How to Initialize an Array in Constructor in Java
- Initialize Array Using Direct Initialization Method
- Initialize Array Using Arrays Class
- Initialize Array Using Constructor Body Initialization Method
-
Initialize Array Using
Arrays.copyOf()
-
Initialize Array Using
Arrays.fill()
-
Initialize Array Using
Arrays.setAll()
- Conclusion
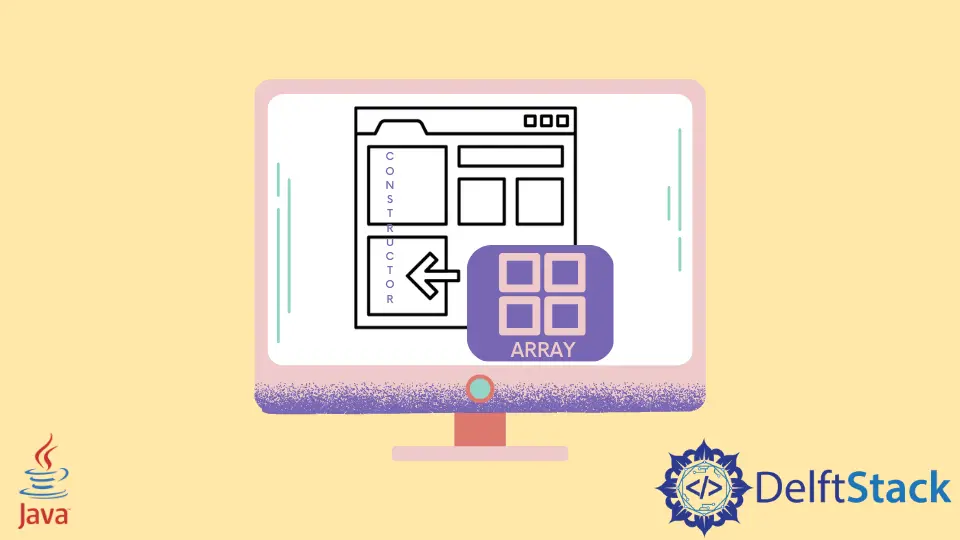
This tutorial introduces methods on how to initialize an array in a constructor in Java and also provides example codes to enhance understanding of the topic.
An array is an index-based data structure that is used to store similar types of data. In Java, we can use an array to store primitive and object values.
An array is also an object in Java and initialized with default values. For example, 0
for int, 0.0
for float/double, and null
for String/object values.
Initializing arrays in Java is crucial for defining their initial state and ensuring predictable behavior in a program. Proper initialization sets the foundation for array operations, preventing unexpected errors or undefined values.
By specifying initial values or allocating memory, developers establish a clear starting point for array elements. This process is fundamental for array-based algorithms, data structures, and overall program logic, contributing to code reliability and functionality.
Initialize Array Using Direct Initialization Method
Initializing arrays in constructors using direct initialization ensures that the array starts with specific values, setting a defined initial state.
This practice is crucial for creating reliable and predictable code preventing unexpected behavior. It establishes the foundation for array-based operations, contributing to the overall integrity and functionality of Java programs.
Let’s begin with a straightforward example demonstrating the direct initialization method in action. Consider a class named ArrayInitializer
that encapsulates an integer array. We’ll initialize this array directly within the constructor.
Example:
import java.util.Arrays;
public class ArrayInitializer {
private int[] myArray;
// Constructor with direct array initialization
public ArrayInitializer() {
this.myArray = new int[] {1, 2, 3, 4, 5};
}
// Getter method to retrieve the array
public int[] getMyArray() {
return myArray;
}
// Main method for demonstration
public static void main(String[] args) {
// Creating an instance of ArrayInitializer
ArrayInitializer arrayObj = new ArrayInitializer();
// Accessing the initialized array
int[] initializedArray = arrayObj.getMyArray();
// Displaying the array elements
System.out.println("Initialized Array: " + Arrays.toString(initializedArray));
}
}
In the ArrayInitializer
class, the constructor public ArrayInitializer()
takes care of the array initialization directly. The private myArray
field is set with values {1, 2, 3, 4, 5}
during object creation.
This direct initialization method provides a concise and readable way to establish the array’s initial state. The getMyArray()
method serves as an accessor, allowing external access to the initialized array while maintaining encapsulation.
In the main
method, an instance of the ArrayInitializer
class is created (ArrayInitializer arrayObj = new ArrayInitializer();
), and the initialized array is obtained using the accessor method. Finally, the array elements are displayed using System.out.println
.
This straightforward approach highlights the simplicity and effectiveness of initializing arrays directly within the constructor for predefined values.
The output of the provided example code would be:
Initialized Array: [1, 2, 3, 4, 5]
This output reflects the successful initialization of the array using the direct initialization method within the constructor.
