How to Fix Class Is Not Abstract and Does Not Override Error in Java
-
Understanding the Error
Class is not abstract and does not override abstract method
in Java -
Solution 1: Override the
canSpeak()
Method - Solution 2: Declare the Class as Abstract
-
Solution 3: Make
Human
a Class and Extend It in theBaby
Class - Conclusion
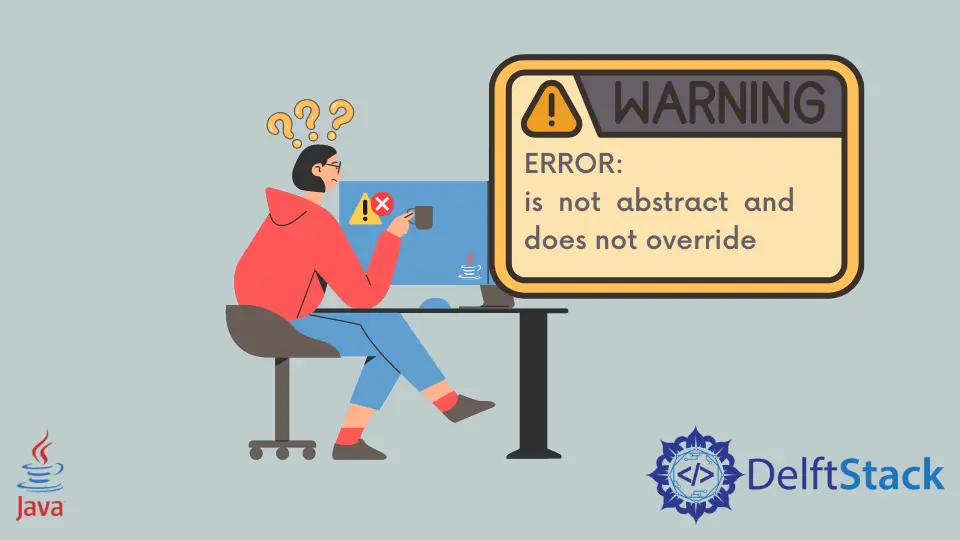
When working with abstract classes and interfaces in Java, it’s not uncommon to encounter the error message: "Class is not abstract and does not override abstract method"
. This error occurs when a class claims to implement an interface or extend an abstract class but fails to provide implementations for all the abstract methods defined in the interface or abstract class.
This article will find solutions to the error Class is not abstract and does not override abstract method
that occurs when we use the concept of abstraction in Java, providing detailed explanations and example codes for each approach.
Understanding the Error Class is not abstract and does not override abstract method
in Java
To understand this error, let’s consider the following scenario:
interface MyInterface {
void myMethod();
}
class MyClass implements MyInterface {
// This class does not provide an implementation for myMethod()
}
In this example, MyClass
claims to implement the MyInterface
interface, which declares the abstract method myMethod()
. However, MyClass
does not provide an implementation for myMethod()
, leading to the error message.
Why Does the Error Class is not abstract and does not override abstract method
Occur in Java?
In the code below, we have two classes and an interface. The class JavaExample
has a main()
method without any body part.
We create a Human
interface with an abstract
method canSpeak()
with boolean
as a return type. We do not specify a body for canSpeak()
because an abstract
method doesn’t have a body.
In the second class, Baby
, we inherit the Human
interface using the implements
keyword. If we use an IDE, there will be an error, and when we run the code, the error will occur, as shown in the output.
public class JavaExample {
public static void main(String[] args) {}
}
class Baby implements Human {}
interface Human {
abstract boolean canSpeak();
}
Output:
JavaExample.java:5: error: Baby is not abstract and does not override abstract method canSpeak() in Human
class Baby implements Human {}
^
1 error
error: compilation failed
If we look at the error Baby is not abstract and does not override abstract method canSpeak() in Human
, we can understand why it occurred. It says that the class Baby
is not abstract, and it doesn’t override the method canSpeak()
of the Human
interface.
This error appears because we have to override the abstract methods to define the body when implementing any class interface with abstract methods.
Solution 1: Override the canSpeak()
Method
To fix the Baby is not abstract and does not override abstract method speak() in Human
error, the first solution is to override the abstract
method canSpeak()
in the Baby
class that implements the Human
interface.
