獲取 Java 中字串的第一個字元
-
在 Java 中使用
charAt()
方法獲取第一個字元 -
在 Java 中使用
charAt()
方法無異常地獲取第一個字元 -
在 Java 中使用字串
substring()
方法獲取第一個字元 -
在 Java 中使用
substring()
方法獲取第一個字元 -
在 Java 中使用
toCharArray()
方法獲取第一個字元 - 總結
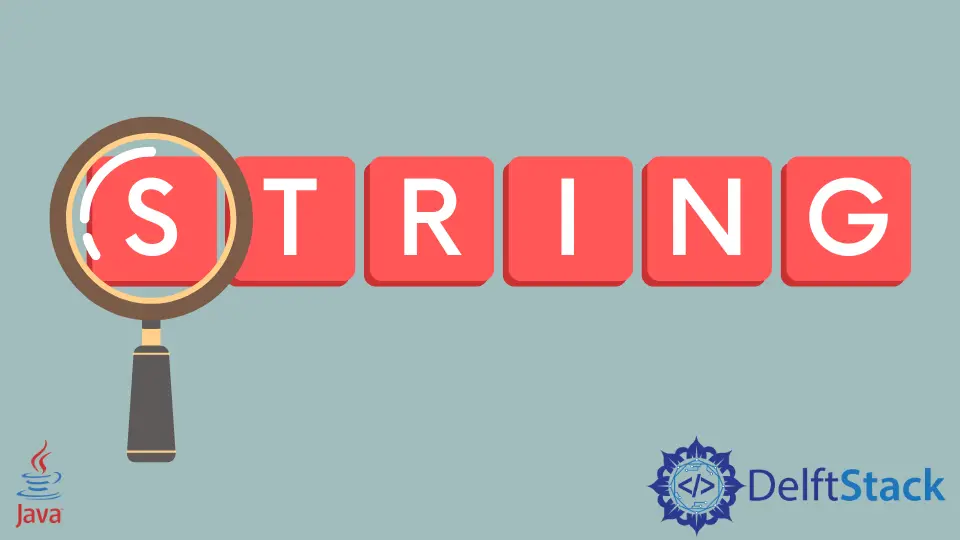
本教程介紹如何在 Java 中獲取字串的第一個字元。我們還列出了一些示例程式碼以幫助你理解該主題。
在 Java 中,字串只是字元的集合或序列。字串可以用於各種目的,我們可能需要訪問它們不同的字元來操作它們。
例如,如果我們有一個名字的字串陣列,並且我們想確保每個名字的第一個字元都是大寫的,我們需要訪問每個字串的第一個字元。
讓我們學習從字串中獲取第一個字元的不同方法。
在 Java 中使用 charAt()
方法獲取第一個字元
charAt()
方法將整數索引值作為引數並返回該索引處的字元。String
類方法及其返回型別是一個 char
值。
下面的程式顯示瞭如何使用此方法獲取字串的第一個字元。我們知道字串遵循從零開始的索引,第一個字元總是出現在索引 0 處。請參見下面的示例。
public class Main {
public static void main(String[] args) {
String s = "demo";
char firstCharacter = s.charAt(0);
System.out.println("The String is: " + s);
System.out.println("The first character of the String is: " + firstCharacter);
}
}
輸出:
The String is: demo
The first character of the String is: d
請注意,charAt()
方法會在以下條件下丟擲 IndexOutOfBoundsException
:如果索引引數大於或等於字串的長度,或者它是負數。如果我們嘗試獲取空字串的第一個字元,也會丟擲此異常。
由於字串為空,以下程式碼將引發此錯誤。
public class Main {
public static void main(String[] args) {
String s = "";
char firstCharacter = s.charAt(0);
System.out.println("The first character of the String is: " + firstCharacter);
}
}
輸出:
Exception in thread "main" java.lang.StringIndexOutOfBoundsException: String index out of range: 0
at java.base/java.lang.StringLatin1.charAt(StringLatin1.java:48)
at java.base/java.lang.String.charAt(String.java:712)
at Main.main(Main.java:6)
在 Java 中使用 charAt()
方法無異常地獲取第一個字元
讓我們編寫一個方法來使用 charAt()
方法列印字串的第一個字元。此過程還將檢查字串是否為空以避免 IndexOutOfBoundsException
。
public class Main {
public static Character getFirstCharacter(String s) {
if (s == null || s.length() == 0)
return null;
else
return (Character) s.charAt(0);
}
public static void main(String[] args) {
String s = "demonstration";
System.out.println("The string is: " + s);
System.out.print("The first character of string is: " + getFirstCharacter(s));
}
}
輸出:
The string is: demonstration
The first character of string is: d
在 Java 中使用字串 substring()
方法獲取第一個字元
字串 substring()
方法可用於從另一個字串中提取子字串。方法簽名如下所示;它有一個過載版本。
public String substring(int startIdx) public String substring(int startIdx, int endIdx)
如果我們只傳遞起始索引,它將獲取一個從 startIdx
開始的子字串,幷包含所有剩餘的字元。如果我們傳遞 endIdx
,它將獲取從 startIdx
開始到 endIdx
(不包括範圍)結束的子字串。
請參考下面的示例。
public class Main {
public static void main(String[] args) {
String s = "demonstration";
System.out.println("The string is: " + s);
System.out.println("Substring starting from index 3: " + s.substring(3));
System.out.println(
"Substring starting from index 2 and ending at index 5: " + s.substring(2, 5));
}
}
輸出:
The string is: demonstration
Substring starting from index 3: onstration
Substring starting from index 2 and ending at index 5: mon
在 Java 中使用 substring()
方法獲取第一個字元
我們可以將起始索引設為 0
,將結束索引設為 1
以獲取字串的第一個字元。請注意,此方法的返回型別是 String
,因此即使是單個字元也會作為 String
返回。如果字串為空,它將丟擲 StringIndexOutOfBoundsException
。我們需要在使用 substring()
方法之前檢查這種情況。
public class Main {
public static String getFirstCharacter(String s) {
if (s == null || s.length() == 0)
return null;
else
return s.substring(0, 1);
}
public static void main(String[] args) {
String s = "demonstration";
System.out.println("The string is: " + s);
System.out.print("The first character of string is: " + getFirstCharacter(s));
}
}
輸出:
The string is: demonstration
The first character of string is: d
在 Java 中使用 toCharArray()
方法獲取第一個字元
我們知道我們可以通過使用它們的索引值來訪問陣列的元素。如果我們可以將字串轉換為 char
資料型別的陣列,我們就可以使用其索引輕鬆獲取任何元素。
我們可以通過索引 0
獲取第一個字元。我們將使用 toCharArray()
方法將字串轉換為字元陣列。
下面顯示了使用 toCharArray()
獲取陣列第一個字元的示例。
public class Main {
public static Character getFirstCharacter(String s) {
if (s == null || s.length() == 0)
return null;
else {
char[] charArr = s.toCharArray();
return charArr[0];
}
}
public static void main(String[] args) {
String s = "demonstration";
System.out.println("The string is: " + s);
System.out.print("The first character of string is: " + getFirstCharacter(s));
}
}
輸出:
The string is: demonstration
The first character of string is: d
總結
在這裡,我們學習瞭如何訪問字串的第一個字元。我們可以使用 charAt()
方法並將索引引數作為 0
傳遞來獲取字串的第一個字元。
我們還可以使用 substring()
方法,開始索引為 0
,結束索引為 1
來獲取第一個字元作為子字串。也可以先使用 toCharArray()
方法將字串轉換為字元陣列,然後使用陣列索引獲取第一個字元。