How to Fix Exception in Thread Main Java.Util.NoSuchElementException: No Line Found
-
NoSuchElementException: No Line Found
in Java - Causes and Solutions -
Handle
NoSuchElementException: No Line Found
Error in Java -
Best Practices to Prevent
NoSuchElementException: No Line Found
Error in Java - Conclusion
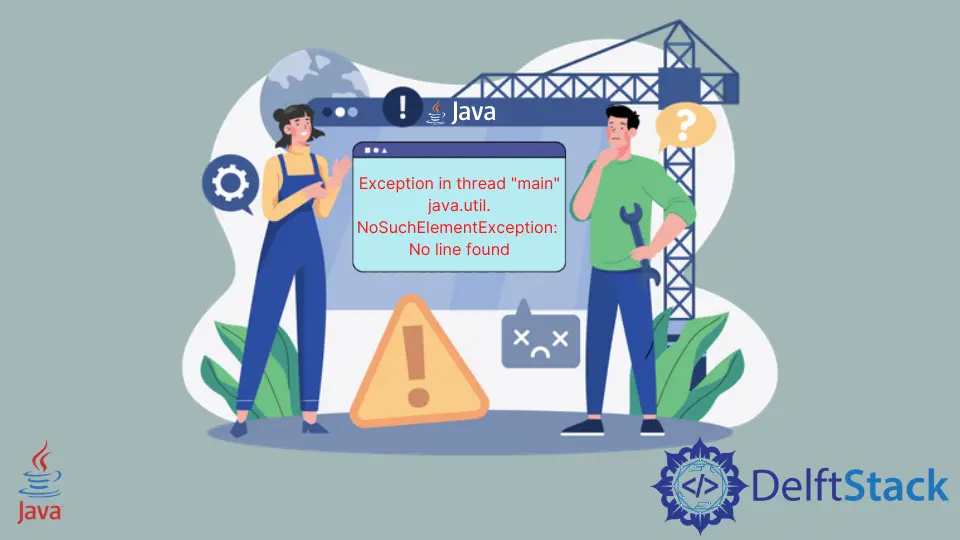
This article explores the common occurrence of the NoSuchElementException: No Line Found
error in Java, shedding light on its causes and providing effective solutions.
This article emphasizes the importance of proper input validation and correct usage of the Scanner
class, addressing issues arising from empty input sources.
By delving into best practices, developers can gain insights into preventing this error, ensuring robust code that gracefully handles diverse input conditions. Whether you are new to the concept or an experienced Java developer, these insights offer a concise guide to enhancing code stability and preventing unexpected program termination.
NoSuchElementException: No Line Found
in Java - Causes and Solutions
The NoSuchElementException: No Line Found
error in Java typically occurs when a program attempts to read a line using the nextLine()
method from a data source like a file or console, but no line is available for reading. This can happen if the input source is empty and the program does not validate whether there is a line to be read before attempting to do so.
Additionally, incorrect usage of the Scanner
class or making more nextLine()
calls than there are lines available can also lead to this exception.
Understanding the causes and solutions of NoSuchElementException: No Line Found
in Java is crucial for writing robust and error-resistant programs. It ensures that developers can anticipate and handle scenarios where input is absent or incomplete, preventing unexpected crashes.
By implementing proper validation and usage of input streams, developers enhance the reliability of their Java applications. This knowledge not only improves code quality but also contributes to a smoother user experience, as the program gracefully handles situations where expected input is not available.
Overall, familiarity with these causes and solutions is fundamental for creating resilient and user-friendly Java applications.
Here are common causes and solutions for this exception:
Issue | Cause | Solution |
---|---|---|
Empty Input Source | The input source (e.g., file, console input stream) is empty. | Ensure that the input source contains data to be read. Verify that the file is not empty or there is input available in the console. |
Missing Input Validation | Failing to check if there is another line available before calling nextLine() on a Scanner object. |
Use hasNextLine() to check if there is a line available before attempting to read it. |
Incorrect Scanner Usage |
Incorrect usage of the Scanner methods or not resetting the Scanner when needed. |
Verify that the Scanner object is correctly instantiated and used. Reset the Scanner if reusing it. |
Unmatched nextLine() Calls |
Making more nextLine() calls than there are lines available. |
Ensure that the number of nextLine() calls matches the number of available lines. |
Understanding and addressing these causes is essential for writing robust Java programs that handle input scenarios effectively.