The direct initialization method provides a clear and readable way to initialize arrays within Java constructors. By directly assigning values during object creation, this method streamlines the code and enhances code readability.
The example above illustrates a simple use case, demonstrating how this method can be employed to initialize arrays seamlessly.
Initialize Array Using Arrays Class
Initializing arrays in constructors using the Arrays class ensures a standardized and efficient approach. This method simplifies code, reducing complexity and potential errors.
By leveraging the Arrays
class, developers adhere to established conventions, promoting consistency and clarity. This streamlined process enhances readability and maintainability, contributing to overall code quality and reliability in Java.
Consider a class named ArrayInitializer
designed to encapsulate an integer array. This time, we will utilize the Arrays
class methods to initialize the array directly within the constructor.
Example:
import java.util.Arrays;
public class ArrayInitializer {
private int[] myArray;
// Constructor with array initialization using Arrays class method
public ArrayInitializer(int size) {
this.myArray = new int[size];
Arrays.fill(myArray, 42);
}
// Getter method to retrieve the array
public int[] getMyArray() {
return myArray;
}
// Main method for demonstration
public static void main(String[] args) {
// Creating an instance of ArrayInitializer
ArrayInitializer arrayObj = new ArrayInitializer(5);
// Accessing the initialized array
int[] initializedArray = arrayObj.getMyArray();
// Displaying the array elements
System.out.println("Initialized Array: " + Arrays.toString(initializedArray));
}
}
In the ArrayInitializer
class, the constructor public ArrayInitializer(int size)
is responsible for initializing an array with a specified size. Within the constructor, a new array of the given size is created using the line this.myArray = new int[size];
.
To populate the array with values, the constructor employs the Arrays.fill()
method from the java.util.Arrays
class. This method takes an array (a
) and a value (val
) as parameters, efficiently assigning the specified value (42
in this case) to every element of the array.
The getMyArray()
method serves as an accessor, enabling the retrieval of the initialized array from outside the class while adhering to encapsulation principles. The main
method demonstrates the practical usage of the ArrayInitializer
class by creating an instance and specifying the array size.
The initialized array is then obtained using the accessor method, and the array elements are displayed using System.out.println
. This concise approach illustrates the simplicity and effectiveness of using the Arrays.fill()
method to initialize arrays within a constructor, ensuring uniform default values for all elements.
The output of the provided example code would be:
Initialized Array: [42, 42, 42, 42, 42]
This output signifies the successful initialization of the array using the Arrays.fill()
method within the constructor. All elements of the array are set to the specified default value (42
).
Utilizing the Arrays.fill()
method within the constructor simplifies the process of array initialization. This approach is especially beneficial when a uniform default value needs to be assigned to all elements.
The example demonstrates how this method can be seamlessly integrated into a constructor, enhancing the efficiency and readability of the code.
Initialize Array Using Constructor Body Initialization Method
Initializing arrays in constructors using the constructor body method provides flexibility and customization. This method allows developers to incorporate specific logic or calculations during initialization, tailoring the process to unique requirements.
By doing so, it ensures a dynamic and personalized array setup, optimizing the constructor’s functionality for diverse scenarios.
Let’s consider a class named ArrayInitializer
that encapsulates an integer array. This time, we will initialize the array within the constructor body.
Example:
import java.util.Arrays;
public class ArrayInitializer {
private int[] myArray;
// Constructor with array initialization in the body
public ArrayInitializer(int size) {
this.myArray = new int[size];
initializeArray();
}
// Method to initialize the array
private void initializeArray() {
for (int i = 0; i < myArray.length; i++) {
myArray[i] = i + 1;
}
}
// Getter method to retrieve the array
public int[] getMyArray() {
return myArray;
}
// Main method for demonstration
public static void main(String[] args) {
// Creating an instance of ArrayInitializer
ArrayInitializer arrayObj = new ArrayInitializer(5);
// Accessing the initialized array
int[] initializedArray = arrayObj.getMyArray();
// Displaying the array elements
System.out.println("Initialized Array: " + Arrays.toString(initializedArray));
}
}
In the ArrayInitializer
class, the constructor public ArrayInitializer(int size)
is designed to initialize an array with a specified size. Within the constructor, a new array of the given size is created using the line this.myArray = new int[size];
.
To populate the array with values, the constructor calls the initializeArray()
method. The initializeArray()
method plays a key role in setting up the array elements.
In this example, a straightforward loop is employed to assign values to each element based on its index, ensuring a sequential order starting from 1. The getMyArray()
method serves as an accessor, allowing the initialized array to be retrieved from outside the class while adhering to encapsulation principles.
