在 Java 中關閉檔案
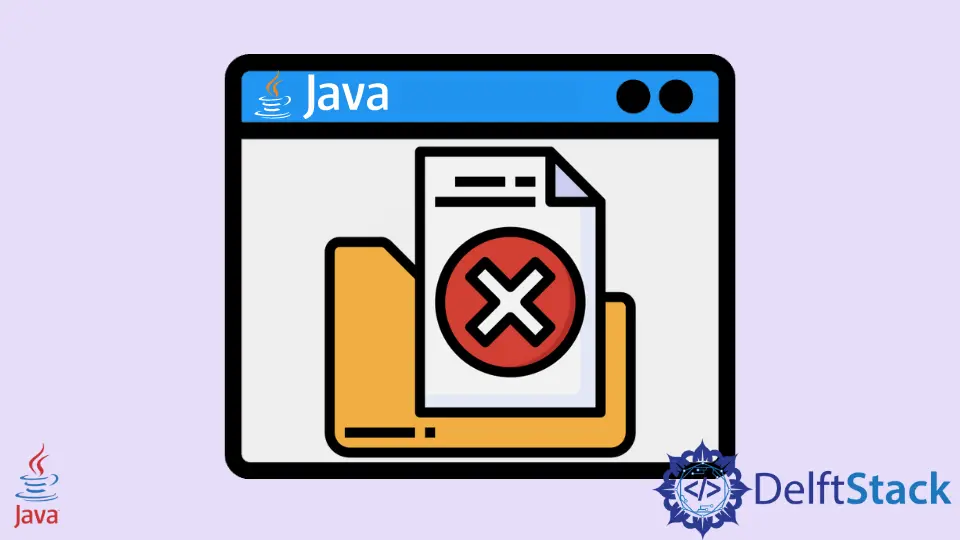
在 Java 程式中,處理檔案是一項基本任務,適當的資源管理是必要的。在過去,關閉檔案需要使用 finally 區塊或 close()
方法的手動介入,可能會出錯並導致資源洩漏。
然而,自從 Java 7 引入了 try-with-resources,並且持續使用 close()
方法後,管理檔案變得更有效率且更不容易出錯。
在本文中,我們將探討如何使用 try-with-resources
和 close()
方法在 Java 中有效地關閉檔案。我們將討論語法,提供每個方法的實際範例,並強調在處理檔案時資源管理的重要性。
使用 try-with-resources
在 Java (7+) 中關閉檔案
在 Java 7 之前,開發人員必須使用 finally
區塊手動關閉檔案,可能會出錯並導致資源洩漏。然而,自從 Java 7 引入了 try-with-resources
,處理檔案變得更有效率且更不容易出錯。
try-with-resources
是 Java 7 中引入的一個語言特性,可以簡化資源管理。它會自動管理資源(如檔案)的關閉,在 try
區塊結束時關閉,無論區塊是正常退出還是因異常退出。使用 try-with-resources
與檔案的語法很簡單:
try (ResourceType resource = new ResourceType()) {
// Use the resource here
} catch (Exception e) {
// Handle exceptions if necessary
}
// Resource is automatically closed when exiting this block
以下是使用 try-with-resources
關閉檔案的方式:
- 資源初始化:在
try
關鍵字後的括號中,聲明並初始化資源(在本例中為相關的檔案類別,如FileInputStream
、FileOutputStream
、BufferedReader
或BufferedWriter
)。該資源應該實現AutoCloseable
接口或其子接口,如Closeable
。 - 資源使用:在
try
區塊內部,根據需要使用資源進行讀取、寫入或其他操作。您不需要在程式碼中明確關閉資源。 - 資源關閉:一旦執行離開
try
區塊,無論是正常完成還是異常退出,資源都會自動關閉。您不需要編寫finally
區塊來確保關閉。
實際範例
讓我們藉由一個從檔案讀取資料的實際範例來看看 try-with-resources
如何運作:
import java.io.FileInputStream;
import java.io.IOException;
public class FileReadExample {
public static void main(String[] args) {
try (FileInputStream fis = new FileInputStream("example.txt")) {
int data;
while ((data = fis.read()) != -1) {
System.out.print((char) data);
}
} catch (IOException e) {
e.printStackTrace();
}
// The FileInputStream is automatically closed
}
}
在此範例中,我們在 try
區塊內使用 FileInputStream
開啟一個檔案並讀取其內容。一旦區塊退出,無論是正常完成還是異常退出,FileInputStream
都會自動關閉,釋放系統資源。
異常處理
try-with-resources
也簡化了異常處理。如果在 try
區塊內發生異常,資源會在異常在 Call Stack 往上傳播之前自動關閉。您可以根據需要在 catch
區塊中捕獲並處理異常。
import java.io.FileOutputStream;
import java.io.IOException;
public class FileWriteExample {
public static void main(String[] args) {
try (FileOutputStream fos = new FileOutputStream("output.txt")) {
// Write data to the file
fos.write("Hello, World!".getBytes());
} catch (IOException e) {
e.printStackTrace();
}
// The FileOutputStream is automatically closed
}
}
使用 close()
方法在 Java 中關閉檔案
下面的程式中使用了 BufferedWriter
類別。這個類別可以將陣列、字串和字符有效地寫入字符輸出串流中。
我們還使用了 FileWriter
類和 BufferedWriter
類。
檔案路徑由 File
類的實例表示。指定的檔案名稱構造出一個抽象路徑名。
BufferedWriter
的 write()
方法將一些文字保存到檔案中。newLine()
方法添加 /n
作為換行符號。
多數串流在使用後不需要關閉。當來源是輸入/輸出通道時,建議關閉串流。
在終止程式或執行任何檔案操作之前,我們應該呼叫 close()
方法。如果不這樣做,我們可能會遺失一些資料。
為了關閉串流並保持資料安全,我們使用 close()
方法。
串流包含一個名為 BaseStream.close()
的方法,它實現了 Autoclosable
接口。幾乎所有的串流實例都不需要被關閉,因為它們被集合支援,而集合是不需要任何額外資源管理的陣列。
如果來源是 IO 通道,則應該關閉該串流。
在進行寫入操作之前,以下是檔案的內容。
import java.io.BufferedWriter;
import java.io.File;
import java.io.FileWriter;
public class CloseFile {
public static void main(String[] args) throws Exception {
File file = new File("/Users/John/Temp/demo1.txt");
if (file.exists()) {
BufferedWriter bufferWriter = new BufferedWriter(new FileWriter(file, true));
bufferWriter.write("New Text");
bufferWriter.newLine();
bufferWriter.close();
}
}
}
進行寫入操作後,檔案的內容已經改變。
結論
在本文中,我們展示了如何使用 try-with-resources
和 close()
方法來關閉檔案,包括讀取和寫入操作,以及每種方法如何簡化例外處理。
這些方法被視為現代 Java 程式設計中關閉檔案和其他資源的最佳實踐,確保有效且可靠的資源管理。
Rupam Saini is an android developer, who also works sometimes as a web developer., He likes to read books and write about various things.
LinkedIn