How to Get the First Character of a String in Java
-
Get the First Character Using the
charAt()
Method in Java -
Get the First Character Using the
charAt()
Method With No Exception in Java -
Get the First Character Using the String
substring()
Method in Java -
Get the First Character Using the
substring()
Method in Java -
Get the First Character Using the
toCharArray()
Method in Java - Summary
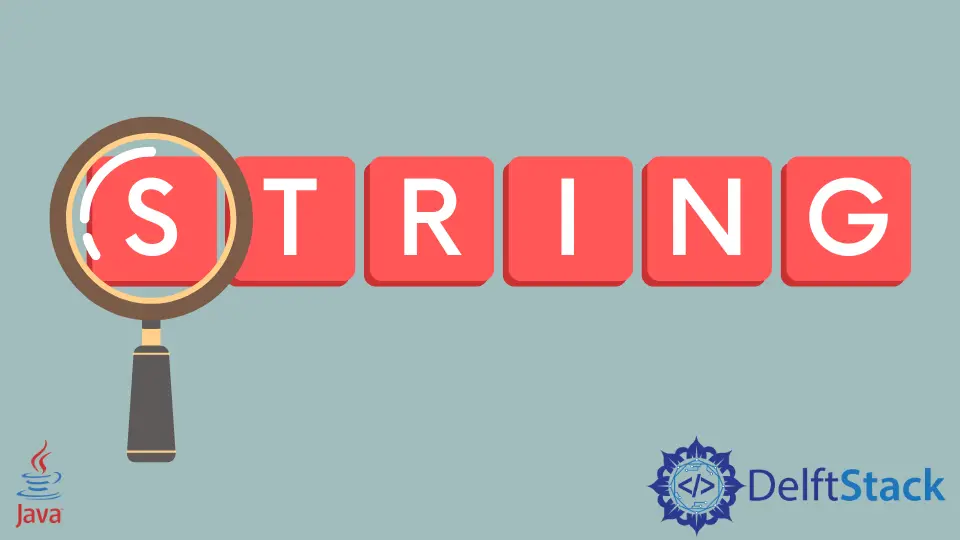
This tutorial introduces how to get the first character of a string in Java. We also listed some example codes to help you understand the topic.
A string is simply a collection or sequence of characters in Java. Strings can be used for various purposes, and we may need to access their different characters to manipulate them.
For example, if we have a string array of first names and we want to make sure that the first character of each name is capitalized, we need to access the first character of each string.
Let’s learn the different ways of fetching the first character from a string.
Get the First Character Using the charAt()
Method in Java
The charAt()
method takes an integer index value as a parameter and returns the character present at that index. The String
class method and its return type are a char
value.
The program below shows how to use this method to fetch the first character of a string. We know that strings follow zero-based indexing, and the first character is always present at index 0. See the example below.
public class Main {
public static void main(String[] args) {
String s = "demo";
char firstCharacter = s.charAt(0);
System.out.println("The String is: " + s);
System.out.println("The first character of the String is: " + firstCharacter);
}
}
Output:
The String is: demo
The first character of the String is: d
Note that the charAt()
method throws an IndexOutOfBoundsException
with this condition: if the index parameter is greater than or equal to the string’s length or if it is negative. This exception is also thrown if we try to get the first character of an empty string.
The following code will throw this error as the string is empty.
public class Main {
public static void main(String[] args) {
String s = "";
char firstCharacter = s.charAt(0);
System.out.println("The first character of the String is: " + firstCharacter);
}
}
Output:
Exception in thread "main" java.lang.StringIndexOutOfBoundsException: String index out of range: 0
at java.base/java.lang.StringLatin1.charAt(StringLatin1.java:48)
at java.base/java.lang.String.charAt(String.java:712)
at Main.main(Main.java:6)
Get the First Character Using the charAt()
Method With No Exception in Java
Let’s write a method to print the string’s first character using the charAt()
method. This process will also check if the string is empty to avoid the IndexOutOfBoundsException
.
public class Main {
public static Character getFirstCharacter(String s) {
if (s == null || s.length() == 0)
return null;
else
return (Character) s.charAt(0);
}
public static void main(String[] args) {
String s = "demonstration";
System.out.println("The string is: " + s);
System.out.print("The first character of string is: " + getFirstCharacter(s));
}
}
Output:
The string is: demonstration
The first character of string is: d
Get the First Character Using the String substring()
Method in Java
The string substring()
method can be used to extract a substring from another string. The method signature is shown below; it has one overloaded version.
public String substring(int startIdx) public String substring(int startIdx, int endIdx)
If we just pass the starting index, it will fetch a substring starting from the startIdx
and include all the remaining characters. If we pass the endIdx
, it will fetch the substring starting at startIdx
and ending at endIdx
(exclusive range).
See the example below.
public class Main {
public static void main(String[] args) {
String s = "demonstration";
System.out.println("The string is: " + s);
System.out.println("Substring starting from index 3: " + s.substring(3));
System.out.println(
"Substring starting from index 2 and ending at index 5: " + s.substring(2, 5));
}
}
Output:
The string is: demonstration
Substring starting from index 3: onstration
Substring starting from index 2 and ending at index 5: mon
Get the First Character Using the substring()
Method in Java
We can pass the starting index as 0
and the ending index as 1
to fetch the string’s first character. Note that the return type of this method is a String
, so even the single character will be returned as a String
. It will throw a StringIndexOutOfBoundsException
if the string is empty. We need to check for this condition before using the substring()
method.
public class Main {
public static String getFirstCharacter(String s) {
if (s == null || s.length() == 0)
return null;
else
return s.substring(0, 1);
}
public static void main(String[] args) {
String s = "demonstration";
System.out.println("The string is: " + s);
System.out.print("The first character of string is: " + getFirstCharacter(s));
}
}
Output:
The string is: demonstration
The first character of string is: d
Get the First Character Using the toCharArray()
Method in Java
We know that we can access the elements of an array by using their index value. If we can convert our string to an array of the char
data type, we can easily fetch any element by using its index.
We can get the first character through index 0
. We will use the toCharArray()
method to convert the string into a character array.
An example to get the first character of the array using toCharArray()
is shown below.
public class Main {
public static Character getFirstCharacter(String s) {
if (s == null || s.length() == 0)
return null;
else {
char[] charArr = s.toCharArray();
return charArr[0];
}
}
public static void main(String[] args) {
String s = "demonstration";
System.out.println("The string is: " + s);
System.out.print("The first character of string is: " + getFirstCharacter(s));
}
}
Output:
The string is: demonstration
The first character of string is: d
Summary
Here, we have learned how to access the first character of a string. We can use the charAt()
method and pass the index parameter as 0
to fetch the string’s first character.
We can also use the substring()
method with the start index as 0
and the end index as 1
to get the first character as a substring. The toCharArray()
method can also be used first to convert the string to a character array and then fetch the first character using the array index.