using RandomStringUtils
of Apache Commons
In the last example, we use a class RandomStringUtils
from the Apache Commons library. RandomStringUtils
class has a function randomAlphanumeric()
to generate random alphanumeric strings. randomAlphanumeric(count)
takes a single argument, that is the length of the randomized string that we want.
In the example below, we have passed only 1 to the randomAlphanumeric()
method as we only want a single character. But this function returns the result as a string. This is why we have to convert it to a char
. So, we use charAt()
to get the character from the string.
import org.apache.commons.lang3.RandomStringUtils;
public class RandomChar {
public static void main(String[] args) {
String randomizedString = RandomStringUtils.randomAlphanumeric(1);
char randomizedCharacter = randomizedString.charAt(0);
System.out.println("Generated Random Character: " + randomizedCharacter);
}
}
Output:
Generated Random Character: L
Rupam Saini is an android developer, who also works sometimes as a web developer., He likes to read books and write about various things.
LinkedIn