How to Initialize All Array Elements to Zero in Java
-
Initialize Array Elements to Zero by Using a
for
Loop -
Initialize Array Elements to Zero by Using the
fill()
Method in Java -
Initialize Array Elements to Zero by Using the
nCopies()
Method - Initialize Array Elements to Zero by Using Reassignment in Java
-
Initialize Array Elements to Zero by Using
Arrays.setAll()
- Conclusion
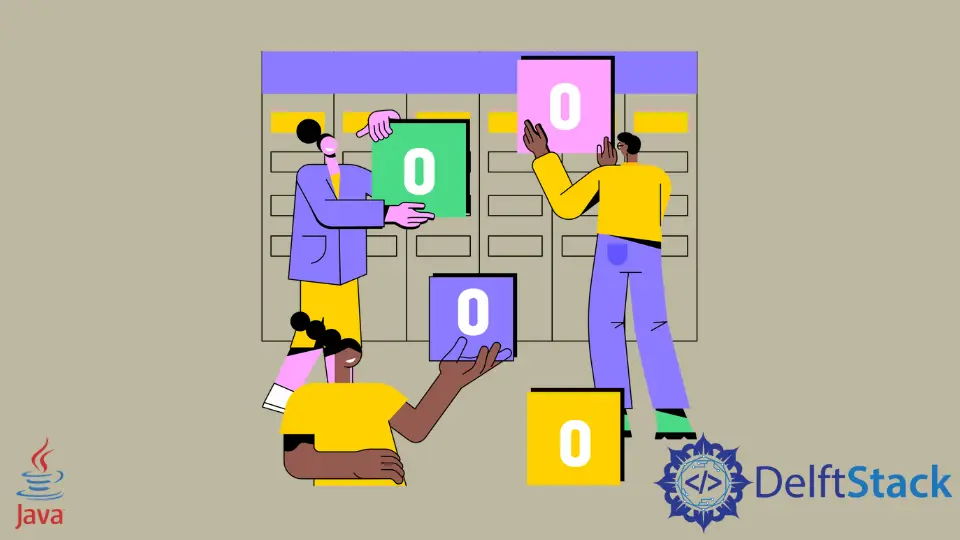
This tutorial promises a comprehensive exploration of an array of re-initialization techniques, providing practical knowledge that can be applied in real-world Java development scenarios.
Reinitializing an array to zero is crucial for establishing a clean starting point in programming. It ensures a predictable state, preventing unintended data interference and offering a fresh slate for computations.
This practice is particularly vital in scenarios where accurate and consistent results depend on a well-defined initial state. Whether preparing for new data or recalculating values, the process of resetting an array to zero enhances code reliability and contributes to the overall stability and predictability of the program.
In this article, readers can look forward to gaining a comprehensive understanding of various methods to re-initialize an array to zero in Java. The content will cover multiple techniques, including the use of for
loops, Arrays.fill()
, nCopies()
, reassignment, and Arrays.setAll()
.
Each method will be explained in a clear and concise manner, providing both novice and experienced Java developers with valuable insights.
Initialize Array Elements to Zero by Using a for
Loop
The for
loop method is straightforward, making the code easy to understand and maintain. It provides a clear and explicit way to iterate through array elements, setting each one to zero.
While other methods may offer concise solutions, the for
loop ensures transparency in the re-initialization process, making it ideal for scenarios where explicit control and simplicity are paramount.
Let’s dive into a practical example to demonstrate how to re-initialize an array to zero using a for
loop in Java:
import java.util.Arrays;
public class ArrayZeroInitializer {
public static void main(String[] args) {
// Declare and initialize an array
int[] numbers = {1, 2, 3, 4, 5, 6, 7, 8, 9, 10};
// Display the original array
System.out.println("Original Array: " + Arrays.toString(numbers));
// Re-initialize array elements to zero using a for loop
for (int i = 0; i < numbers.length; i++) {
numbers[i] = 0;
}
// Display the array after re-initialization
System.out.println("Array After Re-initialization: " + Arrays.toString(numbers));
}
}
In the first step of our process, we declare an array named numbers
and initialize it with specific values. This initial state is essential, serving as the starting point before any re-initialization occurs.
