How to Fix Java Invalid Method Declaration; Return Type Required
- Components of a Method Declaration
-
Fix
Invalid method declaration; return type required
in Java - Conclusion
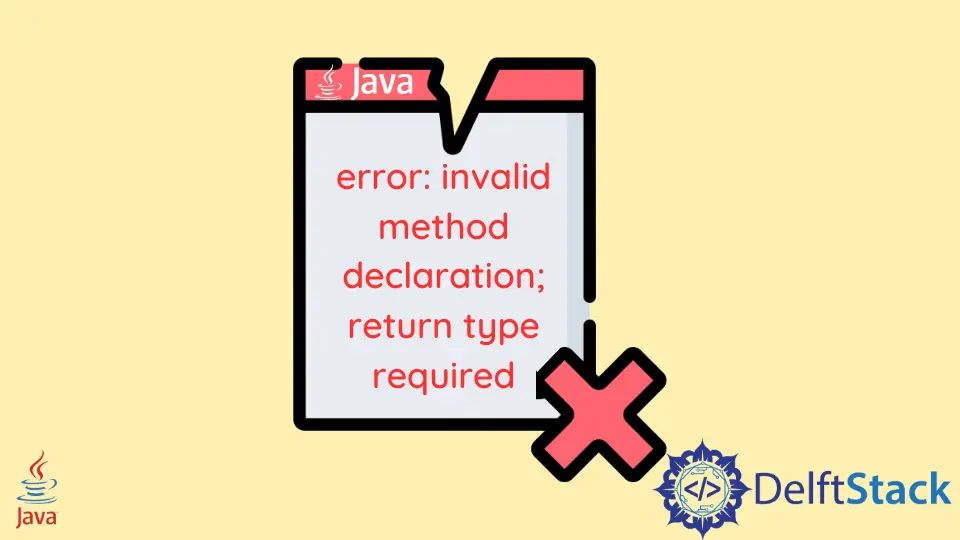
The invalid method declaration; return type required
error appears in Java when a method is declared without specifying a return type, violating the language’s syntax requirement that every method must explicitly declare its return type.
Resolving the invalid method declaration; return type required
error is crucial for maintaining the syntactic integrity and functionality of Java code. Failing to address this error can lead to compilation failures and hinder the execution of the program.
By promptly resolving this issue, developers ensure that their code adheres to Java’s syntax requirements, promoting clarity, readability, and, ultimately, the successful compilation and execution of their programs.
Components of a Method Declaration
Understanding the components of a method declaration is crucial to preventing the invalid method declaration; return type required
error in Java. This error typically occurs when there’s a deviation from the expected syntax of a method declaration, and having a clear understanding of its components helps ensure adherence to Java’s rules.
Component | Description |
---|---|
Access Modifier | Specifies the visibility of the method (e.g., public , private , protected , or package-private). If no modifier is present, it defaults to package-private . |
Return Type | Indicates the type of value the method returns (void if it returns nothing or a specific data type otherwise). |
Method Name | The unique identifier for the method, following Java’s identifier naming conventions. |
By having a comprehensive understanding of these components, developers can proactively structure their method declarations according to Java’s syntax rules. This not only prevents the invalid method declaration; return type required
error but also contributes to writing clean, readable, and error-free code.
Consistent adherence to these principles enhances code maintainability and facilitates collaboration among developers working on the same codebase.
Fix Invalid method declaration; return type required
in Java
Case 1: Missing Return Type
A missing return type in Java occurs when a method fails to specify the type of value it should return. In Java, every method must declare its return type explicitly, indicating the kind of data it will return (such as int
, String
, or void
for no return value).
If the return type is omitted or improperly declared, the compiler flags an error known as the missing return type
error. This ensures clarity and consistency, enabling the compiler to enforce proper method usage and facilitating correct program execution.
