How to Initialize a String Array in Java
- Initialize Array to the Default Value in Java
- Initialize Array to Direct Values in Java
-
Initialize Array to Values Using
Stream
in Java
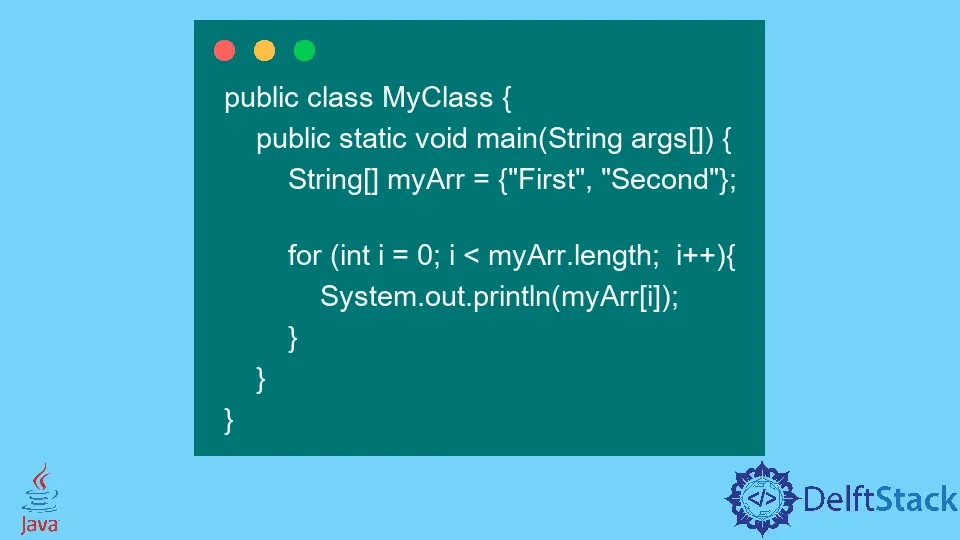
This tutorial introduces methods to initialize a string array in Java.
Java array is of fixed length; therefore, we need to declare an array and initialize it so that it can allocate the correct memory storage for the elements. If we only declare the array, there is no memory allocated for the elements, and it will throw an error when you try to assign a value to any index of the array.
Initialize Array to the Default Value in Java
The below example illustrates how to initialize a String array in Java. It creates an array of the specified length containing the default values null
at all the indexes, set to the desired value later.
public class MyClass {
public static void main(String args[]) {
String[] myArr;
myArr = new String[5];
myArr[0] = "First";
for (int i = 0; i < myArr.length; i++) {
System.out.println(myArr[i]);
}
}
}
Output:
First
null
null
null
null
We reassigned the value at the first index to "First"
while all the other indexes contain the default value null
.
Let’s see what happens if we try to assign a value to any array index without initializing the array.
public class MyClass {
public static void main(String args[]) {
String[] myArr;
myArr[0] = "First";
}
}
Output:
/MyClass.java:4: error: variable myArr might not have been initialized
myArr[0] = "First";
^
1 error
Initialize Array to Direct Values in Java
We can also initialize an array with the values we need in the array. In this case, the length of the array would be fixed to the number of elements we initialize it to. The below example illustrates this:
public class MyClass {
public static void main(String args[]) {
String[] myArr = {"First", "Second"};
for (int i = 0; i < myArr.length; i++) {
System.out.println(myArr[i]);
}
}
}
Output:
First
Second
The above block of code created an array of length 2 since we provided 2 values in the initialization.
Initialize Array to Values Using Stream
in Java
For Java 8 and above, we can make use of Stream
to initialize an array of Strings with given values. The below example illustrates this:
import java.util.stream.*;
public class MyClass {
public static void main(String args[]) {
String[] strings = Stream.of("First", "Second", "Third").toArray(String[] ::new);
for (int i = 0; i < strings.length; i++) {
System.out.println(strings[i]);
}
}
}
Output:
First
Second
Third