Following this, we utilize the Arrays.toString()
method to print the original array, providing a clear representation of its initial contents.
Moving on to the re-initialization phase, a for
loop is employed to iterate through each element of the array, setting each element to zero. This step is crucial in resetting the array to the desired state.
Finally, we print the modified array, confirming that all elements have been successfully re-initialized to zero. This comprehensive explanation breaks down each aspect of the code, offering a clear understanding of the process involved in re-initializing the array using a for
loop in Java.
The output of the provided code will demonstrate the transition of the array from its original state to being entirely populated with zeros:
Original Array: [1, 2, 3, 4, 5, 6, 7, 8, 9, 10]
Array After Re-initialization: [0, 0, 0, 0, 0, 0, 0, 0, 0, 0]
This clearly illustrates the successful re-initialization of the array, ensuring that each element is set to zero, as intended.
Initialize Array Elements to Zero by Using the fill()
Method in Java
The Arrays.fill()
method streamlines the process into a single line of code, making it easy to read and understand. It eliminates the need for explicit loops or complex logic, resulting in cleaner and more concise code.
In Java, the fill()
method is a part of the Arrays
class, and it is used to fill an array or a range of an array with a specified value. Here’s the syntax for the fill()
method:
public static void fill(Object[] array, Object value)
The parameter array
denotes the array that is targeted for filling, and the parameter value
represents the specific value that will be assigned to each element within the array.
This method provides a convenient way to initialize or reset the contents of an array by uniformly assigning the specified value to all its elements.
While other methods may require more lines of code, Arrays.fill()
provides an efficient way to set all array elements to zero, contributing to code readability and maintainability.
Let’s delve into a practical example that demonstrates how to re-initialize an array to zero using the Arrays.fill()
method:
import java.util.Arrays;
public class ArrayZeroFiller {
public static void main(String[] args) {
// Declare and initialize an array
int[] numbers = {1, 2, 3, 4, 5, 6, 7, 8, 9, 10};
// Display the original array
System.out.println("Original Array: " + Arrays.toString(numbers));
// Re-initialize array elements to zero using Arrays.fill()
Arrays.fill(numbers, 0);
// Display the array after re-initialization
System.out.println("Array After Re-initialization: " + Arrays.toString(numbers));
}
}
In the first step, we initiate our process by declaring an array called numbers
and initializing it with specific initial values. This serves as the foundational step, establishing the array’s starting state before any re-initialization occurs.
Moving forward, to provide context and a visual representation, we employ the Arrays.toString()
method to print the original array. This action helps us observe and understand the array’s composition before any modifications take place.
The core of the process involves a concise and expressive line of code utilizing the Arrays.fill()
method. This method takes the target array and the value to set for all its elements during re-initialization.
Subsequently, we ensure the success of the re-initialization process by printing the modified array. This final step confirms that all elements have indeed been effectively set to zero.
Executing the provided code will yield the following output, showcasing the transformation of the array from its original state to being entirely populated with zeros:
Original Array: [1, 2, 3, 4, 5, 6, 7, 8, 9, 10]
Array After Re-initialization: [0, 0, 0, 0, 0, 0, 0, 0, 0, 0]
In summary, the explanation breaks down each aspect of the code, providing a clear understanding of the array re-initialization process using the Arrays.fill()
method in Java.
This clear and concise output demonstrates the effectiveness of the Arrays.fill()
method in re-initializing an array to zero, providing a clean slate for subsequent operations.
Initialize Array Elements to Zero by Using the nCopies()
Method
The nCopies()
method, part of the java.util.Collections
class, provides an elegant and concise way to achieve this in Java.
The method allows for a concise one-liner, creating an immutable list with the specified element (zero) and converting it to an array. This simplicity enhances code readability, making it easy to understand at a glance.
In Java, the nCopies()
method is a static method of the Collections
class. This method is used to create an immutable list consisting of n copies of the specified object. Here’s the syntax for the nCopies()
method:
public static <T> List<T> nCopies(int n, T o)
In Java, the nCopies()
method creates an immutable list with a specified number (n
) of copies of a given object (o
). The method is useful for generating lists with repeated elements in a straightforward manner.