Let’s start by examining a simple Java class that triggers the invalid method declaration
error:
public class InvalidMethodExample {
// Method without a return type
public myMethod() {
System.out.println("Hello, Java!");
}
// Main method to demonstrate the issue
public static void main(String[] args) {
InvalidMethodExample example = new InvalidMethodExample();
example.myMethod();
}
}
In the provided code snippet, we have a class named InvalidMethodExample
containing a method named myMethod
. The issue arises because the myMethod
lacks a return type.
Let’s break down the code to understand why this triggers the invalid method declaration
error.
public myMethod() {
System.out.println("Hello, Java!");
}
In this method declaration, the keyword public
indicates that the method is accessible from outside the class. However, the absence of a return type before the method name (myMethod
) is the root cause of the error.
The main
method attempts to invoke the myMethod
from an instance of the InvalidMethodExample
class. This is where the compiler flags the error due to the missing return type.
To fix the invalid method declaration
error, we need to provide a valid return type for the myMethod
. Let’s modify the code:
public class ValidMethodExample {
// Method with a void return type
public void myMethod() {
System.out.println("Hello, Java!");
}
// Main method to demonstrate the resolution
public static void main(String[] args) {
ValidMethodExample example = new ValidMethodExample();
example.myMethod();
}
}
Now, the myMethod
is declared with a return type of void
, indicating that the method does not return any value. This aligns with the fact that the method prints a message but doesn’t return a result.
The corrected method signature is as follows:
public void myMethod() {
System.out.println("Hello, Java!");
}
By providing a proper return type in the method declaration, we successfully resolve the invalid method declaration; return type required
error.
When the corrected code is executed, the output will be:
Hello, Java!
This demonstrates that the method is now correctly defined and executes without errors, reinforcing the effectiveness of the resolution.
Case 2: Incorrect Method Signature
An incorrect method signature in Java refers to a deviation from the expected format when declaring a method. This includes factors like missing essential components (such as the method name, return type, or parameters) or using incorrect syntax.
The method signature defines the method’s unique identity, comprising its name, return type, and parameter types. Deviating from this prescribed structure triggers a compilation error, hindering the proper functioning of the program.
Ensuring a correct method signature is essential for maintaining syntactic integrity and enabling successful compilation and execution in Java.
Let’s dive into an illustrative code snippet that triggers the invalid method declaration
error:
public class InvalidSignatureExample {
// Method with an incorrect signature
public myMethod() {
System.out.println("Hello, Java!");
}
// Main method to demonstrate the issue
public static void main(String[] args) {
InvalidSignatureExample example = new InvalidSignatureExample();
example.myMethod();
}
}
In this code snippet, we have a class named InvalidSignatureExample
containing a method named myMethod
. The source of the error lies in the method signature:
public myMethod() {
System.out.println("Hello, Java!");
}
Here, the myMethod
lacks a proper return type declaration. Let’s break down why this triggers the invalid method declaration
error.
In the method declaration of myMethod
, the absence of a specified return type violates Java’s requirement that every method must explicitly declare its return type. This violation prompts the compiler to flag the error.
However, in the main
method, due to the incomplete method declaration of myMethod
lacking a return type, the compiler detects this issue before execution, leading to the occurrence of the error.
In solving this error, we need to provide a valid return type in the method signature. Let’s correct the code:
public class ValidSignatureExample {
// Method with a void return type
public void myMethod() {
System.out.println("Hello, Java!");
}
// Main method to demonstrate the resolution
public static void main(String[] args) {
ValidSignatureExample example = new ValidSignatureExample();
example.myMethod();
}
}
Now, the myMethod
is correctly declared with a return type of void
, indicating that the method does not return any value. This aligns with the fact that the method prints a message but doesn’t return a result. The corrected method signature is as follows:
public void myMethod() {
System.out.println("Hello, Java!");
}
By addressing the incorrect method signature, we successfully eliminate the invalid method declaration; return type required
error.
When the corrected code is executed, the output will be:
Hello, Java!
