How to Remove Whitespace From String in Java
- How to Remove Whitespace From String in Java
-
Remove Whitespace Using
replaceAll()
in Java -
Remove
whitespace
UsingApache
Library in Java -
Remove Whitespace Using
Pattern
andMatcher
in Java - Remove Space From a String in Java
-
Remove Space Using
Apache
in Java
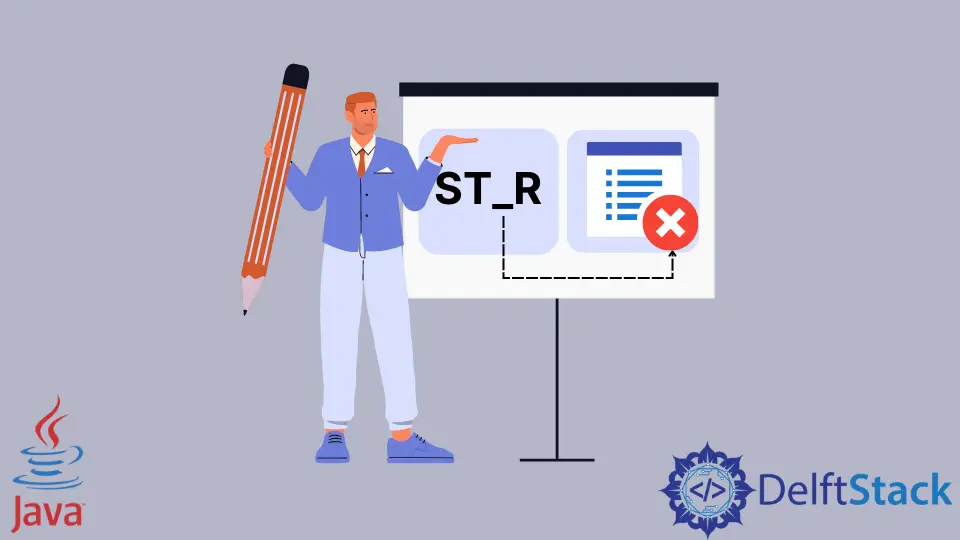
This tutorial introduces how to remove whitespace from string in Java and lists some example codes to understand the space removing process.
How to Remove Whitespace From String in Java
Whitespace is a character that represents a space into a string. The whitespace character can be a " "
, \n
, \t
, etc. To remove these characters from a string, there are several ways for example replace()
method, replaceAll()
, regex
, etc. Let’s see the examples below.
Remove Whitespace Using replaceAll()
in Java
Here, we use replaceAll()
method of String class to remove whitespace. This method takes a regex
as an argument and returns a string after removing all the whitespaces.
public class SimpleTesting {
public static void main(String[] args) {
String str = "Programming is easy to learn";
String result = str.replaceAll("\\s+", "");
System.out.println(result);
}
}
Output:
Programminiseasytolearn
Remove whitespace
Using Apache
Library in Java
If you work with the Apache
library, then use deleteWhitespace()
method of the StringUtils
class to remove whitespaces from a string in Java. See the example below and output.
import org.apache.commons.lang3.StringUtils;
public class SimpleTesting {
public static void main(String[] args) {
String str = "Programming is easy to learn";
String result = StringUtils.deleteWhitespace(str);
System.out.println(result);
}
}
Output:
Programminiseasytolearn
Remove Whitespace Using Pattern
and Matcher
in Java
We can use Pattern
and Matcher
class with replaceAll()
method to remove all the whitespaces from string in Java.
import java.util.regex.Matcher;
import java.util.regex.Pattern;
public class SimpleTesting {
public static void main(String[] args) {
String str = "Programming is easy to learn";
Pattern p = Pattern.compile("[\\s]");
Matcher m = p.matcher(str);
String result = m.replaceAll("");
System.out.println(result);
}
}
Output:
Programminiseasytolearn
Remove Space From a String in Java
If you want to remove space only from a string, then use replace()
method of String class. It will replace all the space (not all whitespace, for example, \n
and \t
) from the string in Java.
public class SimpleTesting {
public static void main(String[] args) {
String str = "Programming is easy to learn";
String result = str.replace(" ", "");
System.out.println(result);
}
}
Output:
Programminiseasytolearn
Remove Space Using Apache
in Java
Here, we use replace()
method of StringUtils
class of Apache
to replace all the spaces from string in Java.
import org.apache.commons.lang3.StringUtils;
public class SimpleTesting {
public static void main(String[] args) {
String str = "Programming is easy to learn";
String result = StringUtils.replace(str, " ", "");
System.out.println(result);
}
}
Output:
Programminiseasytolearn