How to Call a Variable From Another Method in Java
- Call a Static Variable in a Static Method Within the Same Class in Java
- Call a Static Variable From a Non-Static Method Within the Same Class in Java
- Pass Variables as Parameters to Another Method in Java
- Conclusion
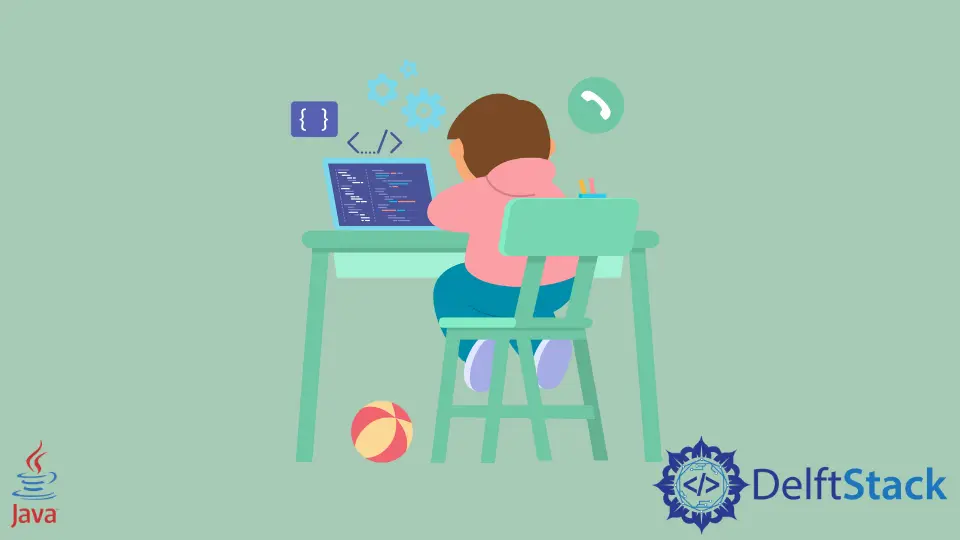
The ability to call a variable from one method to another is a fundamental aspect of building modular and efficient code. Whether you are working on a small project or a large-scale application, understanding various techniques for accessing variables across methods is crucial.
This article explores different methods, from leveraging class-level variables and static modifiers to passing variables as parameters. Each approach offers a unique solution to the common challenge of sharing data between methods within the same class.
Call a Static Variable in a Static Method Within the Same Class in Java
In Java, static variables provide a way to share a variable across different methods within the same class without the need to create an instance of the class. This is particularly useful when you want to maintain a common piece of data that should be accessible from various methods.
To declare a static variable, use the static
keyword followed by the data type and the variable name.
Here’s the syntax:
public class ClassName {
static DataType variableName;
// ... other members and methods
}
Consider the following example:
public class VariableCaller {
static int sharedVariable = 42;
public static void main(String[] args) {
System.out.println("In the main method, sharedVariable is: " + sharedVariable);
anotherMethod();
}
public static void anotherMethod() {
System.out.println("In anotherMethod, sharedVariable is: " + sharedVariable);
}
}
In this example, we declare a class named VariableCaller
with a static variable sharedVariable
of type int
. In the main
method, we print the value of sharedVariable
and then call the anotherMethod
.
In the anotherMethod
, we again print the value of sharedVariable
. Since sharedVariable
is declared static, it can be accessed directly from both the main
method and anotherMethod
without the need to create an instance of the class.
When you run this program, it will output:
In the main method, sharedVariable is: 42
In anotherMethod, sharedVariable is: 42
This demonstrates how a static variable can be used to share a value between different methods within the same class in Java. The static variable sharedVariable
maintains its value across method calls, providing a convenient way to share data within the class.
Call a Static Variable From a Non-Static Method Within the Same Class in Java
When dealing with non-static methods, there are specific considerations to keep in mind when accessing static variables.
Since non-static methods are associated with an instance of a class, and static variables are not bound to any particular instance, we need to handle the access in a way that aligns with the principles of object-oriented programming.
To access a static variable within a non-static method, use the class name followed by the dot (.
) operator and the variable name:
ClassName.variableName;
Consider the following example:
public class VariableCaller {
int instanceVariable = 10;
static int sharedVariable = 42;
public static void main(String[] args) {
VariableCaller instance = new VariableCaller();
instance.accessStaticVariable();
}
public void accessStaticVariable() {
System.out.println(
"In accessStaticVariable method, sharedVariable is: " + VariableCaller.sharedVariable);
}
}
In this example, we define a class VariableCaller
with an instance variable instanceVariable
and a static variable sharedVariable
. In the main
method, we create an instance of VariableCaller
and call the accessStaticVariable
method on that instance.
Inside the accessStaticVariable
method, we print the value of the static variable sharedVariable
using the class name VariableCaller
. To access the static variable within a non-static method, we reference it using the class name, not through the instance.
When you run this program, it will output:
In accessStaticVariable method, sharedVariable is: 42
This illustrates the correct way to access a static variable from a non-static method by using the class name. Remember, static variables are shared among all instances of a class, so referencing them through the class name ensures consistency and clarity in your code.
Pass Variables as Parameters to Another Method in Java
In Java, passing variables as parameters serves as a versatile mechanism for enabling method access to specific values. This approach is particularly useful when a method requires access to a variable declared in another method.
By passing the variable as a parameter, we establish a direct communication channel between the methods.
To pass a variable as a parameter, declare the method with the appropriate data type and include the variable as an argument:
public class ClassName {
// ... other members and methods
public static void methodName(DataType variableName) {
// Access 'variableName' here
// ... method body
}
}
Consider the following example:
public class VariablePasser {
public static void main(String[] args) {
int myVariable = 10;
anotherMethod(myVariable);
}
public static void anotherMethod(int variable) {
System.out.println("In anotherMethod, received variable is: " + variable);
}
}
In this example, we create a class named VariablePasser
. In the main
method, an int
variable named myVariable
is declared and assigned a value of 10
.
Subsequently, the anotherMethod
is called, and myVariable
is passed as a parameter. Inside anotherMethod
, the received parameter is printed.
This approach allows the anotherMethod
to access the value of myVariable
without relying on class-level variables or static modifiers. The parameter acts as a conduit for passing data between methods.
When you run this program, it will output:
In anotherMethod, received variable is: 10
This showcases the effectiveness of passing variables as parameters, providing a dynamic and modular way to share data between different methods in Java. This method allows for a more targeted and controlled approach to variable access, promoting encapsulation and maintainability in your code.
Conclusion
In Java, the effective management and sharing of variables between methods play a pivotal role in crafting robust and maintainable code. We’ve explored several strategies, ranging from the use of static variables and class-level variables to passing variables as parameters.
Each technique brings its advantages, offering developers flexibility and control over their code structure. As you embark on your coding journey, mastering these methods empowers you to design elegant and modular solutions.
By choosing the right approach for your specific needs, you can enhance the clarity and efficiency of your Java programs, ultimately contributing to the development of scalable and well-organized software.
Rupam Saini is an android developer, who also works sometimes as a web developer., He likes to read books and write about various things.
LinkedInRelated Article - Java Class
- How to Make Private Inner Class Member Public in Java
- How to Decompile Java Class
- Allergy Class in Java
- Inner Class and Static Nested Class in Java
- Class Constant in Java
- Friend Class in Java