This demonstrates that the method is now correctly defined and executes without errors, showcasing the effectiveness of resolving the incorrect method signature.
Case 3: Incorrect Return Type
An incorrect return type in Java occurs when a method’s declared return type does not match the actual type of the value returned. Java mandates consistency between the specified return type and the data returned by the method.
If this alignment is disrupted, leading to a mismatch, a compilation error, often termed incorrect return type
, arises. Ensuring harmony between the declared return type and the actual value is crucial for accurate compilation and program execution, promoting code reliability and adherence to Java’s syntax rules.
Let’s see a code snippet that manifests the invalid method declaration
error:
public class InvalidReturnTypeExample {
// Method with an incorrect return type
public int myMethod() {
return "Hello!\n~From Delftstack";
}
// Main method to demonstrate the issue
public static void main(String[] args) {
InvalidReturnTypeExample example = new InvalidReturnTypeExample();
String s = myMethod();
System.out.println(s);
}
}
In this code snippet, the class InvalidReturnTypeExample
contains a method named myMethod
with a significant flaw in its return type declaration:
public int myMethod() {
return "Hello!\n~From Delftstack";
}
The error stems from an inconsistency in the return type declaration of the myMethod
. Although it asserts to return an int
, there is no corresponding return
statement with an actual integer value.
This mismatch prompts the compiler to identify the error, expecting a return type in line with the declared int
.
Consequently, when the main
method invokes myMethod
within the InvalidReturnTypeExample
class, the absence of a correct return type disrupts the compilation process. The error surfaces before execution, emphasizing the importance of aligning declared return types with the actual content of the method to ensure smooth compilation and execution.
To address this error and correct the code, you should ensure that the return type in the method declaration matches the actual type of the value being returned. In this case, since the method is returning a string, the correct return type should be String
.
Here’s the corrected code:
public class CorrectedReturnTypeExample {
// Method with the correct return type
public String myMethod() {
return "Hello!\n~From Delfstack";
}
// Main method to demonstrate the resolution
public static void main(String[] args) {
CorrectedReturnTypeExample example = new CorrectedReturnTypeExample();
String s = example.myMethod(); // corrected method invocation
System.out.println(s);
}
}
In this corrected code, the myMethod
is declared to return a String
, aligning with the actual return statement.
Additionally, when invoking the method in the main
method, it should be done through an instance of the class (example.myMethod()
), as the method is not declared static. This ensures that the declared return type matches the actual type returned by the method, resolving the invalid method declaration; return type required
error.
When the corrected code is executed, the output will be:
Hello, Java!
~From Delftstack
This output confirms that the method is now accurately defined, executes without errors, and underscores the effectiveness of resolving the incorrect return type.
Case 4: Missing Method Name
A missing method name in Java denotes the absence of a valid identifier for a declared method. Java syntax requires every method to have a unique and properly named identifier, following modifiers like public void
or similar.
When a method lacks a valid name, it triggers a compilation error known as the missing method name
error. This issue disrupts the syntactic structure, preventing the compiler from recognizing and processing the method correctly.
Resolving this error involves providing a valid and descriptive name for the method, ensuring compliance with Java’s naming conventions for method identifiers.
Let’s delve into a code snippet that exemplifies the invalid method declaration
error, specifically caused by a missing method name:
public class InvalidMethodNameExample {
// Method without a name
public void() {
System.out.println("Hello, Java!");
}
// Main method to demonstrate the issue
public static void main(String[] args) {
InvalidMethodNameExample example = new InvalidMethodNameExample();
example.myMethod(); // Invoking the non-existent method
}
}
In this code snippet, the class InvalidMethodNameExample
contains a method that lacks a name:
public void() {
System.out.println("Hello, Java!");
}
Here, the absence of a method name is the root cause of the invalid method declaration
error.
The error in the given code arises from a missing method name within the InvalidMethodNameExample
class.
In Java, every method declaration must include a valid name following modifiers such as public void
. The absence of a proper method name violates this syntax rule, leading to the invalid method declaration
error.
