How to Create a Dropdown Menu in Java
-
Create a Dropdown Menu Using
JOptionPane
in Java -
Create a Dropdown Menu Using
JComboBox
in Java - Conclusion
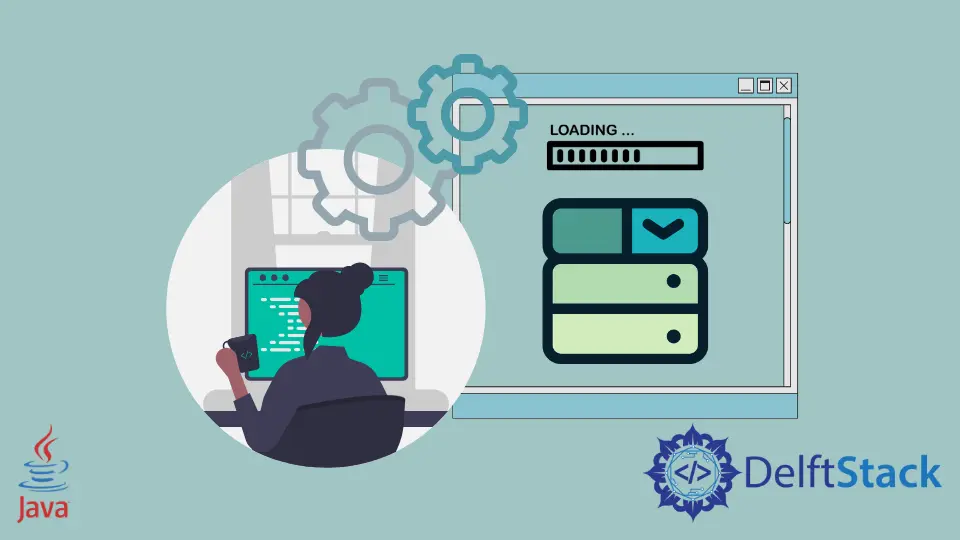
In the realm of Java programming, graphical user interfaces (GUIs) play a pivotal role in delivering a seamless and interactive user experience. Dropdown menus, a fundamental element of GUI design, empower users to make selections from a list of options conveniently.
In this article, we will learn how we can create a dropdown menu in Java using two methods. Both techniques are used to create GUI components, and the dropdown menu is one of them.
Create a Dropdown Menu Using JOptionPane
in Java
The JOptionPane
class is a part of the javax.swing
package, used mostly to create dialog boxes. In the dialog boxes, we can add multiple types of elements and one of them is the dropdown component.
Dropdown menus are a common UI element used to present users with a selection of options in a concise and accessible manner. While there are various ways to implement dropdown menus in Java, this section will focus on utilizing the JOptionPane
method to create a simple and effective dropdown menu.
Let’s explore a practical example that demonstrates how to use JOptionPane
to create a dropdown menu. In this scenario, we’ll design a program that prompts the user to choose their favorite fruit from a list of options.
Code Input:
import javax.swing.*;
public class DropDown {
public static void main(String[] args) {
// Define an array of fruit options
String[] optionsToChoose = {"Apple", "Orange", "Banana", "Pineapple", "None of the listed"};
// Display a dropdown-style dialog using JOptionPane
String getFavFruit = (String) JOptionPane.showInputDialog(
null,
"What fruit do you like the most?",
"Choose Fruit",
JOptionPane.QUESTION_MESSAGE,
null,
optionsToChoose,
optionsToChoose[3]);
// Print the selected fruit to the console
System.out.println("Your chosen fruit: " + getFavFruit);
}
}
In the first line of the code, we import the javax.swing
package, which includes the JOptionPane
class—a powerful tool for creating various types of dialogs, including dropdown menus.
Next, we define an array named optionsToChoose
containing different fruit options, including Apple
, Orange
, Banana
, Pineapple
, and an option for None of the listed
.
We then use the JOptionPane.showInputDialog
method to display a dialog prompting the user to choose their favorite fruit. The method takes various parameters, including the parent component (in this case, null
for a centered dialog), a message, a title, a message type, an array of options, and the default selection (set to optionsToChoose[3]
).
