Java の if 文で文字列を比較する
-
Java の
if
文で==
演算子を使って文字列を比較する -
equal()
関数を使用して文字列を Javaif
ステートメントと比較する -
compareTo()
関数を使用して文字列を Javaif
ステートメントと比較する
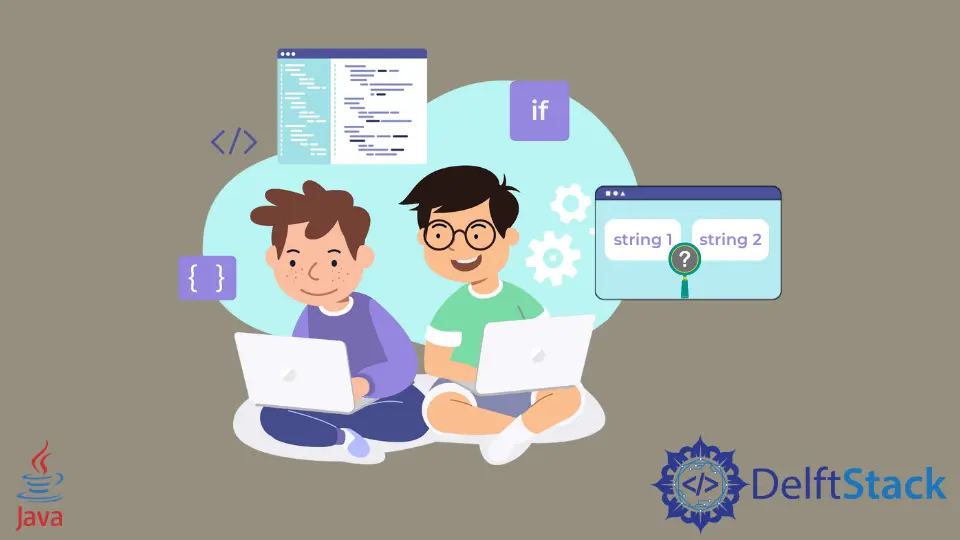
このガイドでは、Java での if ステートメントの文字列比較について説明します。2つの文字列を比較するには、一般に 3つの方法があります。これらの操作の基本を理解し、比較しているもの(コンテンツ、参照、または文字列の違い)を見つける必要があります。これを詳しく見てみましょう。
Java の if
文で ==
演算子を使って文字列を比較する
==
演算子を使用して if ステートメントで 2つの文字列を比較すると、それらの文字列の参照番号が比較されますが、コンテンツの比較と同じように機能することがわかります。同じ内容の文字列が 2つある場合は、同じものとして表示されます。どうして?Java のコンパイラは、同じ内容の 2つの文字列を同じメモリに格納するのに十分成熟しているためです。
equal()
関数を使用して文字列を Java if
ステートメントと比較する
equal()
関数を使用して、2つの文字列の内容を比較できます。内容が類似しているかどうかを確認します。大文字と小文字が区別されますが、代わりに equalsIgnoreCase()
関数を使用して大文字と小文字を区別することもできます。
compareTo()
関数を使用して文字列を Java if
ステートメントと比較する
この関数では、2つの文字列の違いを取得します。各文字の Unicode 値に基づいて辞書式に比較します。両方の文字列が等しい場合は 0
値を取得し、文字列が他の文字列よりも小さい場合は 0
値未満を取得します。その逆も同様です。
次の自明のコードを見てください。
public class Main {
public static void main(String[] args) {
String str1 = "jeff";
String str2 = "jeff";
String str3 = new String("jeff"); // to declare
String str10 = new String("jeff");
System.out.println("-----------------Using == Operator ----------------");
// using == opreater use for Refrence Comapring instead of content comparison.
if (str1
== str2) { // equal and if Conditon True because both have same Refrence Memory address.
System.out.println("Str1 And Str2 Equal");
}
if (str1
== str3) { // Not Equal If Condition False Because == opreater compares objects refrence.
System.out.println("Str1 and Str3 are equals");
}
if (str10
== str3) { // Not Equal If Condition False Because == opreater compares objects refrence.
System.out.println("Str10 and Str3 are equals");
}
System.out.println("-----------------Using .equal Method----------------");
// Using .equals Method. for String Content Comparison.
if (str1.equals(str2)) { // equal and if Conditon True because both have same string
System.out.println("Str1 And Str2 Equal");
}
if (str1.equals(str3)) { // Equal If Condition true String have same Content.
System.out.println("Str1 and Str3 are equals");
}
// compare two strings diffrence
System.out.println("-----------------Using Compare Method----------------");
// first string.toCompare(String2)
System.out.println(str1.compareTo(str2));
}
}
出力:
Output:
-----------------Using == Operator ----------------
Str1 And Str2 Equal
-----------------Using .equal Method----------------
Str1 And Str2 Equal
Str1 and Str3 are equals
-----------------Using Compare Method----------------
0
Haider specializes in technical writing. He has a solid background in computer science that allows him to create engaging, original, and compelling technical tutorials. In his free time, he enjoys adding new skills to his repertoire and watching Netflix.
LinkedIn