When the main
method attempts to invoke the non-existent method (myMethod
) using an instance of InvalidMethodNameExample
, a compilation error occurs. This error is attributed to the missing method name in the class, emphasizing the necessity of adhering to Java’s syntax conventions by providing a valid name for every method.
To resolve this error, we need to provide a valid name for the method. Let’s correct the code:
public class ValidMethodNameExample {
// Method with a valid name
public void myMethod() {
System.out.println("Hello, Java!");
}
// Main method to demonstrate the resolution
public static void main(String[] args) {
ValidMethodNameExample example = new ValidMethodNameExample();
example.myMethod();
}
}
Now, the method myMethod
is correctly declared with a valid name:
public void myMethod() {
System.out.println("Hello, Java!");
}
By addressing the missing method name, we successfully eliminate the invalid method declaration; return type required
error.
When the corrected code is executed, the output will be:
Hello, Java!
This output confirms that the method is now correctly defined, executes without errors, and highlights the effectiveness of resolving the missing method name.
Case 5: Spelling Error
A spelling error in a Java method occurs when a word within the code is misspelled, deviating from the correct syntax. Java is case-sensitive, so even a small typographical mistake can lead to compilation errors.
In the context of a method, a misspelled method name, parameter, or keyword can result in the compiler being unable to recognize and interpret the intended code, leading to issues like the invalid method declaration
error.
Resolving this error involves carefully reviewing and correcting the spelling mistake to ensure accurate interpretation by the Java compiler.
Let’s explore a code snippet where a simple typo leads to the invalid method declaration
error:
public class TypoExample {
// Method with a typo in the method name
public static void myMehod() {
System.out.println("Hello, Java!");
}
// Main method to demonstrate the issue
public static void main(String[] args) {
myMethod(); // Invoking the method with the typo
}
}
In this code snippet, the class TypoExample
contains a method named myMehod
with a subtle typo:
public static void myMehod() {
System.out.println("Hello, Java!");
}
Here, the typo in the method name (myMehod
instead of myMethod
) results in the invalid method declaration
error.
The error in the provided code is due to a typo in the method name declaration. Specifically, the method is declared with the name myMehod
instead of the correct name myMethod
.
Notably, Java is case-sensitive, meaning that myMehod
and myMethod
are distinct identifiers. Consequently, when the main
method tries to invoke the method using the incorrect name (myMehod
), the compiler detects the error.
This results in a compilation error due to the non-existent method name, emphasizing the importance of precise naming and adherence to case sensitivity in Java to avoid such errors.
To resolve this error, we need to correct the typo in the method name. Let’s rectify the code:
public class CorrectedExample {
// Method with the correct name
public static void myMehod() {
System.out.println("Hello, Java!");
}
// Main method to demonstrate the resolution
public static void main(String[] args) {
myMethod(); // Invoking the corrected method
}
}
Now, the method is correctly named myMethod
:
public static void myMethod() {
System.out.println("Hello, Java!");
}
By rectifying the typo in the method name, we successfully eliminated the invalid method declaration; return type required
error. Paying attention to such details enhances code clarity and reduces the likelihood of syntax-related errors.
When the corrected code is executed, the output will be:
Hello, Java!
This output confirms that the method is now correctly named, executes without errors, and underscores the effectiveness of fixing typos in method declarations.
Case 6: Missing Comma in enum
Declaration
A missing comma in a Java enum
declaration signifies the absence of a necessary separator between individual constants. Enums
require commas between each constant to maintain proper syntax.
If a comma is omitted, it disrupts the enum
structure, causing subsequent constants to be misinterpreted. This leads to compilation errors, particularly affecting methods using the enum
.
Rectifying this issue involves adding the missing commas to ensure a correct enum
definition, preventing compilation errors, and ensuring the enum
is accurately interpreted and utilized throughout the Java code.