Once the user makes a selection, the chosen fruit is stored in the getFavFruit
variable. We then print this selected fruit to the console.
Code Output:
When you run the code, a dialog box will appear, asking the user to choose their favorite fruit. After making a selection, the chosen fruit will be printed to the console.
Your chosen fruit: Orange
Create a Dropdown Menu Using JComboBox
in Java
In Java, the JComboBox
class, part of the Swing
framework, provides a versatile solution for implementing dropdown menus. This section will guide you through the process of creating a Java program that utilizes the JComboBox
method to present a user-friendly dropdown menu.
Let’s explore a comprehensive example that demonstrates the use of JComboBox
to create a dropdown menu. The program will feature a JFrame
with a JComboBox
containing fruit options, a JButton
for user interaction, and a JLabel
to display the selected fruit.
Code Input:
import java.awt.event.ActionEvent;
import java.awt.event.ActionListener;
import javax.swing.*;
public class JcomboBox {
public static void main(String[] args) {
// Array of fruit options
String[] optionsToChoose = {"Apple", "Orange", "Banana", "Pineapple", "None of the listed"};
// Creating a JFrame
JFrame jFrame = new JFrame();
// Creating a JComboBox and setting its bounds
JComboBox<String> jComboBox = new JComboBox<>(optionsToChoose);
jComboBox.setBounds(80, 50, 140, 20);
// Creating a JButton and setting its bounds
JButton jButton = new JButton("Done");
jButton.setBounds(100, 100, 90, 20);
// Creating a JLabel and setting its bounds
JLabel jLabel = new JLabel();
jLabel.setBounds(90, 150, 400, 100);
// Adding components to the JFrame
jFrame.add(jComboBox);
jFrame.add(jButton);
jFrame.add(jLabel);
// Setting layout to null
jFrame.setLayout(null);
// Setting the size and visibility of the JFrame
jFrame.setSize(350, 250);
jFrame.setVisible(true);
// ActionListener for the JButton
jButton.addActionListener(new ActionListener() {
@Override
public void actionPerformed(ActionEvent e) {
// Getting the selected fruit from JComboBox
String selectedFruit = "You selected " + jComboBox.getItemAt(jComboBox.getSelectedIndex());
// Setting the text of the JLabel
jLabel.setText(selectedFruit);
}
});
}
}
First, we import the necessary packages for event handling and Swing components.
Next, we create a JFrame
and components such as JComboBox
, JButton
, and JLabel
. The bounds are set to determine their positions and sizes within the JFrame
.
Components are added to the JFrame
to display them.
We then set the layout to null to manually position the components. The size of the JFrame
is set to 350 pixels by 250 pixels.
Finally, an ActionListener
is added to the JButton
. When the button is clicked, it retrieves the selected fruit from the JComboBox
and updates the text of the JLabel
accordingly.
Code Output:
When the program is executed, a JFrame
window will appear. It contains a JComboBox
with fruit options, a Done
JButton
, and an initially empty JLabel
.
Upon selecting a fruit and clicking the Done
button, the JLabel
will update to display the selected fruit.
You selected Apple
Conclusion
In this article, we explored two distinct methods to implement dropdown menus, each offering its unique advantages. The first method involved using the JOptionPane
class, a part of the javax.swing
package, primarily used for creating dialog boxes.
The second method utilized the JComboBox
class, a versatile component within the Swing
framework. This approach allows developers to create more customized and interactive dropdown menus embedded within a JFrame
.
Both methods cater to different use cases, with JOptionPane
being suitable for simpler dialog-based interactions and JComboBox
providing more control and flexibility for integrated dropdowns within a graphical interface.
Rupam Saini is an android developer, who also works sometimes as a web developer., He likes to read books and write about various things.
LinkedInRelated Article - Java GUI
- Background Colors in Java
- How to Create Alert Popup in Java
- Button Group in Java
- Best GUI Builders for Java Swing Applications
- How to Create Java Progress Bar Using JProgressBar Class
- How to Create Tic Tac Toe GUI in Java