Handle NoSuchElementException: No Line Found
Error in Java
In Java programming, dealing with exceptions is an essential skill for writing robust and reliable code. One common exception that developers often encounter is the NoSuchElementException
, particularly when working with input streams and attempting to read lines that may not exist.
This exception, if left unhandled, can lead to runtime errors and unexpected program termination.
Preventing the Exception in Thread Main Java.Util.NoSuchElementException: No Line Found
error is crucial for writing robust and reliable Java code. By incorporating preventative measures, such as checking for the availability of a line using hasNextLine()
before attempting to read it, developers ensure that their programs gracefully handle scenarios where input is absent.
This proactive approach prevents unexpected program termination, enhances user experience, and contributes to overall code stability. By addressing potential issues related to input availability, developers create more resilient applications capable of handling varying input conditions, leading to improved software quality and user satisfaction.
Let’s dive into a complete working example that demonstrates the correct ways to handle the NoSuchElementException
. We’ll use the Scanner
class to read lines from the console, providing insightful comments along the way.
import java.util.NoSuchElementException;
import java.util.Scanner;
public class NoSuchElementExceptionExample {
public static void main(String[] args) {
// Use hasNextLine() check to prevent NoSuchElementException
Scanner scanner = new Scanner(System.in);
try {
// Check if there is another line available
if (scanner.hasNextLine()) {
// Read the next line
String line = scanner.nextLine();
// Process the line
System.out.println("Input line: " + line);
} else {
System.out.println("No lines available.");
}
} catch (NoSuchElementException e) {
// Handle the exception gracefully
System.out.println("Exception caught: " + e.getMessage());
} finally {
// Close the scanner to release resources
scanner.close();
}
}
}
In this code example, the process begins with the initialization of a Scanner
object, which is responsible for reading input from the console (System.in
). The entire code is enclosed within a try-catch
block, showcasing a robust exception-handling mechanism.
Within the try
block, a check is performed using hasNextLine()
to verify the availability of another line before attempting to read it. If a line is present, the code proceeds to read it using nextLine()
, enabling subsequent processing and display.
In the event of a NoSuchElementException
– indicating the absence of a line – the catch
block is triggered, gracefully handling the exception by printing an informative message. Finally, the finally
block ensures the proper closure of the Scanner
, releasing system resources irrespective of whether an exception occurred.
This structured approach ensures the code’s resilience to varying input conditions.
Output:
If there is input available, the program will successfully read and process the input line. If no lines are available, it will gracefully handle the NoSuchElementException
and inform the user that no lines are present.
This approach ensures that your Java program remains robust and user-friendly, handling both expected and unexpected scenarios when dealing with input streams.
Best Practices to Prevent NoSuchElementException: No Line Found
Error in Java
Input Validation
Ensure proper validation of input sources before attempting to read lines. Use methods like hasNextLine()
to check for the existence of input before invoking nextLine()
.
Try-Catch
Handling
Wrap code sections involving input reading in a try-catch
block. This allows for graceful handling of exceptions, preventing unexpected program termination.
Scanner
Usage Best Practices
Verify correct instantiation and usage of the Scanner
class. Be attentive to resetting the Scanner
when necessary, especially if reusing it for multiple inputs.
Matching Input Calls
Ensure the number of nextLine()
calls aligns with the available lines to prevent making more calls than there are lines in the input source.
Resource Management
Properly manage resources by closing the Scanner
in a finally
block. This ensures the release of system resources, contributing to code cleanliness and efficiency.
Conclusion
Understanding the causes and solutions for the NoSuchElementException: No Line Found
error in Java is pivotal for writing robust and error-resistant code. By addressing issues related to empty input sources, implementing proper input validation, and following best practices with the Scanner
class, developers can prevent unexpected program termination and create more resilient applications.
Incorporating these best practices not only enhances code stability but also contributes to a smoother user experience by gracefully handling scenarios where input is absent. This comprehensive approach ensures that Java programs are well-equipped to handle diverse input conditions, promoting reliability and user satisfaction.
Sheeraz is a Doctorate fellow in Computer Science at Northwestern Polytechnical University, Xian, China. He has 7 years of Software Development experience in AI, Web, Database, and Desktop technologies. He writes tutorials in Java, PHP, Python, GoLang, R, etc., to help beginners learn the field of Computer Science.
LinkedIn Facebook