How to Create a Dynamic Array in Java
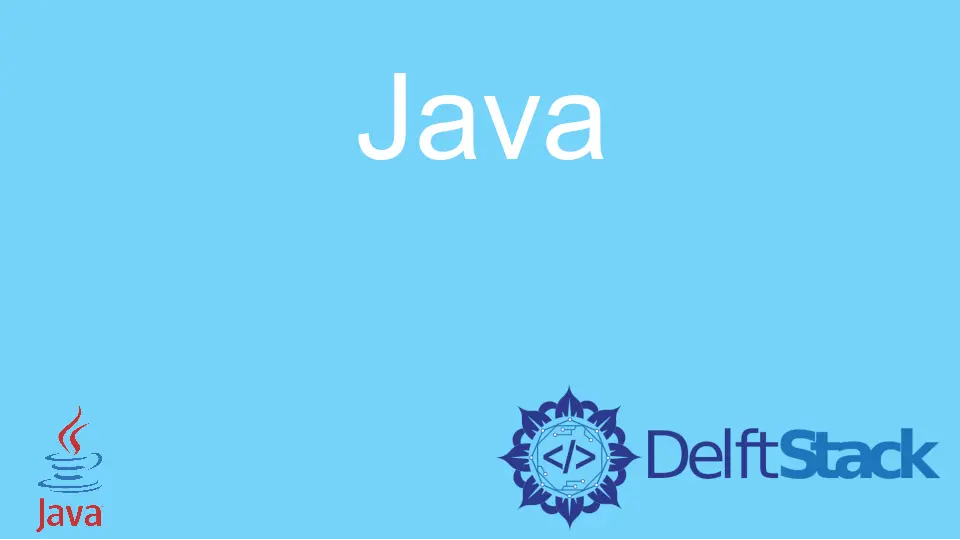
An array is a fixed-size data structure whose size can’t be changed once declared. A dynamic array provides us the facility to create arrays of dynamic sizes. We can increase and decrease these sizes accordingly with, and we’re going to discuss how to make a Java dynamic array in this article.
Create a Dynamic Array using Custom Logic in Java
In the example, we use custom logic that includes methods to add an element at the end of the array or at any index. When the array is full, the array size increases two times. We also remove and shrink the array in the process.
We use two classes to test the dynamic array logic; the first is the DynamicClass
and the second is the DynamicArrayTest
class. In the DynamicArrayTest
, we create an int
type array intArray
and two int
variables named size
and capacity
. The array size is the number of items in it, and an array’s capacity is the total space in it.
We create a constructor of the DynamicArrayTest
class and initialize intArray
with an int
array having the size of 2
. Then, we initialize the size
with 0
and capacity
as 2
. Finally, to add an element at the array’s last position, we create the addElementToArray()
method that accepts an int
element as a parameter. In this function, we first check if the size
and capacity
of the array are the same.
If it’s true, we call the increaseArraySize()
function because the array is full. In the increaseArraySize()
method, we create an empty int
array tempArray
to temporarily store the array elements and we compare the size
and capacity
. We initialize tempArray
with an array and set its size twice the current capacity of the array.
In increaseArraySize()
, we check if the capacity
is more than or equal to 0
. Then we call the System.arraycopy()
method that copies one array’s elements to another array. There, we specify the array to be copied, the starting index to copy, the array where we want to copy the elements, the destination position, and the size of the new array that we want. After all that, we reinitialize intArray
with the elements of tempArray
and increase the size of capacity
.
Now, we create a method to remove the element and name it removeElement()
. In this function, we check the size
of the array if it is greater than zero. Then, we replace the last element of the array with a zero, and we decrease the size by one. Notice that this method removes only the last element of the array.
When the array is full, the capacity of that array is increased, and empty spaces are filled. These empty, unused spaces can increase memory use and memory garbage. To fix this, we remove the empty indexes using the shrinkSize()
function. Here, we create a temporary array and copy all the elements of intArray
in the function, whose size is the same as its elements, and then copy the array elements back to intArray
.
class DynamicArrayTest {
int[] intArray;
int size;
int capacity;
public DynamicArrayTest() {
intArray = new int[2];
size = 0;
capacity = 2;
}
public void addElementToArray(int a) {
if (size == capacity) {
increaseArraySize();
}
intArray[size] = a;
size++;
}
public void increaseArraySize() {
int[] tempArray = null;
if (size == capacity) {
tempArray = new int[capacity * 2];
{
if (capacity >= 0) {
System.arraycopy(intArray, 0, tempArray, 0, capacity);
}
}
}
intArray = tempArray;
capacity = capacity * 2;
}
public void shrinkSize() {
int[] temp;
if (size > 0) {
temp = new int[size];
System.arraycopy(intArray, 0, temp, 0, size);
capacity = size;
intArray = temp;
}
}
public void removeElement() {
if (size > 0) {
intArray[size - 1] = 0;
size--;
}
}
}
public class DynamicArray {
public static void main(String[] args) {
DynamicArrayTest dynamicArrayTest = new DynamicArrayTest();
dynamicArrayTest.addElementToArray(10);
dynamicArrayTest.addElementToArray(20);
dynamicArrayTest.addElementToArray(30);
dynamicArrayTest.addElementToArray(40);
dynamicArrayTest.addElementToArray(50);
System.out.println("items of intArray:");
for (int i = 0; i < dynamicArrayTest.capacity; i++) {
System.out.print(dynamicArrayTest.intArray[i] + " ");
}
System.out.println();
System.out.println("Capacity of the intArray: " + dynamicArrayTest.capacity);
System.out.println("Size of the intArray: " + dynamicArrayTest.size);
dynamicArrayTest.removeElement();
System.out.println("\nItems after removing the last element");
for (int i = 0; i < dynamicArrayTest.capacity; i++) {
System.out.print(dynamicArrayTest.intArray[i] + " ");
}
System.out.println("\nCapacity of the intArray: " + dynamicArrayTest.capacity);
System.out.println("Size of the intArray: " + dynamicArrayTest.size);
dynamicArrayTest.shrinkSize();
System.out.println("\nItems after removing unused space");
for (int i = 0; i < dynamicArrayTest.capacity; i++) {
System.out.print(dynamicArrayTest.intArray[i] + " ");
}
System.out.println("\nCapacity of the intArray: " + dynamicArrayTest.capacity);
System.out.println("Size of the intArray: " + dynamicArrayTest.size);
}
}
Output:
items of intArray:
10 20 30 40 50 0 0 0
Capacity of the intArray: 8
Size of the intArray: 5
Items after removing the last element
10 20 30 40 0 0 0 0
Capacity of the intArray: 8
Size of the intArray: 4
Items after removing unused space
10 20 30
Capacity of the intArray: 3
Size of the intArray: 3
Rupam Saini is an android developer, who also works sometimes as a web developer., He likes to read books and write about various things.
LinkedIn