The canSpeak()
function returns false
and in the main()
method we create an object of the Baby
class and call the overridden function canSpeak()
. In the output, we can see that there’s no error and the expected value shows.
public class JavaExample {
public static void main(String[] args) {
Baby baby = new Baby();
System.out.println("Can Speak? " + baby.canSpeak());
}
}
class Baby implements Human {
@Override
public boolean canSpeak() {
return false;
}
}
interface Human {
abstract boolean canSpeak();
}
Output:
Can Speak? false
This code defines a program to determine if a baby can speak. It consists of three components: the JavaExample
class, the Baby
class, and the Human
interface.
In the JavaExample
class, the main
method is the entry point. Inside it, an instance of the Baby
class is created, and the canSpeak
method of the Baby
instance is called.
The result is printed to the console using System.out.println
. The Baby
class implements the Human
interface, which requires it to provide an implementation for the canSpeak
method.
Here, the Baby
class overrides canSpeak
and returns false
, indicating that a baby cannot speak. The Human
interface declares a single abstract method, canSpeak
, specifying that any class implementing it must provide an implementation for this method.
This code demonstrates the use of interfaces to define common behaviors, and in this specific case, it asserts that a Baby
cannot speak according to the canSpeak
method’s implementation in the Baby
class.
Solution 2: Declare the Class as Abstract
If you cannot provide an implementation for all the abstract
methods in an interface or abstract
class within the current class, you can declare the class as abstract
.
This solution involves making the class Baby
an abstract
.
We have the same code here, but Baby
is an abstract
class. It cannot be initialized; we only create an instance of the Baby
class in the main()
method.
public class JavaExample {
public static void main(String[] args) {
Baby baby;
}
}
abstract class Baby implements Human {}
interface Human {
abstract boolean canSpeak();
}
By declaring Baby
as abstract
, it is not mandatory to provide implementations for all the abstract
methods immediately. Subclasses that extend Baby
will be responsible for implementing the required methods.
Solution 3: Make Human
a Class and Extend It in the Baby
Class
The last solution is a different one. Instead of implementing an interface, we can change the interface Human
to a class and extend that class in the Baby
class using the keyword extends
.
We specify the body of canSpeak()
in the Human
class itself, which removes the error.
public class JavaExample {
public static void main(String[] args) {
Baby baby = new Baby();
System.out.println("Can Speak? " + baby.canSpeak());
}
}
class Baby extends Human {}
class Human {
boolean canSpeak() {
return false;
}
}
Output:
Can Speak? false
This code defines a simple program that checks if a Baby
can speak. It consists of three components: the JavaExample
class, the Baby
class, and the Human
class.
In the JavaExample
class, the main
method serves as the entry point of the program. Within it, an instance of the Baby
class is created using the new
keyword.
Subsequently, the canSpeak
method of the Baby
instance is called. The result is then printed to the console using System.out.println
.
The Baby
class extends the Human
class, which means it inherits the canSpeak
method from the Human
class. In this case, the canSpeak
method in the Human
class returns false
, indicating that a human, including a baby, cannot speak in this context.
Overall, this code demonstrates class inheritance in Java, where the Baby
class inherits behavior from the Human
class and checks if a baby can speak based on the canSpeak
method’s implementation in the Human
class.
Conclusion
This article provides comprehensive solutions for resolving the error "Class is not abstract and does not override abstract method"
in Java. This error occurs when a class claims to implement an interface or extend an abstract class but lacks implementations for all the abstract methods defined in the interface or abstract class.
-
The first solution involves overriding the abstract method in the implementing class to provide the required implementation.
-
The second solution suggests declaring the class as
abstract
, allowing subclasses to be responsible for implementing the required methods. -
The third solution transforms the interface into a class and extends it in the implementing class, enabling a default implementation of the method in the base class.
Each solution is accompanied by an example code and detailed explanations to illustrate their application. By applying these solutions, developers can effectively address the specified error, ensuring their Java programs function correctly.
Rupam Saini is an android developer, who also works sometimes as a web developer., He likes to read books and write about various things.
LinkedInRelated Article - Java Error
- How to Fix the Error: Failed to Create the Java Virtual Machine
- How to Fix the Missing Server JVM Error in Java
- How to Fix the 'No Java Virtual Machine Was Found' Error in Eclipse
- How to Fix Javax.Net.SSL.SSLHandShakeException: Remote Host Closed Connection During Handshake
- How to Fix the Error: Failed to Create the Java Virtual Machine
- How to Fix Java.Lang.VerifyError: Bad Type on Operand Stack