The resulting list is fixed and cannot be modified after its creation.
While other methods may involve more lines of code or complexity, nCopies()
provides an elegant solution, streamlining the re-initialization process and contributing to clean and efficient code.
Let’s delve into a practical example that illustrates how to re-initialize an array to zero using the nCopies()
method:
import java.util.Arrays;
import java.util.Collections;
import java.util.List;
public class ArrayZeroReInitializer {
public static void main(String[] args) {
// Declare and initialize an array
Integer[] numbersArray = {1, 2, 3, 4, 5, 6, 7, 8, 9, 10};
// Display the original array
System.out.println("Original Array: " + Arrays.toString(numbersArray));
// Re-initialize array elements to zero using nCopies()
List<Integer> zeroList = Collections.nCopies(numbersArray.length, 0);
Integer[] zeroArray = zeroList.toArray(new Integer[0]);
// Display the array after re-initialization
System.out.println("Array After Re-initialization: " + Arrays.toString(zeroArray));
}
}
In the initial phase, our process commences with the declaration of an array named numbersArray
, which is then initialized with specific initial values. This foundational step sets the stage for the subsequent example.
Moving on, we employ the Arrays.toString()
method to print the original array, providing valuable context by visualizing the array’s state before any re-initialization occurs.
The pivotal part of our process revolves around the utilization of the Collections.nCopies()
method. This method enables the creation of an immutable list containing a specified element, in this case, zero.
The subsequent conversion of this list into an array facilitates the re-initialization of our target array. To validate the success of the re-initialization, we print the modified array, confirming that all elements have been effectively set to zero.
Executing the provided code will yield the following output, showcasing the transformation of the array from its original state to being entirely populated with zeros:
Original Array: [1, 2, 3, 4, 5, 6, 7, 8, 9, 10]
Array After Re-initialization: [0, 0, 0, 0, 0, 0, 0, 0, 0, 0]
In essence, this explanation systematically breaks down each step of the code, elucidating the array re-initialization process using the Collections.nCopies()
method in Java.
This output serves as a clear demonstration of the effectiveness of the nCopies()
method in re-initializing an array to zero, providing a clean slate for subsequent operations.
Initialize Array Elements to Zero by Using Reassignment in Java
The reassignment method involves a simple for
loop that iterates through each array element, setting it to zero. This approach provides direct control over each element, making the code easy to comprehend.
While other methods may offer concise solutions, reassignment ensures transparency in the re-initialization process, making it ideal for scenarios where simplicity and explicit control are key considerations.
The reassignment method involves traversing the array using a loop and individually setting each element to zero. While it may seem straightforward, this method provides fine-grained control over the re-initialization process.
Let’s delve into a practical example that illustrates how to re-initialize an array to zero using the reassignment method:
public class ArrayZeroReassignment {
public static void main(String[] args) {
// Declare and initialize an array
int[] numbers = {1, 2, 3, 4, 5, 6, 7, 8, 9, 10};
// Display the original array
System.out.println("Original Array: ");
printArray(numbers);
// Re-initialize array elements to zero through reassignment
reinitializeArrayToZero(numbers);
// Display the array after re-initialization
System.out.println("Array After Re-initialization: ");
printArray(numbers);
}
// Helper method to print the array
private static void printArray(int[] array) {
for (int element : array) {
System.out.print(element + " ");
}
System.out.println();
}
// Helper method to re-initialize the array to zero through reassignment
private static void reinitializeArrayToZero(int[] array) {
for (int i = 0; i < array.length; i++) {
array[i] = 0;
}
}
}
In the initial step of our process, we embark on declaring an array named numbers
and initializing it with specific initial values. This array serves as the foundational starting point before any re-initialization occurs.
Following this, to provide context and a clear visual representation, we utilize a helper method called printArray
. This method plays a crucial role in displaying the original array, iterating through each element, and printing it on the console.
Moving on to the core of our process, the re-initialization through reassignment, we rely on the reinitializeArrayToZero
helper method. This method utilizes a loop to iterate through the array, systematically reassigning each element to zero.
As a result, the array is effectively reset to the desired state. Finally, we use the printArray
method once again to display the modified array, confirming that all elements have been successfully re-initialized to zero.
