Ausnahme im Hauptthread Java.Util.NoSuchElementException: Keine Zeile gefunden
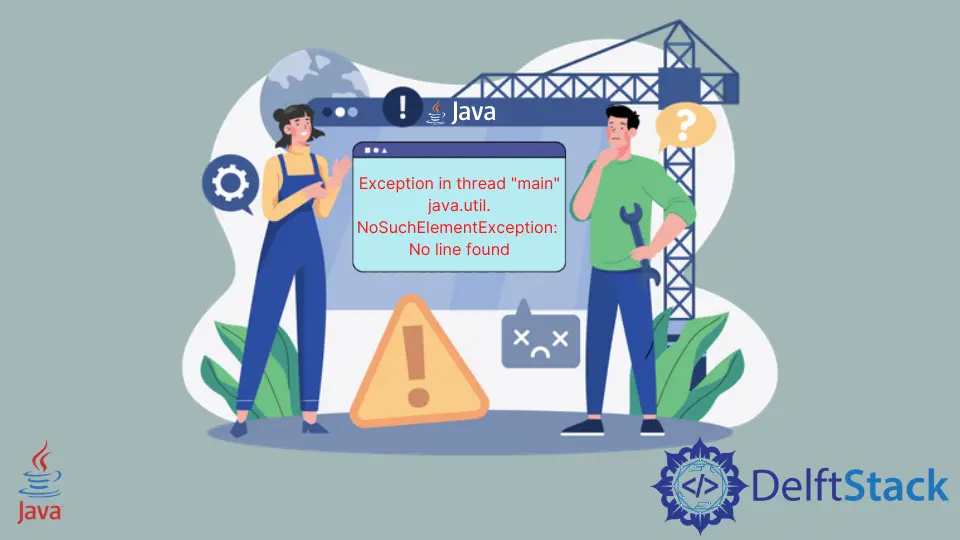
Dieses Tutorial demonstriert das Lösen der Exception in thread "main" java.util.NoSuchElementException: No line found
in Java.
Ausnahme in Thread "main" java.util.NoSuchElementException: Keine Zeile gefunden
Die java.util.NoSuchElementException
ist eine ungeprüfte Laufzeitausnahme. Die JVM löst diese Ausnahme aus, wenn wir Methoden wie next()
, nextElement()
, Iteratoren oder Methoden oder Enumerationen verwenden.
Der Fehler Exception in thread "main" java.util.NoSuchElementException: No line found
tritt auf, wenn wir einen Scanner
verwenden, um Benutzereingaben mit Methoden wie nextLine()
zu erhalten; Der Fehler tritt auf, wenn wir versuchen, die Methode ohne Begrenzung zu verwenden.
Lassen Sie uns ein Beispiel ausprobieren, das diesen Fehler demonstriert.
package delftstack;
import java.util.Scanner;
public class Example {
static boolean[][] Articles;
public static void main(String[] args) {
// This initiates all array values to be false
Articles = new boolean[4][4];
for (int i = 0; i < 4; i++) {
for (int j = 0; j < 4; j++) {
Articles[i][j] = false;
}
// Welcome message
System.out.println("-------------------------");
System.out.println("Welcome to Delftstack.com.");
System.out.println("-------------------------\n");
// Starts program
Programstart();
}
}
public static void Programstart() {
// to read users' input
Scanner sc = new Scanner(System.in);
// user input
String Requested_Lanuguage;
String Requested_Article;
// Counters for articles array
int Count_Language = 0;
int Count_Artciles = 0;
// User to select their choice of Programming Language
System.out.print("Please type 1 for Java or 2 for Python: ");
// Language preference
Requested_Lanuguage = sc.nextLine();
switch (Requested_Lanuguage) {
case "1":
// User selects Java
System.out.println(">>> You have selected Java. \n");
break;
case "2":
// User selects Python
System.out.println(">>> You have selected Python. \n");
break;
default:
// User has not selected a valid Programming Language
System.out.println(">>> You have not selected a valid choice. Please try again. \n");
Programstart();
break;
}
// user to select their choice of article
System.out.print("Please type 1 for Web and 2 for App: ");
// Article preference
Requested_Article = sc.nextLine();
switch (Requested_Article) {
case "1":
// User selects Web Articles
System.out.println(">>> You have selected Web Articles. \n");
break;
case "2":
// User selects App Articles
System.out.println(">>> You have selected App Articles. \n");
break;
default:
// User has not selected a valid article
System.out.println(">>> You have not selected a choice. Please try again. \n");
Programstart();
break;
}
// Closes Scanner
sc.close();
}
}
Die Ausgabe für den obigen Code lautet:
-------------------------
Welcome to Delftstack.com.
