如何在 Java 中删除字符串中的空格
Mohammad Irfan
2023年10月12日
Java
Java String
- 在 Java 中如何删除字符串中的空白处
-
在 Java 中使用
replaceAll()
删除空白字符 -
在 Java 中使用
Apache
库删除whitespace
- 在 Java 中使用 Pattern 和 Matcher 删除空白字符
- 在 Java 中删除字符串中的空格
-
在 Java 中使用
Apache
删除空格
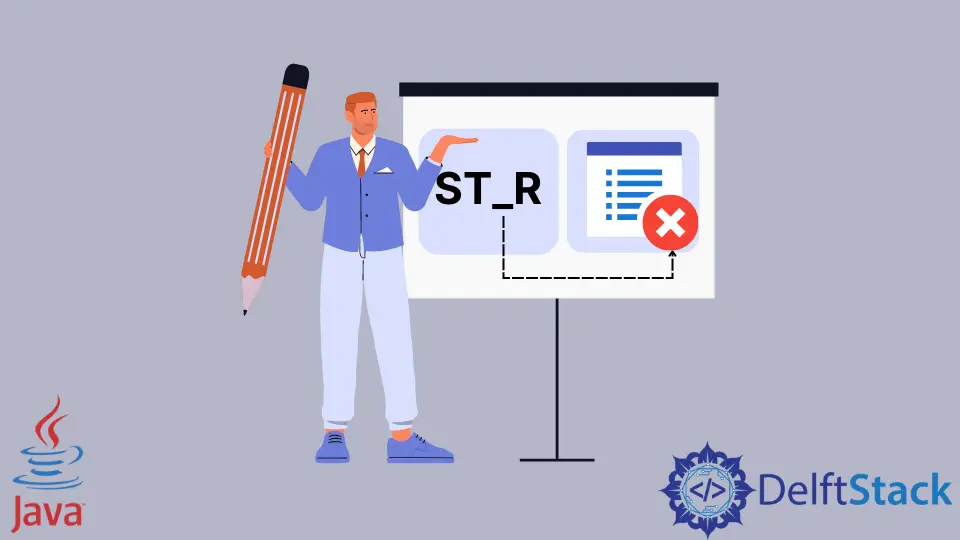
本教程介绍了如何在 Java 中从字符串中删除空格,并列举了一些示例代码来了解空格删除过程。
在 Java 中如何删除字符串中的空白处
空格是指在字符串中表示一个空格的字符,它可以是" "
、\
n、\t
等。要从字符串中删除这些字符,有几种方法,例如 replace()
方法,replaceAll()
,regex
等。让我们看看下面的例子。
在 Java 中使用 replaceAll()
删除空白字符
在这里,我们使用 String
类的 replaceAll()
方法来删除空格。这个方法使用一个 regex
作为参数,并在去除所有空白后返回一个字符串。
public class SimpleTesting {
public static void main(String[] args) {
String str = "Programming is easy to learn";
String result = str.replaceAll("\\s+", "");
System.out.println(result);
}
}
输出:
Programminiseasytolearn
在 Java 中使用 Apache
库删除 whitespace
如果你使用 Apache
库,那么可以使用 StringUtils
类的 deleteWhitespace()
方法在 Java 中删除字符串中的空白。请看下面的例子和输出。
import org.apache.commons.lang3.StringUtils;
public class SimpleTesting {
public static void main(String[] args) {
String str = "Programming is easy to learn";
String result = StringUtils.deleteWhitespace(str);
System.out.println(result);
}
}
输出:
Programminiseasytolearn
在 Java 中使用 Pattern 和 Matcher 删除空白字符
我们可以使用 Pattern
和 Matcher
类与 replaceAll()
方法来删除 Java 字符串中的所有空格。
import java.util.regex.Matcher;
import java.util.regex.Pattern;
public class SimpleTesting {
public static void main(String[] args) {
String str = "Programming is easy to learn";
Pattern p = Pattern.compile("[\\s]");
Matcher m = p.matcher(str);
String result = m.replaceAll("");
System.out.println(result);
}
}
输出:
Programminiseasytolearn
在 Java 中删除字符串中的空格
如果你只想从一个字符串中删除空格,那么使用 String
类的 replace()
方法。在 Java 中,它将替换掉字符串中所有的空格(不是所有的空白符号,比如\t
,\n
不会被删除。)。
public class SimpleTesting {
public static void main(String[] args) {
String str = "Programming is easy to learn";
String result = str.replace(" ", "");
System.out.println(result);
}
}
输出:
Programminiseasytolearn
在 Java 中使用 Apache
删除空格
在这里,我们使用 Apache
的 StringUtils
类的 replace()
方法来替换 Java 中字符串的所有空格。
import org.apache.commons.lang3.StringUtils;
public class SimpleTesting {
public static void main(String[] args) {
String str = "Programming is easy to learn";
String result = StringUtils.replace(str, " ", "");
System.out.println(result);
}
}
输出:
Programminiseasytolearn
Enjoying our tutorials? Subscribe to DelftStack on YouTube to support us in creating more high-quality video guides. Subscribe