Executing the provided code will yield the following output, demonstrating the transformation of the array from its original state to being entirely populated with zeros:
Original Array:
1 2 3 4 5 6 7 8 9 10
Array After Re-initialization:
0 0 0 0 0 0 0 0 0 0
This comprehensive explanation breaks down each aspect of the code, offering a clear understanding of the array re-initialization process using the reassignment method in Java.
This output serves as a clear demonstration of the effectiveness of the reassignment method in re-initializing an array to zero, providing a clean slate for subsequent operations.
Initialize Array Elements to Zero by Using Arrays.setAll()
The Arrays.setAll()
method is a powerful tool introduced in Java 8 that allows developers to succinctly re-initialize array elements based on a specified function.
Re-initializing an array is a common operation in programming, ensuring a known and consistent starting point for subsequent computations. The Arrays.setAll()
method provides an elegant and expressive solution, allowing developers to specify a function that determines the value of each array element during re-initialization.
In Java, the setAll()
method is a part of the Arrays
class, and it is used to set all elements of an array based on the index. Here’s the syntax for the setAll()
method:
public static <T> void setAll(T[] array, IntUnaryOperator generator)
The parameter array
represents the array for which elements will be set, while the generator
parameter is an IntUnaryOperator
that defines how each element is computed based on its index. Essentially, the setAll()
method offers a streamlined way to initialize or modify array elements by applying a specific formula or computation based on the index of each element.
The method allows developers to define the logic for re-initialization through a lambda expression or function. This flexibility is valuable in scenarios where a more complex initialization process is required.
While other methods may provide simplicity, Arrays.setAll()
stands out when explicit control over each element’s value is necessary, contributing to cleaner and more customized code.
Let’s delve into a practical example that illustrates how to re-initialize an array to zero using the Arrays.setAll()
method:
import java.util.Arrays;
public class ArrayZeroInitializer {
public static void main(String[] args) {
// Declare and initialize an array
int[] numbers = {1, 2, 3, 4, 5, 6, 7, 8, 9, 10};
// Display the original array
System.out.println("Original Array: " + Arrays.toString(numbers));
// Re-initialize array elements to zero using Arrays.setAll()
Arrays.setAll(numbers, i -> 0);
// Display the array after re-initialization
System.out.println("Array After Re-initialization: " + Arrays.toString(numbers));
}
}
In the first step of our process, we initiate by declaring an array named numbers
and initializing it with specific initial values. This foundational step sets the stage for our example, providing an initial state for the array before any re-initialization occurs.
Moving forward, to provide context and a visual representation, we utilize the Arrays.toString()
method to print the original array. This step is crucial in helping us observe and understand the array’s composition before any modifications take place.
The core of the process involves a concise and expressive line of code using the Arrays.setAll()
method. This method takes the target array and a lambda expression or function, allowing developers to define the logic for each element’s value during re-initialization.
Subsequently, we ensure the success of the re-initialization process by printing the modified array. This final step confirms that all elements have indeed been effectively set to zero.
Executing the provided code will yield the following output, showcasing the transformation of the array from its original state to being entirely populated with zeros:
Original Array: [1, 2, 3, 4, 5, 6, 7, 8, 9, 10]
Array After Re-initialization: [0, 0, 0, 0, 0, 0, 0, 0, 0, 0]
In summary, the explanation systematically breaks down each aspect of the code, providing a clear understanding of the array re-initialization process using the Arrays.setAll()
method in Java.
This output serves as a clear demonstration of the effectiveness of the Arrays.setAll()
method in re-initializing an array to zero, providing a clean slate for subsequent operations.
Conclusion
Re-initializing an array to zero in Java offers diverse methods, each with its own advantages.
The for
loop method provides simplicity and power, offering a common practice for array manipulation.
On the other hand, using Arrays.fill()
streamlines the process into a single line, enhancing code readability.
The nCopies()
method offers a concise solution, contributing to clean and efficient code.
Reassignment, though more explicit, provides transparency in the re-initialization process.
Lastly, Arrays.setAll()
allows for a defined logic, promoting clarity in code.
As Java developers advance, mastering these methods becomes essential for crafting efficient and maintainable code.