How to Close a File in Java
-
Close a File Using the
try-with-resources
in Java (7+) -
Use the
close()
Method to Close a File in Java - Conclusion
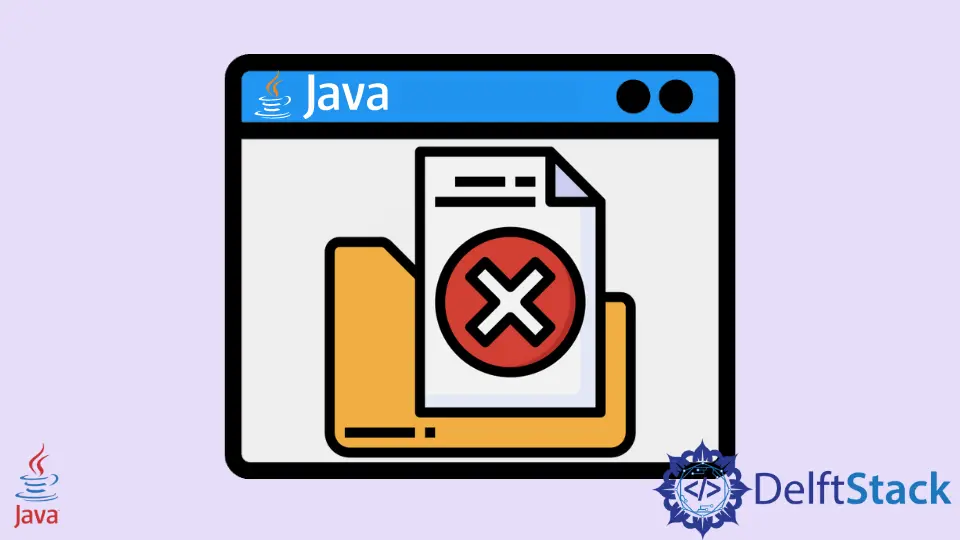
Handling files is a fundamental task in Java programming, and proper resource management is essential. In the past, closing files required manual intervention using finally blocks or the close()
method, which could be error-prone and lead to resource leaks.
However, with the introduction of try-with-resources in Java 7 and the continued use of the close()
method, managing files has become more efficient and less error-prone.
In this article, we will explore how to effectively close files in Java using both try-with-resources
and the close()
method. We’ll discuss the syntax, provide practical examples for each method, and highlight the importance of resource management when working with files.
Close a File Using the try-with-resources
in Java (7+)
Prior to Java 7, developers had to manually close files using finally
blocks, which could be error-prone and lead to resource leaks. However, with the introduction of try-with-resources
in Java 7, handling files became more efficient and less error-prone.
try-with-resources
is a language feature introduced in Java 7 that simplifies resource management. It automatically manages the closing of resources, such as files, at the end of the try
block, whether the block is exited normally or due to an exception. The syntax for using try-with-resources
with files is straightforward:
try (ResourceType resource = new ResourceType()) {
// Use the resource here
} catch (Exception e) {
// Handle exceptions if necessary
}
// Resource is automatically closed when exiting this block
Here’s how to use try-with-resources
to close files:
- Resource Initialization: In the parentheses following the
try
keyword, you declare and initialize the resource (in this case, a file-related class, such asFileInputStream
,FileOutputStream
,BufferedReader
, orBufferedWriter
). The resource should implement theAutoCloseable
interface or its subinterfaces, such asCloseable
. - Resource Usage: Inside the
try
block, you use the resource as needed for reading, writing, or other operations. You don’t need to explicitly close the resource in your code. - Resource Closure: Once the execution leaves the
try
block, whether it’s due to normal completion or an exception, the resource is automatically closed. You don’t need to write afinally
block to ensure closure.
Practical Example
Let’s see how try-with-resources
works with a practical example of reading data from a file:
import java.io.FileInputStream;
import java.io.IOException;
public class FileReadExample {
public static void main(String[] args) {
try (FileInputStream fis = new FileInputStream("example.txt")) {
int data;
while ((data = fis.read()) != -1) {
System.out.print((char) data);
}
} catch (IOException e) {
e.printStackTrace();
}
// The FileInputStream is automatically closed
}
}
In this example, we open a file using FileInputStream
within the try
block and read its contents. Once the block is exited, whether due to normal completion or an exception, the FileInputStream
is automatically closed, releasing system resources.
Exception Handling
try-with-resources
simplifies exception handling as well. If an exception occurs within the try
block, the resource is closed automatically before the exception is propagated up the call stack. You can catch and handle exceptions in the catch
block as needed.
import java.io.FileOutputStream;
import java.io.IOException;
public class FileWriteExample {
public static void main(String[] args) {
try (FileOutputStream fos = new FileOutputStream("output.txt")) {
// Write data to the file
fos.write("Hello, World!".getBytes());
} catch (IOException e) {
e.printStackTrace();
}
// The FileOutputStream is automatically closed
}
}
Use the close()
Method to Close a File in Java
The BufferedWriter
class is utilized in the following program. This class allows you to efficiently write arrays, strings, and characters into a character-output stream.
We also employ the FileWriter
class, designed for writing streams of characters, and the BufferedWriter
class.
A file path is represented by an instance of the File
class file. An abstract pathname is constructed from the specified pathname string.
The BufferedWriter
’s write()
method saves some text to the file. The newLine()
method adds a /n
as a line separator.
The majority of streams don’t need to be closed after being used. When the source is an Input/Output Channel, it is recommended to close the stream.
We should invoke the close()
method before terminating the program or executing any file operations. We might lose some data if we don’t.
As a result, to close the stream and keep the data secure, the close()
method is utilized.
Streams include a method called BaseStream.close()
, which implements Autoclosable
. Almost all stream instances don’t need to be closed because they’re supported by collections, which are arrays that don’t need any additional resource management.
The stream should be closed if the source is an IO channel.
The contents of the file are shown below before conducting the write operation.
import java.io.BufferedWriter;
import java.io.File;
import java.io.FileWriter;
public class CloseFile {
public static void main(String[] args) throws Exception {
File file = new File("/Users/John/Temp/demo1.txt");
if (file.exists()) {
BufferedWriter bufferWriter = new BufferedWriter(new FileWriter(file, true));
bufferWriter.write("New Text");
bufferWriter.newLine();
bufferWriter.close();
}
}
}
After performing the write operation, the file’s contents changed.
Conclusion
In this article, we’ve demonstrated how to use both try-with-resources
and the close()
method to close files, both for reading and writing operations, and how each approach simplifies exception handling.
These methods are considered best practices in modern Java programming for closing files and other resources, ensuring efficient and reliable resource management.
Rupam Saini is an android developer, who also works sometimes as a web developer., He likes to read books and write about various things.
LinkedIn