How to Compare String With the Java if Statement
-
Compare String With the Java
if
Statement Using the==
and!=
Operators -
Compare String With the Java
if
Statement Using theequals()
Function -
Compare String With the Java
if
Statement Using thecompareTo()
Function - Conclusion
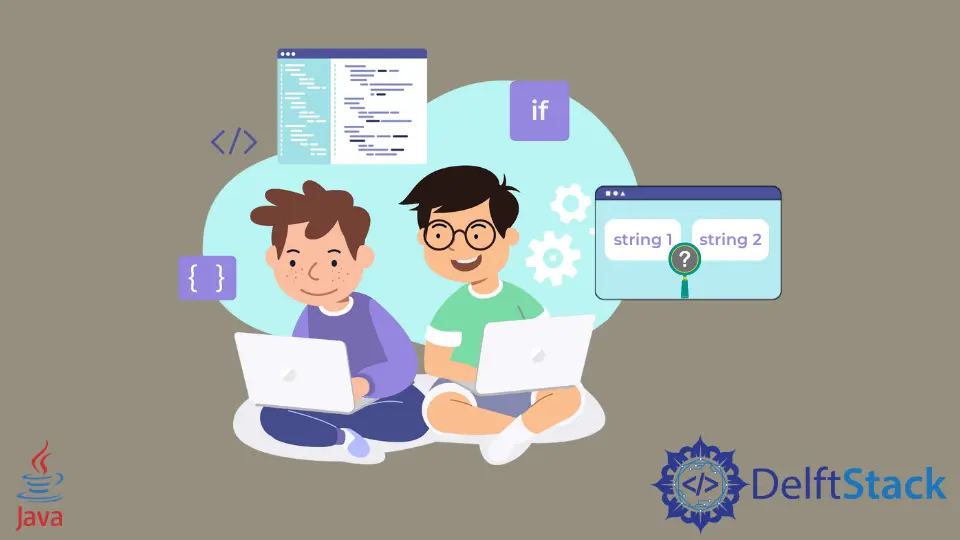
In Java, comparing strings is a common task, and the if
statement plays a crucial role in making such comparisons. This involves assessing the equality or order of strings based on their content or memory references.
In this guide, we’ll explore various methods and operators within the context of using the if
statements for string comparison in Java.
Compare String With the Java if
Statement Using the ==
and !=
Operators
In Java, when we compare strings using the if
statement and the ==
and !=
operators, we are evaluating the memory references of the string objects rather than their actual content.
The ==
operator checks if two strings are the same (identical memory objects), while the !=
operator checks if they are different (distinct objects). It’s important to note that we are comparing the memory addresses or references of the string objects, not their actual content.
Code:
public class StringComparisonExample {
public static void main(String[] args) {
String str1 = "hello";
String str2 = "hello";
String str3 = new String("hello");
// Using == for equality comparison
if (str1 == str2) {
System.out.println("Strings are equal using == operator.");
} else {
System.out.println("Strings are not equal using == operator.");
}
// Using != for inequality comparison
if (str1 != str2) {
System.out.println("Strings are not equal using != operator.");
} else {
System.out.println("Strings are equal using != operator.");
}
// Comparing different objects in memory
if (str1 == str3) {
System.out.println("Strings are equal.");
} else {
System.out.println("Strings are not equal.");
}
}
}
Output:
Strings are equal using == operator.
Strings are not equal using != operator.
Strings are not equal.
Let’s explain the output.
The first if
statement (str1 == str2
) compares two string literals, and since string literals are interned in Java, both str1
and str2
refer to the same object in memory. Therefore, the condition is true, and the first message is printed.
The second if
statement (str1 != str2
) checks if str1
and str2
are not the same object in memory. Since they refer to the same object (as explained in the first point), the condition is false, and the second message is printed.
The third if
statement (str1 == str3
) compares str1
(a string literal) with str3
(a string created with new String("hello")
), and although their contents are the same, they are different objects in memory. Therefore, the condition is false, and the third message is printed.
For content-based comparisons, where we want to check if the actual character sequences are the same, it is more appropriate to use the equals()
method.
Compare String With the Java if
Statement Using the equals()
Function
Through the equals()
function, we can compare the content of the two strings. It will see if the content is similar.
