How to Check if a String Is a Number in Java
- Check if a String Is a Number in Java
-
Check if a String Is a Number Using the
Character
Class in Java - Check if a String Is a Number Using Apache Library in Java
-
Check if a String Is a Number Using the
Double
Class in Java - Check if a String Is a Number in Java 8
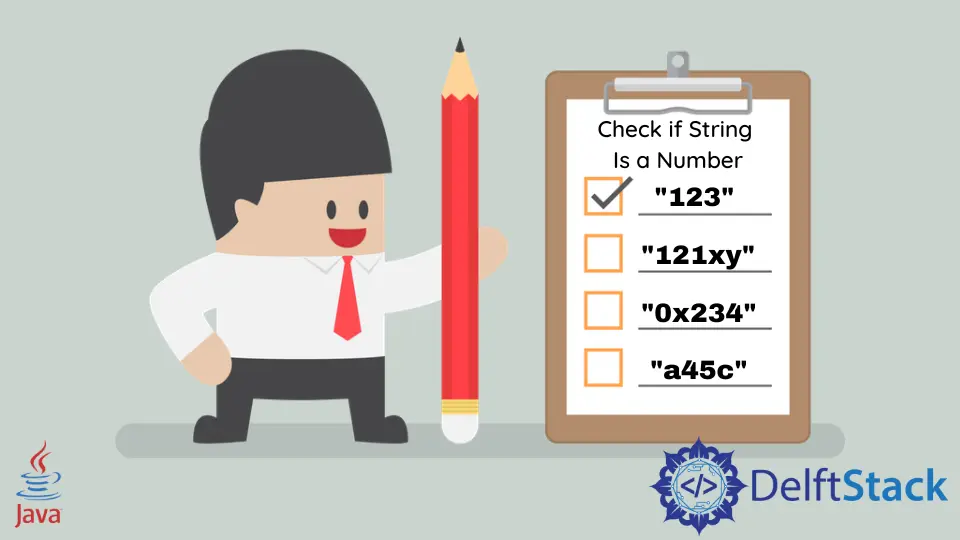
This tutorial introduces how to check whether a string is numeric in Java and lists some example codes to understand it.
There are several ways to check numeric string like using regex, the Double
class, the Character
class or Java 8 functional approach, etc.
Check if a String Is a Number in Java
A String
is numeric if and only if it contains numbers (valid numeric digits). For example, "123"
is a valid numeric string while "123a"
not a valid numeric string because it contains an alphabet.
To check numeric string, we could use the matched()
method of the String
class that takes regex
as an argument and returns a boolean value either true
or false
.
public class SimpleTesting {
public static void main(String[] args) {
String str = "123";
boolean isNumeric = str.matches("[+-]?\\d*(\\.\\d+)?");
System.out.println(isNumeric);
str = "121xy";
isNumeric = str.matches("[+-]?\\d*(\\.\\d+)?");
System.out.println(isNumeric);
str = "0x234";
isNumeric = str.matches("[+-]?\\d*(\\.\\d+)?");
System.out.println(isNumeric);
}
}
Output:
true
false
false
Check if a String Is a Number Using the Character
Class in Java
We can use the isDigit()
method of the Character
class to check each character in a loop. It returns either true
or false
value.
public class SimpleTesting {
public static void main(String[] args) {
String str = "1123";
boolean isNumeric = true;
for (int i = 0; i < str.length(); i++) {
if (!Character.isDigit(str.charAt(i))) {
isNumeric = false;
}
}
System.out.println(isNumeric);
}
}
Output:
true
Check if a String Is a Number Using Apache Library in Java
If you are using Apache, you can use the isNumeric()
method of the StringUtils
class, which returns true
if it contains a numeric sequence.
import org.apache.commons.lang3.StringUtils;
public class SimpleTesting {
public static void main(String[] args) {
String str = "1123";
boolean isNumeric = StringUtils.isNumeric(str);
System.out.println(isNumeric);
str = "123xyz";
isNumeric = StringUtils.isNumeric(str);
System.out.println(isNumeric);
}
}
Output:
true
false
Check if a String Is a Number Using the Double
Class in Java
We can use the parseDouble()
method of Double class that converts a string to double and returns a double type value. It throws an exception if it cannot be parsed.
public class SimpleTesting {
public static void main(String[] args) {
String str = "1123";
try {
Double.parseDouble(str);
System.out.println("It is a numerical string");
} catch (NumberFormatException e) {
System.out.println("It is not a numerical string");
}
}
}
Output:
It is a numerical string
Check if a String Is a Number in Java 8
If you are using Java 8 or higher version, then you can use this example to check numeric string. Here, the isDigit()
method of the Character
class is passed in allMatch()
as a method reference.
public class SimpleTesting {
public static void main(String[] args) {
String str = "1123";
boolean isNumeric = str.chars().allMatch(Character::isDigit);
System.out.println(isNumeric);
str = "ab234";
isNumeric = str.chars().allMatch(Character::isDigit);
System.out.println(isNumeric);
}
}
Output:
true
false