-------------------------
Please type 1 for Java or 2 for Python: 1
>>> You have selected Java.
Please type 1 for Web and 2 for App: 1
>>> You have selected Web Articles.
Exception in thread "main" -------------------------
Welcome to Delftstack.com.
-------------------------
Please type 1 for Java or 2 for Python: java.util.NoSuchElementException: No line found
at java.base/java.util.Scanner.nextLine(Scanner.java:1651)
at delftstack.Example.Programstart(Example.java:45)
at delftstack.Example.main(Example.java:24)
Der Fehler tritt auf, weil wir die Methode nextLine()
ohne Begrenzung verwenden. Um dieses Problem zu lösen, müssen wir den Code Requested_Article = sc.nextLine();
ersetzen. zu folgendem Code.
while (sc.hasNextLine()) {
Requested_Article = sc.nextLine();
// Switch condition here
}
Versuchen wir die Lösung.
package delftstack;
import java.util.Scanner;
public class Example {
static boolean[][] Articles;
public static void main(String[] args) {
// This initiates all array values to be false
Articles = new boolean[4][4];
for (int i = 0; i < 4; i++) {
for (int j = 0; j < 4; j++) {
Articles[i][j] = false;
}
// Welcome message
System.out.println("-------------------------");
System.out.println("Welcome to Delftstack.com.");
System.out.println("-------------------------\n");
// Starts program
Programstart();
}
}
public static void Programstart() {
// to read users' input
Scanner sc = new Scanner(System.in);
// user input
String Requested_Lanuguage;
String Requested_Article;
// Counters for articles array
int Count_Language = 0;
int Count_Artciles = 0;
// User to select their choice of Programming Language
System.out.print("Please type 1 for Java or 2 for Python: ");
// Language preference
Requested_Lanuguage = sc.nextLine();
switch (Requested_Lanuguage) {
case "1":
// User selects Java
System.out.println(">>> You have selected Java. \n");
break;
case "2":
// User selects Python
System.out.println(">>> You have selected Python. \n");
break;
default:
// User has not selected a valid Programming Language
System.out.println(">>> You have not selected a valid choice. Please try again. \n");
Programstart();
break;
}
// user to select their choice of article
System.out.print("Please type 1 for Web and 2 for App: ");
// Article preference
while (sc.hasNextLine()) {
Requested_Article = sc.nextLine();
switch (Requested_Article) {
case "1":
// User selects Web Articles
System.out.println(">>> You have selected Web Articles. \n");
break;
case "2":
// User selects App Articles
System.out.println(">>> You have selected App Articles. \n");
break;
default:
// User has not selected a valid article
System.out.println(">>> You have not selected a choice. Please try again. \n");
Programstart();
break;
}
}
// Closes Scanner
sc.close();
}
}
Der obige Code löst jetzt nicht die Exception in thread "main" java.util.NoSuchElementException: No line found
aus. Siehe die Ausgabe:
-------------------------
Welcome to Delftstack.com.
-------------------------
Please type 1 for Java or 2 for Python: 1
>>> You have selected Java.
Please type 1 for Web and 2 for App: 1
>>> You have selected Web Articles.
1
>>> You have selected Web Articles.
>>> You have not selected a choice. Please try again.
Please type 1 for Java or 2 for Python:
Sheeraz is a Doctorate fellow in Computer Science at Northwestern Polytechnical University, Xian, China. He has 7 years of Software Development experience in AI, Web, Database, and Desktop technologies. He writes tutorials in Java, PHP, Python, GoLang, R, etc., to help beginners learn the field of Computer Science.
LinkedIn Facebook