It’s case-sensitive, but we can also ignore the case sensitivity by using the equalsIgnoreCase()
function instead.
Basic Syntax:
str1.equals(str2)
In the syntax, the parameter is str2
. The equals()
method is called on the string referenced by str1
, and the content of this string is compared to the content of the string passed as an argument to the equals()
method, which is str2
in this case.
The equals()
method is comparing the content of str1
to the content of str2
to determine if they are equal. Let’s give an example.
Code 1:
public class StringComparisonExample {
public static void main(String[] args) {
String str1 = "hello";
String str2 = "hello";
if (str1.equals(str2)) {
System.out.println("Strings are equal.");
} else {
System.out.println("Strings are not equal.");
}
}
}
Output:
Strings are equal.
In the code above, we initialize two string variables, str1
and str2
, both containing the value "hello"
. Using the equals()
method within the if
statement, we compare the content of these strings.
Since the content is identical, the condition evaluates to true, and the program prints Strings are equal
.
In this next example, we will use the equalsIgnoreCase()
method in Java to compare two strings while ignoring their case.
Code 2:
public class StringComparisonExample {
public static void main(String[] args) {
String str1 = "Hello";
String str2 = "hello";
if (str1.equalsIgnoreCase(str2)) {
System.out.println("Strings are equal.");
} else {
System.out.println("Strings are not equal.");
}
}
}
Output:
Strings are equal.
In this code, we declare two string variables, str1
and str2
, with different cases: "Hello"
and "hello"
. Using the equalsIgnoreCase()
method within the if
statement, we compare these strings while disregarding case differences.
Despite the difference in letter case, the method considers them equal, and thus, the condition evaluates to true and prints Strings are equal.
, demonstrating a case-insensitive comparison of the string content.
Compare String With the Java if
Statement Using the compareTo()
Function
The compareTo()
method in Java is a method provided by the Comparable
interface, which is implemented by the String
class and many other classes in the Java standard library. It is used to compare the lexicographical (dictionary) order of two strings.
The compareTo()
method returns an integer: 0
if the strings are equal, a negative value if the calling string precedes the argument string, and a positive value if it follows.
Basic Syntax:
str1.compareTo(str2)
In the syntax str1.compareTo(str2)
, str2
is the parameter. This expression compares the lexicographical order of the string referenced by str1
to that of str2
.
In the following example, three strings—str1
, str2
, and str3
—are compared using compareTo()
with the if
statement.
Code:
public class StringComparisonExample {
public static void main(String[] args) {
String str1 = "hello";
String str2 = "hello";
String str3 = "world";
// Comparing str1 and str2 using compareTo()
int result1 = str1.compareTo(str2);
if (result1 == 0) {
System.out.println("str1 is the same as str2");
} else if (result1 < 0) {
System.out.println("str1 comes before str2");
} else {
System.out.println("str1 comes after str2");
}
// Comparing str1 and str3 using compareTo()
int result2 = str1.compareTo(str3);
if (result2 == 0) {
System.out.println("str1 is the same as str3");
} else if (result2 < 0) {
System.out.println("str1 comes before str3");
} else {
System.out.println("str1 comes after str3");
}
}
}
Output:
str1 is the same as str2
str1 comes before str3
In this code, we compare three string variables: str1
, str2
, and str3
. Using the compareTo()
method, we assess the lexicographical order of str1
and str2
, and the result, stored in result1
, is then evaluated with if-else
statements.
Since str1
and str2
are equal, the program prints str1 is the same as str2
. Next, we compare str1
with str3
using compareTo()
, storing the result in result2
.
Given that str1
precedes str3
lexicographically, the output is str1 comes before str3
.
Conclusion
In conclusion, our guide on string comparison in Java using the if
statement has covered diverse methods and operators. We began with the ==
and !=
operators, showcasing their evaluation of memory references.
Moving on, we explored content-based comparisons with the equals()
method and its case-insensitive counterpart, equalsIgnoreCase()
. Lastly, we delved into lexicographical comparisons using the compareTo()
method.
Our examples and explanations aim to provide a comprehensive understanding of these techniques for effective string comparison in Java.
Haider specializes in technical writing. He has a solid background in computer science that allows him to create engaging, original, and compelling technical tutorials. In his free time, he enjoys adding new skills to his repertoire and watching Netflix.
LinkedIn