The main
method exemplifies the usage of the ArrayInitializer
class, creating an instance and specifying the array size. The initialized array is then obtained using the accessor method, and the array elements are displayed using System.out.println
.
This approach demonstrates a concise and effective way to initialize arrays within a constructor while maintaining encapsulation and flexibility through accessor methods.
The output of the provided example code would be:
Initialized Array: [1, 2, 3, 4, 5]
This output confirms the successful initialization of the array using the constructor body initialization method. The array elements are populated based on the custom logic defined in the initializeArray()
method.
Separating the initialization logic into a dedicated method promotes code readability and maintainability. The example illustrates how this method can be employed to initialize arrays with custom logic, offering flexibility in the array setup process.
Initialize Array Using Arrays.copyOf()
Initializing arrays in constructors with the Arrays.copyOf()
method ensures simplicity and adaptability. This method efficiently clones existing arrays, promoting concise code and minimizing redundancy.
By leveraging this approach, developers achieve a streamlined and readable solution while benefiting from the versatility to initialize arrays from various sources or specific portions.
Consider a class named ArrayInitializer
designed to encapsulate an integer array. This time, we’ll leverage the Arrays.copyOf()
method to initialize the array directly within the constructor.
Example:
import java.util.Arrays;
public class ArrayInitializer {
private int[] myArray;
// Constructor with array initialization using Arrays.copyOf() method
public ArrayInitializer(int[] sourceArray) {
this.myArray = Arrays.copyOf(sourceArray, sourceArray.length);
}
// Getter method to retrieve the array
public int[] getMyArray() {
return myArray;
}
// Main method for demonstration
public static void main(String[] args) {
// Creating an array to be used for initialization
int[] sourceArray = {1, 2, 3, 4, 5};
// Creating an instance of ArrayInitializer
ArrayInitializer arrayObj = new ArrayInitializer(sourceArray);
// Accessing the initialized array
int[] initializedArray = arrayObj.getMyArray();
// Displaying the array elements
System.out.println("Initialized Array: " + Arrays.toString(initializedArray));
}
}
In the ArrayInitializer
class, the constructor public ArrayInitializer(int[] sourceArray)
is designed to initialize a new array based on an existing one. The provided sourceArray
serves as the blueprint for the new array’s values.
Within the constructor, the Arrays.copyOf()
method, a member of the java.util.Arrays
class, is employed. This method takes two parameters: the original array (sourceArray
) and the new length of the array.
In our example, it efficiently duplicates the entire contents of sourceArray
into a new array (myArray
). The getMyArray()
method serves as an accessor, allowing the retrieval of the initialized array from outside the class while maintaining encapsulation principles.
The main
method illustrates the usage of the ArrayInitializer
class, where an array (sourceArray
) is created for initialization. An instance of the class is then created (ArrayInitializer arrayObj = new ArrayInitializer(sourceArray);
), and the initialized array is obtained using the accessor method.
Finally, the array elements are displayed using System.out.println
. This approach showcases the simplicity and effectiveness of the Arrays.copyOf()
method in dynamically initializing array elements within a constructor.
The output of the provided example code would be:
Initialized Array: [1, 2, 3, 4, 5]
This output confirms the successful initialization of the array using the Arrays.copyOf()
method within the constructor. The elements of the initialized array precisely match those of the sourceArray
, demonstrating the effectiveness of this initialization concept.
Leveraging the Arrays.copyOf()
method within the constructor provides a powerful way to initialize arrays in Java. This method offers flexibility by allowing arrays to be initialized from existing arrays or specific portions thereof.
The example illustrates how this method seamlessly integrates into a constructor, providing a concise and effective means of array initialization.
Initialize Array Using Arrays.fill()
Initializing arrays in constructors with the Arrays.fill()
method is crucial for simplicity and uniformity. This method efficiently populates the entire array with a specified value, ensuring consistency and reducing redundancy.
By employing Arrays.fill()
, developers streamline the initialization process, creating clean and concise code that is easy to read and maintain.
Consider a class named ArrayInitializer
designed to encapsulate an integer array. This time, we’ll employ the Arrays.fill()
method to initialize the array directly within the constructor.
Example:
import java.util.Arrays;
public class ArrayInitializer {
private int[] myArray;
// Constructor with array initialization using Arrays.fill() method
public ArrayInitializer(int size, int initValue) {
this.myArray = new int[size];
Arrays.fill(myArray, initValue);
}
// Getter method to retrieve the array
public int[] getMyArray() {
return myArray;
}
// Main method for demonstration
public static void main(String[] args) {
// Creating an instance of ArrayInitializer
ArrayInitializer arrayObj = new ArrayInitializer(5, 42);
// Accessing the initialized array
int[] initializedArray = arrayObj.getMyArray();
// Displaying the array elements
System.out.println("Initialized Array: " + Arrays.toString(initializedArray));
}
}
In the ArrayInitializer
class, the constructor public ArrayInitializer(int size, int initValue)
plays a key role in initializing an array. This constructor takes two parameters: size
, representing the desired size of the array, and initValue
, indicating the value with which each element of the array should be initialized.
