How to Initialize an Empty Array in Java
-
Using the
new
Keyword to Declare an Empty Array in Java - Declare and Initialize an Empty Array With a Predefined Size
-
Initialize an Array Without Using the
new
Keyword - Declare an Empty Array Using Array Initializer
- Conclusion
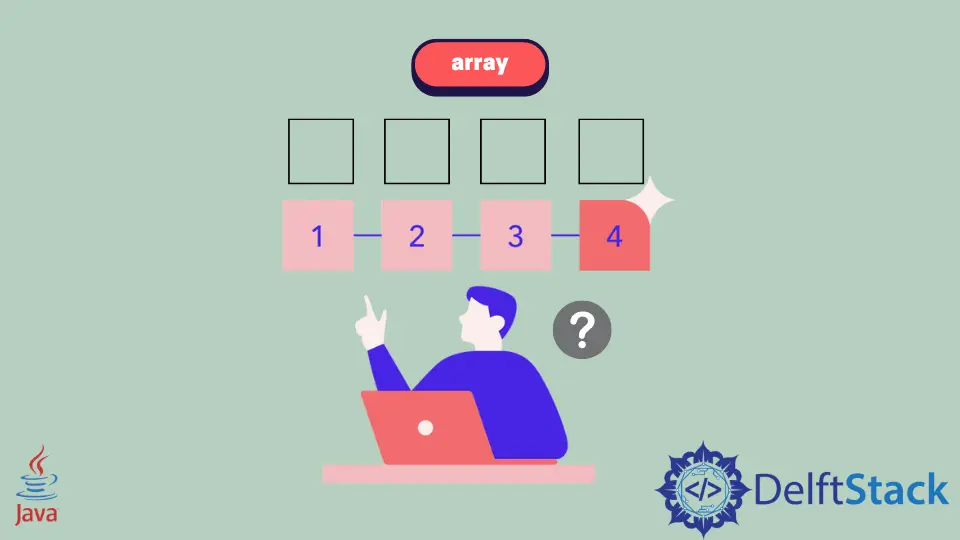
Arrays are like containers that allow you to store and organize multiple values of the same type in a single variable. In Java, initializing an empty array involves using the new
keyword, and there are several ways to achieve this.
Using the new
Keyword to Declare an Empty Array in Java
When we declare an array using the new
keyword, we’re indicating that the array’s size and memory allocation will be determined at runtime.
This dynamic allocation is particularly useful when the size of the array is not known in advance or when it needs to change during the execution of the program.
Syntax of Declaring an Empty Array
To declare an empty array using the new
keyword, you can use one of the following syntax options:
data - type[] array - name = new data - type[size];
// or
data - type array - name[] = new data - type[size];
Here’s a breakdown of each component:
data-type
: This specifies the type of elements the array will hold. It can be any valid data type in Java, such asint
,double
,String
, or a custom object type.array-name
: This is the chosen identifier for your array. It follows the same rules as naming any other variable in Java.size
: This denotes the number of elements the array can hold. It’s an integer value representing the size of the array. This size can be determined dynamically during runtime.
Let’s look at a couple of examples to make this clearer:
public class ArrayInitializationExample {
public static void main(String[] args) {
int[] numbers = new int[5]; // Example 1
String[] names = new String[10]; // Example 2
printIntArray("Initial state of 'numbers' array:", numbers);
printStringArray("\nInitial state of 'names' array:", names);
}
private static void printIntArray(String message, int[] array) {
System.out.println(message);
for (int value : array) {
System.out.print(value + " ");
}
}
private static void printStringArray(String message, String[] array) {
System.out.println(message);
for (String value : array) {
System.out.print(value + " ");
}
}
}
Output:
Initial state of 'numbers' array:
0 0 0 0 0
Initial state of 'names' array:
null null null null null null null null null null
In Example 1
, we declare an array named numbers
that can hold 5 integers. Similarly, in Example 2
, we declare an array named names
capable of storing 10 Strings.
The new
keyword ensures that the necessary memory is allocated for these arrays during runtime.
By using the new
keyword, Java handles the behind-the-scenes work of memory allocation, allowing you to focus on utilizing arrays dynamically in your programs.
Declare and Initialize an Empty Array With a Predefined Size
Now, let’s explore a straightforward example where we declare an empty array with a predefined size and then use a for
loop to initialize its values.
Consider the following Java code:
public class DeclareEmptyArray {
public static void main(String args[]) {
int size = 5;
int array[] = new int[size];
for (int i = 0; i < size; i++) {
array[i] = i + 1;
System.out.println("Value at index " + i + ": " + array[i]);
}
}
}
In this code, first, we create an array named array
capable of holding 5 integers. The new
keyword, along with the specified size, ensures that the necessary memory is allocated for the array.
