How to Print 2D Array in Java
-
Print 2D Array in Java Using
Arrays.deepToString()
-
Print 2D Array Using Nested
for-each
Loops in Java -
Print 2D Array Using Nested
for
Loops in Java
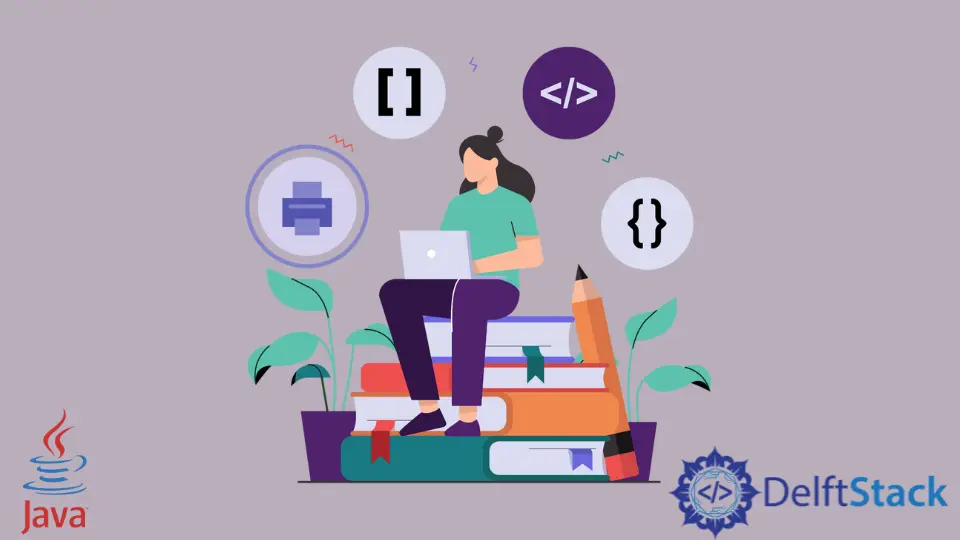
This tutorial discusses methods to print 2D arrays in Java. There are several ways to print 2D arrays in Java. Below we discuss each of these methods in detail.
Print 2D Array in Java Using Arrays.deepToString()
The Arrays
class provides a built-in method Arrays.deepToString()
to display a 2D array. The below example illustrates how to use this method.
import java.util.*;
public class MyClass {
public static void main(String args[]) {
// Create a 2D array
int rows = 3;
int columns = 2;
int[][] array = new int[rows][columns];
for (int i = 0; i < rows; i++) {
for (int j = 0; j < columns; j++) array[i][j] = 0;
}
// Print the 2D array
System.out.println(Arrays.deepToString(array));
}
}
Output:
[[0, 0], [0, 0], [0, 0]]
It is the simplest method to print the content of a 2D array in Java.
Print 2D Array Using Nested for-each
Loops in Java
This method uses the for-each
loops twice to iterate over the 2D array. The below example illustrates this.
import java.util.*;
public class MyClass {
public static void main(String args[]) {
int rows = 3;
int columns = 2;
int[][] array = new int[rows][columns];
for (int i = 0; i < rows; i++) {
for (int j = 0; j < columns; j++) array[i][j] = 0;
}
for (int[] x : array) {
for (int y : x) {
System.out.print(y + " ");
}
System.out.println();
}
}
}
Output:
0 0
0 0
0 0
A 2D array is essentially an array of arrays. In the above example, the first for
loop loops over each array in the 2D array (which is equivalent to a row in a 2D array) while the nested second for
loop iterates over the individual elements (which is equivalent to column values of the particular row).
Print 2D Array Using Nested for
Loops in Java
This method uses two nested for
loops to iterate over the 2D array and print the contents. The below example illustrates this.
import java.util.*;
public class MyClass {
public static void main(String args[]) {
int rows = 3;
int columns = 2;
int[][] array = new int[rows][columns];
for (int i = 0; i < rows; i++) {
for (int j = 0; j < columns; j++) array[i][j] = 0;
}
for (int i = 0; i < array.length; i++) {
for (int j = 0; j < array[i].length; j++) {
System.out.print(array[i][j] + " ");
}
System.out.println();
}
}
}
Output:
0 0
0 0
0 0
This method is just a different variant of the nested for-each
loops. We iterate over every column for each row and print the element at that position.