How to Check if an Array Contains Int in Java
-
Check if Array Contains the Specified Value Using the
anyMatch()
Method -
Check if an Array Contains the Specified Value Using the
contains()
Method -
Check if an Array Contains the Specified Value Using the
contains()
Method -
Check if an Array Contains the Specified Value Using the
binarySearch()
Method - Check if an Array Contains the Specified Value Using the Custom Code
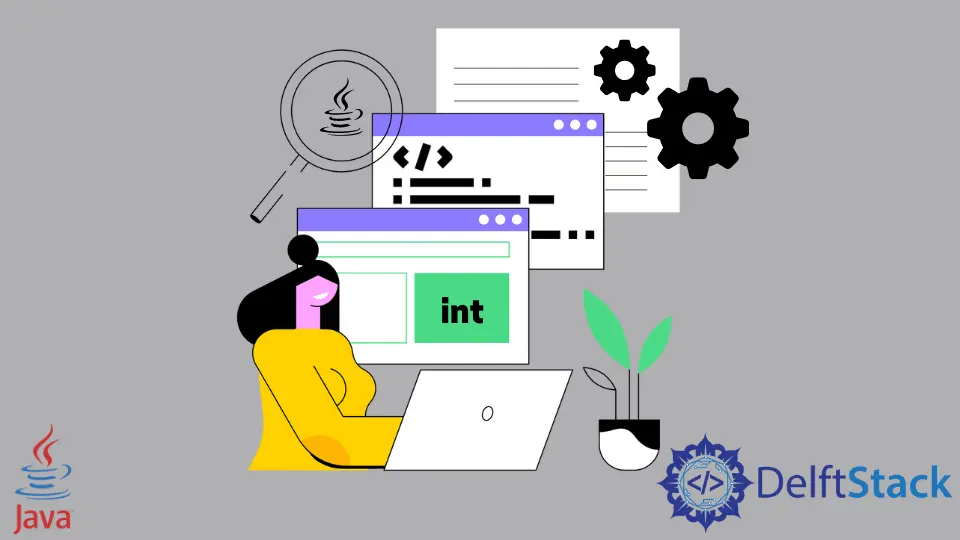
This tutorial introduces how to check if an array contains an int value in Java and lists some example codes to understand the topic.
An array is a container that stores elements of the same data type. For example, an integer array can have only integer type values. Here, we will check if an array contains the given specified value. In this article, we used several built-in methods such as anyMatch()
, contains()
, binarySearch()
, etc that we will find a value in the given array.
Check if Array Contains the Specified Value Using the anyMatch()
Method
We can use the anyMatch()
method to find the specified value in the given array. This method returns a boolean value either true
or false
. It takes a lambda expression as an argument and can be used in Java 8 or higher version.
import java.util.Arrays;
public class SimpleTesting {
public static void main(String[] args) {
int[] arr = {10, 25, 23, 14, 85, 65};
int key = 14;
boolean val = contains(arr, key);
System.out.println("Array contains " + key + "? \n" + val);
}
public static boolean contains(final int[] arr, final int key) {
return Arrays.stream(arr).anyMatch(i -> i == key);
}
}
Output:
Array contains 14?
true
Check if an Array Contains the Specified Value Using the contains()
Method
We can use the contains()
method to find the specified value in the given array. This method returns a boolean value either true
or false
. It takes two arguments; the first is an array, and the second is the value to find. The contains()
method belongs to ArrayUtils
class of Apache commons library. See the example below.
import org.apache.commons.lang3.ArrayUtils;
public class SimpleTesting {
public static void main(String[] args) {
int[] arr = {10, 25, 23, 14, 85, 65};
int key = 14;
boolean val = contains(arr, key);
System.out.println("Array contains " + key + "? \n" + val);
}
public static boolean contains(final int[] arr, final int key) {
return ArrayUtils.contains(arr, key);
}
}
Output:
Array contains 14?
true
Check if an Array Contains the Specified Value Using the contains()
Method
We can convert the array to the list using Arrays.asList()
and then use the list’s contains()
method to find the specified value in the given array. This method returns a boolean value, either true or false. It takes a value as an argument that needs to be found. See the example below.
import java.util.Arrays;
public class SimpleTesting {
public static void main(String[] args) {
int[] arr = {10, 25, 23, 14, 85, 65};
int key = 14;
boolean val = contains(arr, key);
System.out.println("Array contains " + key + "? \n" + val);
}
public static boolean contains(final int[] arr, final int key) {
return Arrays.asList(arr).contains(key);
}
}
Output:
Array contains 14?
true
Check if an Array Contains the Specified Value Using the binarySearch()
Method
We can use the binarySearch()
method to find the specified value in the given array. This method returns a value after matching. It works if the array is sort so, before applying this method sort the array. See the example below.
import java.util.Arrays;
public class SimpleTesting {
public static void main(String[] args) {
int[] arr = {10, 25, 23, 14, 85, 65};
int key = 14;
boolean val = contains(arr, key);
System.out.println("Array contains " + key + "? \n" + val);
}
public static boolean contains(final int[] arr, final int key) {
Arrays.sort(arr);
return Arrays.binarySearch(arr, key) >= 0;
}
}
Output:
Array contains 14?
true
Check if an Array Contains the Specified Value Using the Custom Code
We can use the custom code to find the specified value in the given array. We create a custom method to find the value in the array and return a boolean value, either true or false. This method takes two arguments; the first is an array, and the second is the value that needs to be found. See the example below.
public class SimpleTesting {
public static void main(String[] args) {
int[] arr = {10, 25, 23, 14, 85, 65};
int key = 14;
boolean val = contains(arr, key);
System.out.println("Array contains " + key + "? \n" + val);
}
public static boolean contains(final int[] arr, final int key) {
boolean found = false;
for (int i = 0; i < arr.length; i++) {
if (arr[i] == key) {
found = true;
}
}
return found;
}
}
Output:
Array contains 14?
true