如何在 Java 中检查一个字符串是否是一个数字
Mohammad Irfan
2023年10月12日
Java
Java String
- 在 Java 中检查一个字符串是否是数字
-
使用 Java 中的
Character
类检查字符串是否是数字 - 在 Java 中使用 Apache 库检查一个字符串是否是一个数字
-
使用 Java 中的
Double
类检查一个字符串是否是一个数字 - 在 Java 8 中检查一个字符串是否是一个数字
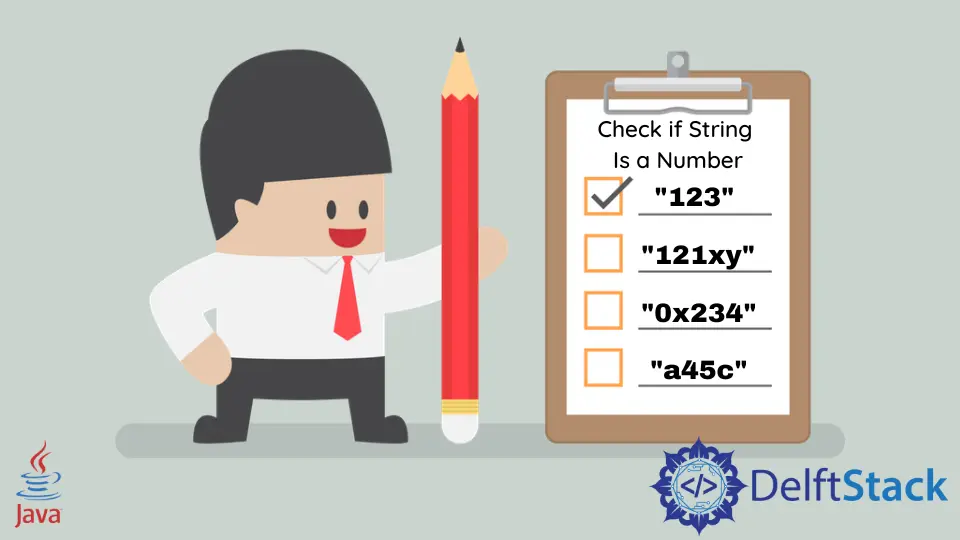
本教程将介绍在 Java 中如何检查一个字符串是否为数字,并列举了一些示例代码来了解它。
检查数字字符串的方法有好几种,如使用 regex、Double
类、Character
类或 Java 8 函数式方法等。
在 Java 中检查一个字符串是否是数字
如果且仅当字符串包含数字(有效的数字位)时,它才是数字字符串。例如,"123"
是一个有效的数字字符串,而 "123a"
不是一个有效的数字字符串,因为它包含一个字母。
为了检查数字字符串,我们可以使用 String
类的 matched()
方法,该方法以 regex
为参数,并返回一个布尔值 true
或 false
。
public class SimpleTesting {
public static void main(String[] args) {
String str = "123";
boolean isNumeric = str.matches("[+-]?\\d*(\\.\\d+)?");
System.out.println(isNumeric);
str = "121xy";
isNumeric = str.matches("[+-]?\\d*(\\.\\d+)?");
System.out.println(isNumeric);
str = "0x234";
isNumeric = str.matches("[+-]?\\d*(\\.\\d+)?");
System.out.println(isNumeric);
}
}
输出:
true
false
false
使用 Java 中的 Character
类检查字符串是否是数字
我们可以使用 Character
类的 isDigit()
方法来检查循环中的每个字符。它返回 true
或 false
值。
public class SimpleTesting {
public static void main(String[] args) {
String str = "1123";
boolean isNumeric = true;
for (int i = 0; i < str.length(); i++) {
if (!Character.isDigit(str.charAt(i))) {
isNumeric = false;
}
}
System.out.println(isNumeric);
}
}
输出:
true
在 Java 中使用 Apache 库检查一个字符串是否是一个数字
如果你使用的是 Apache 库,你可以使用 StringUtils
类的 isNumeric()
方法,如果它包含一个数字序列,则返回 true
。
import org.apache.commons.lang3.StringUtils;
public class SimpleTesting {
public static void main(String[] args) {
String str = "1123";
boolean isNumeric = StringUtils.isNumeric(str);
System.out.println(isNumeric);
str = "123xyz";
isNumeric = StringUtils.isNumeric(str);
System.out.println(isNumeric);
}
}
输出:
true
false
使用 Java 中的 Double
类检查一个字符串是否是一个数字
我们可以使用 Double
类的 parseDouble()
方法,将一个字符串转换为 double
并返回一个 double
类型的值。如果它不能被解析,它会抛出一个异常。
public class SimpleTesting {
public static void main(String[] args) {
String str = "1123";
try {
Double.parseDouble(str);
System.out.println("It is a numerical string");
} catch (NumberFormatException e) {
System.out.println("It is not a numerical string");
}
}
}
输出:
It is a numerical string
在 Java 8 中检查一个字符串是否是一个数字
如果你使用的是 Java 8 或更高版本,那么你可以使用这个例子来检查数字字符串。这里,Character
类的 isDigit()
方法被传递到 allMatch()
作为方法引用。
public class SimpleTesting {
public static void main(String[] args) {
String str = "1123";
boolean isNumeric = str.chars().allMatch(Character::isDigit);
System.out.println(isNumeric);
str = "ab234";
isNumeric = str.chars().allMatch(Character::isDigit);
System.out.println(isNumeric);
}
}
输出:
true
false
Enjoying our tutorials? Subscribe to DelftStack on YouTube to support us in creating more high-quality video guides. Subscribe