How to Convert Long to Int in Java
-
Use Type Casting to Convert a
long
to anint
in Java -
Use
Math.toIntExac()
to Convert along
to anint
in Java
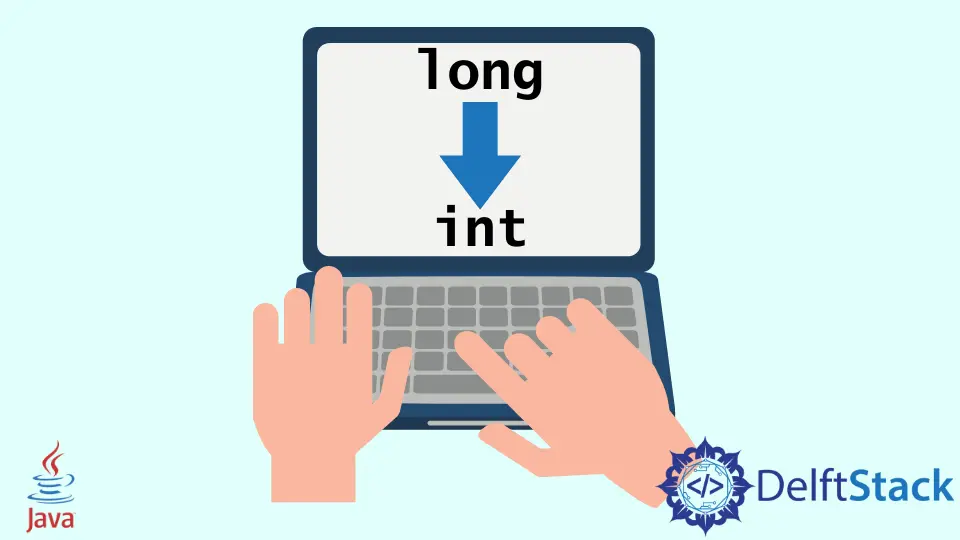
This tutorial discusses methods to convert a long
to an int
in Java.
Use Type Casting to Convert a long
to an int
in Java
The simplest way to convert a long
to an int
in Java is to type cast the long
to the int
using (int) longVar
. The below example illustrates this:
public class MyClass {
public static void main(String args[]) {
long myLong = 10000l;
int myInt = (int) myLong;
System.out.println("Value of long: " + myLong);
System.out.println("Value after conversion to int: " + myInt);
}
}
Output:
Value of long: 10000
Value after conversion to int: 10000
Use Math.toIntExac()
to Convert a long
to an int
in Java
In Java 8 and above, we can use the built-in Math
class method - Math.toIntExac()
to convert a long
to an int
in Java. The below example illustrates this:
public class MyClass {
public static void main(String args[]) {
long myLong = 10000l;
int myInt = Math.toIntExact(myLong);
System.out.println("Value of long: " + myLong);
System.out.println("Value after conversion to int: " + myInt);
}
}
Output:
Value of long: 10000
Value after conversion to int: 10000
These are the two commonly used methods to convert a long
to an int
in Java. However, we need to ensure that the long
value we have can be stored entirely in the int
since both have different memory limits, 32 bits vs 64 bits.
Both methods behave differently when we try to convert a long
value that is greater than 32 bits to an int
.
The below example illustrates how typecasting behaves in this case.
public class MyClass {
public static void main(String args[]) {
long myLong = 10000000000l;
int myInt = (int) myLong;
System.out.println("Value of long: " + myLong);
System.out.println("Value after conversion to int: " + myInt);
}
}
Output:
Value of long: 10000000000
Value after conversion to int: 1410065408
Note that the resulting converted value is wrong since we can not fit this long
value in an int
variable.
The below example illustrates how Math.toIntExac()
behaves in this case.
public class MyClass {
public static void main(String args[]) {
long myLong = 10000000000l;
int myInt = Math.toIntExact(myLong);
System.out.println("Value of long: " + myLong);
System.out.println("Value after conversion to int: " + myInt);
}
}
Output:
> Exception in thread "main" java.lang.ArithmeticException: integer overflow
at java.base/java.lang.Math.toIntExact(Math.java:1071)
at MyClass.main(MyClass.java:4)
Note that this method gives an integer overflow
error instead of erroneously trying to fit the long
to an int
.