获取 Java 中字符串的第一个字符
-
在 Java 中使用
charAt()
方法获取第一个字符 -
在 Java 中使用
charAt()
方法无异常地获取第一个字符 -
在 Java 中使用字符串
substring()
方法获取第一个字符 -
在 Java 中使用
substring()
方法获取第一个字符 -
在 Java 中使用
toCharArray()
方法获取第一个字符 - 总结
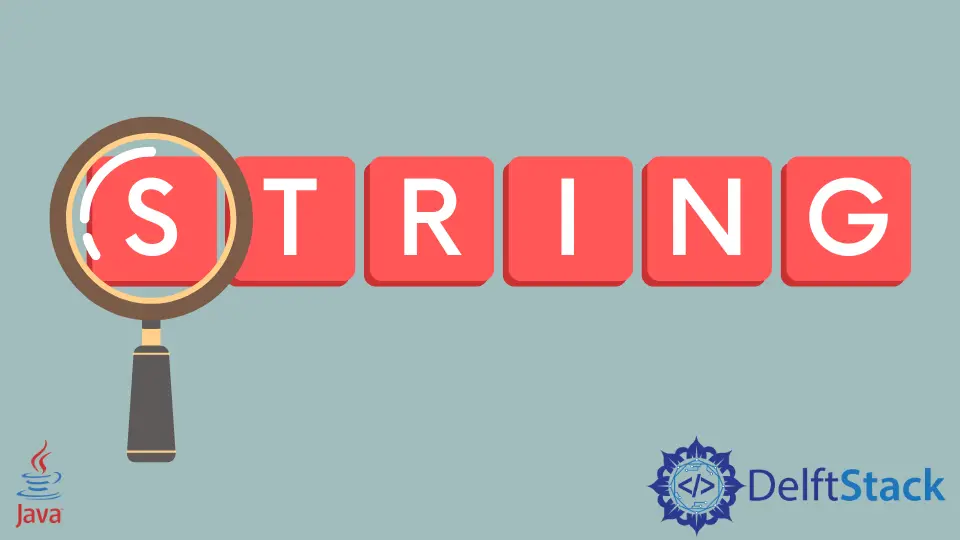
本教程介绍如何在 Java 中获取字符串的第一个字符。我们还列出了一些示例代码以帮助你理解该主题。
在 Java 中,字符串只是字符的集合或序列。字符串可以用于各种目的,我们可能需要访问它们不同的字符来操作它们。
例如,如果我们有一个名字的字符串数组,并且我们想确保每个名字的第一个字符都是大写的,我们需要访问每个字符串的第一个字符。
让我们学习从字符串中获取第一个字符的不同方法。
在 Java 中使用 charAt()
方法获取第一个字符
charAt()
方法将整数索引值作为参数并返回该索引处的字符。String
类方法及其返回类型是一个 char
值。
下面的程序显示了如何使用此方法获取字符串的第一个字符。我们知道字符串遵循从零开始的索引,第一个字符总是出现在索引 0 处。请参见下面的示例。
public class Main {
public static void main(String[] args) {
String s = "demo";
char firstCharacter = s.charAt(0);
System.out.println("The String is: " + s);
System.out.println("The first character of the String is: " + firstCharacter);
}
}
输出:
The String is: demo
The first character of the String is: d
请注意,charAt()
方法会在以下条件下抛出 IndexOutOfBoundsException
:如果索引参数大于或等于字符串的长度,或者它是负数。如果我们尝试获取空字符串的第一个字符,也会抛出此异常。
由于字符串为空,以下代码将引发此错误。
public class Main {
public static void main(String[] args) {
String s = "";
char firstCharacter = s.charAt(0);
System.out.println("The first character of the String is: " + firstCharacter);
}
}
输出:
Exception in thread "main" java.lang.StringIndexOutOfBoundsException: String index out of range: 0
at java.base/java.lang.StringLatin1.charAt(StringLatin1.java:48)
at java.base/java.lang.String.charAt(String.java:712)
at Main.main(Main.java:6)
在 Java 中使用 charAt()
方法无异常地获取第一个字符
让我们编写一个方法来使用 charAt()
方法打印字符串的第一个字符。此过程还将检查字符串是否为空以避免 IndexOutOfBoundsException
。
public class Main {
public static Character getFirstCharacter(String s) {
if (s == null || s.length() == 0)
return null;
else
return (Character) s.charAt(0);
}
public static void main(String[] args) {
String s = "demonstration";
System.out.println("The string is: " + s);
System.out.print("The first character of string is: " + getFirstCharacter(s));
}
}
输出:
The string is: demonstration
The first character of string is: d
在 Java 中使用字符串 substring()
方法获取第一个字符
字符串 substring()
方法可用于从另一个字符串中提取子字符串。方法签名如下所示;它有一个重载版本。
public String substring(int startIdx) public String substring(int startIdx, int endIdx)
如果我们只传递起始索引,它将获取一个从 startIdx
开始的子字符串,并包含所有剩余的字符。如果我们传递 endIdx
,它将获取从 startIdx
开始到 endIdx
(不包括范围)结束的子字符串。
请参考下面的示例。
public class Main {
public static void main(String[] args) {
String s = "demonstration";
System.out.println("The string is: " + s);
System.out.println("Substring starting from index 3: " + s.substring(3));
System.out.println(
"Substring starting from index 2 and ending at index 5: " + s.substring(2, 5));
}
}
输出:
The string is: demonstration
Substring starting from index 3: onstration
Substring starting from index 2 and ending at index 5: mon
在 Java 中使用 substring()
方法获取第一个字符
我们可以将起始索引设为 0
,将结束索引设为 1
以获取字符串的第一个字符。请注意,此方法的返回类型是 String
,因此即使是单个字符也会作为 String
返回。如果字符串为空,它将抛出 StringIndexOutOfBoundsException
。我们需要在使用 substring()
方法之前检查这种情况。
public class Main {
public static String getFirstCharacter(String s) {
if (s == null || s.length() == 0)
return null;
else
return s.substring(0, 1);
}
public static void main(String[] args) {
String s = "demonstration";
System.out.println("The string is: " + s);
System.out.print("The first character of string is: " + getFirstCharacter(s));
}
}
输出:
The string is: demonstration
The first character of string is: d
在 Java 中使用 toCharArray()
方法获取第一个字符
我们知道我们可以通过使用它们的索引值来访问数组的元素。如果我们可以将字符串转换为 char
数据类型的数组,我们就可以使用其索引轻松获取任何元素。
我们可以通过索引 0
获取第一个字符。我们将使用 toCharArray()
方法将字符串转换为字符数组。
下面显示了使用 toCharArray()
获取数组第一个字符的示例。
public class Main {
public static Character getFirstCharacter(String s) {
if (s == null || s.length() == 0)
return null;
else {
char[] charArr = s.toCharArray();
return charArr[0];
}
}
public static void main(String[] args) {
String s = "demonstration";
System.out.println("The string is: " + s);
System.out.print("The first character of string is: " + getFirstCharacter(s));
}
}
输出:
The string is: demonstration
The first character of string is: d
总结
在这里,我们学习了如何访问字符串的第一个字符。我们可以使用 charAt()
方法并将索引参数作为 0
传递来获取字符串的第一个字符。
我们还可以使用 substring()
方法,开始索引为 0
,结束索引为 1
来获取第一个字符作为子字符串。也可以先使用 toCharArray()
方法将字符串转换为字符数组,然后使用数组索引获取第一个字符。