Java에서 동적 배열 만들기
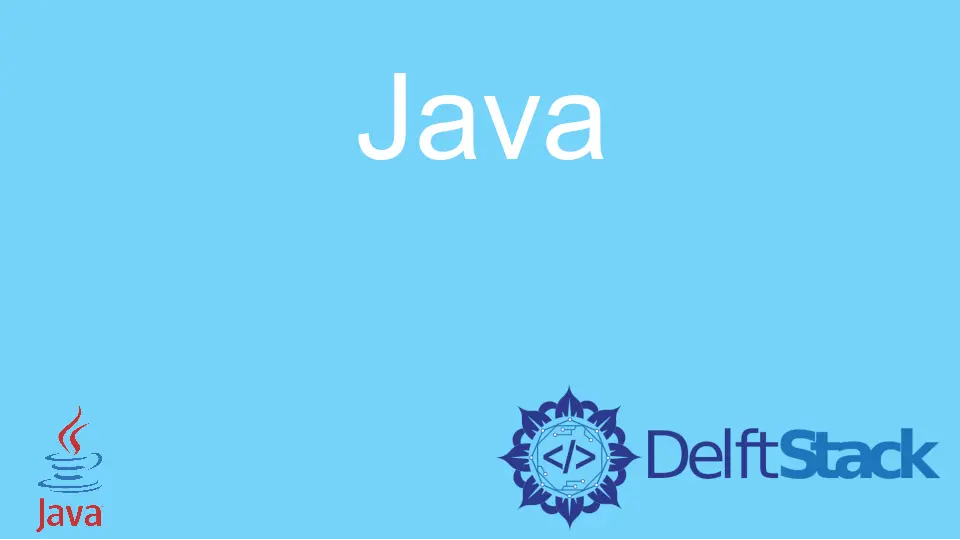
배열은 일단 선언되면 크기를 변경할 수없는 고정 크기 데이터 구조입니다. 동적 배열은 동적 크기의 배열을 생성하는 기능을 제공합니다. 이에 따라 이러한 크기를 늘리거나 줄일 수 있으며이 기사에서는 Java 동적 배열을 만드는 방법에 대해 논의 할 것입니다.
Java에서 사용자 지정 논리를 사용하여 동적 배열 만들기
이 예에서는 배열의 끝이나 인덱스에 요소를 추가하는 메서드를 포함하는 사용자 지정 논리를 사용합니다. 어레이가 가득 차면 어레이 크기가 두 배 증가합니다. 또한 프로세스에서 어레이를 제거하고 축소합니다.
두 개의 클래스를 사용하여 동적 배열 논리를 테스트합니다. 첫 번째는DynamicClass
이고 두 번째는DynamicArrayTest
클래스입니다. DynamicArrayTest
에서int
유형 배열intArray
와size
및capacity
라는 두 개의int
변수를 생성합니다. 어레이 크기는 그 안에있는 항목의 수이고 어레이의 용량은 그 안에있는 총 공간입니다.
DynamicArrayTest
클래스의 생성자를 만들고2
크기의int
배열로intArray
를 초기화합니다. 그런 다음size
를0
으로 초기화하고capacity
를2
로 초기화합니다. 마지막으로 배열의 마지막 위치에 요소를 추가하기 위해int
요소를 매개 변수로 허용하는addElementToArray()
메소드를 작성합니다. 이 함수에서 먼저 배열의size
와capacity
가 동일한 지 확인합니다.
사실이면 배열이 가득 차서increaseArraySize()
함수를 호출합니다. increaseArraySize()
메소드에서 빈int
배열tempArray
를 만들어 배열 요소를 임시로 저장하고size
와capacity
를 비교합니다. 배열로tempArray
를 초기화하고 배열의 현재 용량의 두 배 크기를 설정합니다.
increaseArraySize()
에서capacity
가0
이상인지 확인합니다. 그런 다음 한 배열의 요소를 다른 배열로 복사하는System.arraycopy()
메소드를 호출합니다. 여기에서 복사 할 배열, 복사 할 시작 인덱스, 요소를 복사 할 배열, 대상 위치 및 원하는 새 배열의 크기를 지정합니다. 결국,tempArray
의 요소로intArray
를 다시 초기화하고capacity
의 크기를 늘립니다.