Let’s dive into a code snippet where a missing comma in an enum
declaration leads to the invalid method declaration
error:
public class MissingCommaExample {
// Enum with a missing comma
public enum Days { MONDAY, TUESDAY WEDNESDAY, THURSDAY, FRIDAY }
// Method using the enum
public void printDay(Days day) {
System.out.println("Today is " + day);
}
// Main method to demonstrate the issue
public static void main(String[] args) {
MissingCommaExample example = new MissingCommaExample();
example.printDay(Days.WEDNESDAY);
}
}
In this code snippet, we have an enum
named Days
representing the days of the week:
public enum Days { MONDAY, TUESDAY WEDNESDAY, THURSDAY, FRIDAY }
Notice the missing comma between TUESDAY
and WEDNESDAY
. This seemingly innocent typo can lead to the invalid method declaration
error.
In this instance, the absence of a comma disrupts the structure of the enum
, causing the subsequent constant, WEDNESDAY
, to be incorrectly interpreted, resulting in a compilation error.
The impact of this error extends to the printDay
method, which attempts to utilize the Days
enum
. However, due to the error in the enum
declaration, a ripple effect occurs, leading to the manifestation of the invalid method declaration
error.
The error surfaces as a consequence of the disrupted structure in the enum
, emphasizing the importance of proper syntax adherence, especially when defining enumerations, to prevent cascading errors in the code.
To resolve this error, we need to correct the enum
declaration by adding the missing comma. Let’s rectify the code:
public class CorrectedExample {
// Enum with the corrected comma
public enum Days { MONDAY, TUESDAY, WEDNESDAY, THURSDAY, FRIDAY }
// Method using the corrected enum
public void printDay(Days day) {
System.out.println("Today is " + day);
}
// Main method to demonstrate the resolution
public static void main(String[] args) {
CorrectedExample example = new CorrectedExample();
example.printDay(Days.WEDNESDAY);
}
}
Now, the Days
enum
is correctly declared with the missing comma added:
public enum Days { MONDAY, TUESDAY, WEDNESDAY, THURSDAY, FRIDAY }
By ensuring proper syntax in enum
declarations, we successfully eliminate the invalid method declaration; return type required
error.
When the corrected code is executed, the output will be:
Today is WEDNESDAY
This output confirms that the corrected enum
is used seamlessly in the printDay
method, emphasizing the effectiveness of fixing the missing comma in enum
declarations. As you work with enums
in Java, meticulous syntax adherence ensures a smooth and error-resistant coding experience.
Conclusion
The invalid method declaration; return type required
error can result from various issues: missing return types, incorrect signatures, incorrect return types, missing method names, typos, and missing commas in enums
. These errors highlight the importance of precise syntax adherence in Java coding.
Resolving each issue involves meticulous attention to detail, ensuring accurate method declarations, correct signatures, and proper enum
syntax. By addressing these elements, developers can create robust and error-free Java code, promoting clarity, preventing compilation issues, and facilitating smooth program execution.
Haider specializes in technical writing. He has a solid background in computer science that allows him to create engaging, original, and compelling technical tutorials. In his free time, he enjoys adding new skills to his repertoire and watching Netflix.
LinkedInRelated Article - Java Function
- How to Return a Boolean Method in Java
- Covariant Return Type in Java
- How to Write an Anonymous Function in Java
- How to Use System.gc() for Garbage Collection in Java
- How to Create Callback Functions in Java
- How to Return Nothing From a Function in Java
Related Article - Java Error
- How to Fix the Error: Failed to Create the Java Virtual Machine
- How to Fix the Missing Server JVM Error in Java
- How to Fix the 'No Java Virtual Machine Was Found' Error in Eclipse
- How to Fix Javax.Net.SSL.SSLHandShakeException: Remote Host Closed Connection During Handshake
- How to Fix the Error: Failed to Create the Java Virtual Machine
- How to Fix Java.Lang.VerifyError: Bad Type on Operand Stack