Then, the for
loop is employed to iterate through each index of the array. For each iteration, the array element at the current index is assigned a value, in this case, i + 1
.
This results in the array being filled with values from 1
to 5
.
The System.out.println
statements are responsible for printing out the values stored in the array at each index. This provides a visual representation of the array’s contents.
Expected Output:
Value at index 0: 1
Value at index 1: 2
Value at index 2: 3
Value at index 3: 4
Value at index 4: 5
In this example, the output showcases the values assigned to each index of the array. This process is foundational for scenarios where you know the size of the array beforehand and want to populate it systematically.
Initialize an Array Without Using the new
Keyword
In Java, there’s an alternative method for initializing arrays that doesn’t involve explicitly using the new
keyword. Instead, you can directly assign values to the array.
Let’s explore this approach through the following example:
import java.util.Arrays;
public class DeclareEmptyArray {
public static void main(String args[]) {
int array[] = {5, 5, 5, 5, 5};
for (int i = 0; i < array.length; i++) {
array[i] = i + 1;
System.out.println("Value updated at index " + i + ": " + array[i]);
}
}
}
Here, we initialize an array named array
with the values {5, 5, 5, 5, 5}
. Unlike the previous examples using new
, here we directly provide the initial values enclosed in curly braces {}
.
The for
loop is then employed to iterate through each index of the array. For each iteration, the array element at the current index is updated with a new value, in this case, i + 1
. This effectively replaces the original values in the array.
The System.out.println
statements print out the updated values at each index. This helps visualize the changes made to the array.
Expected Output:
Value updated at index 0: 1
Value updated at index 1: 2
Value updated at index 2: 3
Value updated at index 3: 4
Value updated at index 4: 5
This method offers a more concise way to initialize an array, especially when you already know the initial values you want. It can be particularly handy when the array elements are known at compile-time and don’t need to be dynamically determined during runtime.
Declare an Empty Array Using Array Initializer
You can also declare an empty array using an array initializer, providing a concise way to start with an array of size 0
. Let’s explore this approach through the following example:
public class DeclareEmptyArray {
public static void main(String args[]) {
int array[] = new int[0]; // Declaring an empty array
// Array initialization and printing can be added here as needed
}
}
As you can see, we first declare an empty array named array
with size 0
using int array[] = new int[0];
. This syntax signals that the array is initially empty, and no memory is allocated for elements.
The comment indicates that array initialization and printing can be added as needed. This step is where you can dynamically add elements to the array based on certain conditions during the execution of your program.
This approach becomes particularly useful when you want to start with an empty array and dynamically add elements to it based on certain conditions.
It provides a flexible foundation for scenarios where the size of the array is unknown at the beginning, allowing you to adapt your data structure as your program progresses.
Consider a situation where you need to read input from a user, and the number of inputs is not known in advance. You can start with an empty array and dynamically add elements as the user provides input.
import java.util.Arrays;
import java.util.Scanner;
public class DynamicArrayExample {
public static void main(String args[]) {
int array[] = new int[0];
Scanner scanner = new Scanner(System.in);
System.out.print("Enter a number (or type 'done' to finish): ");
String input = scanner.next();
while (!input.equals("done")) {
int number = Integer.parseInt(input);
int newArray[] = Arrays.copyOf(array, array.length + 1);
newArray[array.length] = number;
array = newArray;
System.out.print("Enter another number (or type 'done' to finish): ");
input = scanner.next();
}
System.out.println("Final Array: " + Arrays.toString(array));
}
}
Output:
Enter a number (or type 'done' to finish): 1
Enter another number (or type 'done' to finish): 2
Enter another number (or type 'done' to finish): 3
Enter another number (or type 'done' to finish): 4
Enter another number (or type 'done' to finish): 5
Enter another number (or type 'done' to finish): done
Final Array: [1, 2, 3, 4, 5]
In this example, the array starts empty, and elements are dynamically added based on user input. The Arrays.copyOf
method is used to resize the array as needed.
Understanding this array initialization approach provides you with a versatile tool for handling dynamic scenarios where the size of the array evolves during program execution.
Conclusion
Initializing an empty array in Java might seem a bit daunting at first, but breaking it down into smaller steps can make the process more manageable.
Understanding the basics of the new
keyword and the different ways to initialize arrays sets a solid foundation for working with arrays in Java.
As you get more comfortable with these concepts, you’ll find arrays to be powerful tools for handling collections of data in your programs.