이제 요소를 제거하는 메서드를 만들고 이름을removeElement()
로 지정합니다. 이 함수에서 배열의size
가 0보다 크면 확인합니다. 그런 다음 배열의 마지막 요소를 0으로 바꾸고 크기를 1만큼 줄입니다. 이 메서드는 배열의 마지막 요소 만 제거합니다.
어레이가 가득 차면 해당 어레이의 용량이 증가하고 빈 공간이 채워집니다. 이러한 비어 있고 사용되지 않은 공간은 메모리 사용과 메모리 쓰레기를 증가시킬 수 있습니다. 이 문제를 해결하기 위해shrinkSize()
함수를 사용하여 빈 인덱스를 제거합니다. 여기에서 임시 배열을 만들고 함수의intArray
의 모든 요소를 복사합니다.이 요소의 크기는 해당 요소와 동일한 것입니다. 그런 다음 배열 요소를 다시intArray
에 복사합니다.
class DynamicArrayTest {
int[] intArray;
int size;
int capacity;
public DynamicArrayTest() {
intArray = new int[2];
size = 0;
capacity = 2;
}
public void addElementToArray(int a) {
if (size == capacity) {
increaseArraySize();
}
intArray[size] = a;
size++;
}
public void increaseArraySize() {
int[] tempArray = null;
if (size == capacity) {
tempArray = new int[capacity * 2];
{
if (capacity >= 0) {
System.arraycopy(intArray, 0, tempArray, 0, capacity);
}
}
}
intArray = tempArray;
capacity = capacity * 2;
}
public void shrinkSize() {
int[] temp;
if (size > 0) {
temp = new int[size];
System.arraycopy(intArray, 0, temp, 0, size);
capacity = size;
intArray = temp;
}
}
public void removeElement() {
if (size > 0) {
intArray[size - 1] = 0;
size--;
}
}
}
public class DynamicArray {
public static void main(String[] args) {
DynamicArrayTest dynamicArrayTest = new DynamicArrayTest();
dynamicArrayTest.addElementToArray(10);
dynamicArrayTest.addElementToArray(20);
dynamicArrayTest.addElementToArray(30);
dynamicArrayTest.addElementToArray(40);
dynamicArrayTest.addElementToArray(50);
System.out.println("items of intArray:");
for (int i = 0; i < dynamicArrayTest.capacity; i++) {
System.out.print(dynamicArrayTest.intArray[i] + " ");
}
System.out.println();
System.out.println("Capacity of the intArray: " + dynamicArrayTest.capacity);
System.out.println("Size of the intArray: " + dynamicArrayTest.size);
dynamicArrayTest.removeElement();
System.out.println("\nItems after removing the last element");
for (int i = 0; i < dynamicArrayTest.capacity; i++) {
System.out.print(dynamicArrayTest.intArray[i] + " ");
}
System.out.println("\nCapacity of the intArray: " + dynamicArrayTest.capacity);
System.out.println("Size of the intArray: " + dynamicArrayTest.size);
dynamicArrayTest.shrinkSize();
System.out.println("\nItems after removing unused space");
for (int i = 0; i < dynamicArrayTest.capacity; i++) {
System.out.print(dynamicArrayTest.intArray[i] + " ");
}
System.out.println("\nCapacity of the intArray: " + dynamicArrayTest.capacity);
System.out.println("Size of the intArray: " + dynamicArrayTest.size);
}
}
출력:
items of intArray:
10 20 30 40 50 0 0 0
Capacity of the intArray: 8
Size of the intArray: 5
Items after removing the last element
10 20 30 40 0 0 0 0
Capacity of the intArray: 8
Size of the intArray: 4
Items after removing unused space
10 20 30
Capacity of the intArray: 3
Size of the intArray: 3
Rupam Saini is an android developer, who also works sometimes as a web developer., He likes to read books and write about various things.
LinkedIn