Within the constructor, a new array of the specified size is created using the line this.myArray = new int[size];
. Subsequently, the Arrays.fill()
method from the java.util.Arrays
class is employed.
This method takes an array (a
) and a value (val
) as parameters, efficiently setting all elements of the array to the specified value. In our example, it initializes the entire array with the value provided in the constructor (initValue
).
The getMyArray()
method serves as an accessor, enabling the retrieval of the initialized array from outside the class while adhering to encapsulation principles. The main
method exemplifies the usage of the ArrayInitializer
class, creating an instance and specifying both the array size and the initialization value.
Finally, the initialized array’s elements are displayed using System.out.println
. This approach harnesses the simplicity and efficiency of the Arrays.fill()
method to initialize array elements dynamically within the constructor.
The output of the provided example code would be:
Initialized Array: [42, 42, 42, 42, 42]
This output signifies the successful initialization of the array using the Arrays.fill()
method within the constructor. All elements of the array are set to the specified default value (42
), demonstrating the effectiveness of this initialization concept.
The Arrays.fill()
method offers a convenient and straightforward approach to initializing arrays within Java constructors. This method is particularly useful when a uniform default value needs to be assigned to all elements of the array.
Initialize Array Using Arrays.setAll()
Initializing arrays in constructors using the Arrays.setAll()
method offers dynamic and customized initialization. This method allows developers to apply index-dependent logic during array setup, providing flexibility.
By leveraging Arrays.setAll()
, code becomes concise, readable, and adaptable to specific requirements. This approach ensures efficient and personalized array initialization within constructors.
Consider a class named ArrayInitializer
designed to encapsulate an integer array. This time, we’ll harness the Arrays.setAll()
method to initialize the array directly within the constructor.
Example:
import java.util.Arrays;
public class ArrayInitializer {
private int[] myArray;
// Constructor with array initialization using Arrays.setAll() method
public ArrayInitializer(int size) {
this.myArray = new int[size];
Arrays.setAll(myArray, i -> i * 2);
}
// Getter method to retrieve the array
public int[] getMyArray() {
return myArray;
}
// Main method for demonstration
public static void main(String[] args) {
// Creating an instance of ArrayInitializer
ArrayInitializer arrayObj = new ArrayInitializer(5);
// Accessing the initialized array
int[] initializedArray = arrayObj.getMyArray();
// Displaying the array elements
System.out.println("Initialized Array: " + Arrays.toString(initializedArray));
}
}
In the ArrayInitializer
class, the constructor public ArrayInitializer(int size)
is designed to initialize an array based on a specified size. Within the constructor, a new array of the given size is created using the line this.myArray = new int[size];
.
Subsequently, the Arrays.setAll()
method, a member of the java.util.Arrays
class, is employed to efficiently initialize the array. This method takes an array and an IntUnaryOperator
, which is a functional interface representing an operation that accepts an integer and produces an integer.
In our case, the operator (i -> i * 2
) is applied to each index in the array, dynamically calculating and assigning values based on the index. The getMyArray()
method functions as an accessor, allowing the initialized array to be retrieved from outside the class while maintaining encapsulation principles.
The main
method exemplifies the usage of the ArrayInitializer
class by creating an instance and specifying the array size. Finally, the initialized array’s elements are displayed using System.out.println
.
This approach leverages the power of Arrays.setAll()
to dynamically initialize array elements within the constructor.
The output of the provided example code would be:
Initialized Array: [0, 2, 4, 6, 8]
This output confirms the successful initialization of the array using the Arrays.setAll()
method within the constructor. The array elements are calculated based on the provided formula (i * 2
), illustrating the dynamic nature and effectiveness of this initialization concept.
The Arrays.setAll()
method empowers Java developers to initialize arrays within constructors with a dynamic, index-dependent approach. This method is particularly useful when the array elements need to be calculated based on a specific formula or logic.
Conclusion
The choice of array initialization method in constructors depends on the specific needs of a Java program.
Direct initialization provides a straightforward and concise approach while initializing in the constructor body offers flexibility and customization.
Utilizing array classes like Arrays.copyOf()
, Arrays.fill()
, and Arrays.setAll()
streamlines the process, each serving distinct purposes. Arrays.copyOf()
excels in efficient cloning, Arrays.fill
() ensures uniformity, and Arrays.setAll()
allows dynamic initialization.
Ultimately, selecting the appropriate method aligns with the coding requirements, promoting clarity, efficiency, and maintainability in array initialization within constructors.