스레드 기본 Java.Util.NoSuchElementException의 예외: 줄을 찾을 수 없음
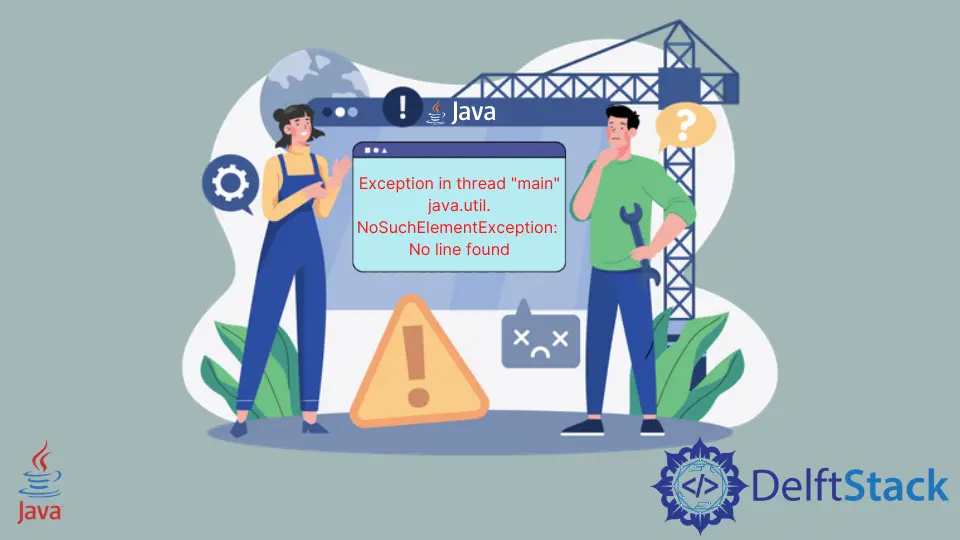
이 튜토리얼은 Java에서 스레드 "main" java.util.NoSuchElementException의 예외: 줄을 찾을 수 없음
을 해결하는 방법을 보여줍니다.
스레드 "main"의 예외 java.util.NoSuchElementException: 줄을 찾을 수 없음
java.util.NoSuchElementException
은 런타임 확인되지 않은 예외입니다. JVM은 next()
, nextElement()
, 반복자 또는 메소드 또는 열거와 같은 메소드를 사용할 때 이 예외를 발생시킵니다.
스레드 "main" 예외 java.util.NoSuchElementException: 라인을 찾을 수 없음
오류는 스캐너
를 사용하여 nextLine()
과 같은 메서드로 사용자 입력을 가져올 때 발생합니다. 경계 없이 메서드를 사용하려고 하면 오류가 발생합니다.
이 오류를 보여주는 예를 살펴보겠습니다.
package delftstack;
import java.util.Scanner;
public class Example {
static boolean[][] Articles;
public static void main(String[] args) {
// This initiates all array values to be false
Articles = new boolean[4][4];
for (int i = 0; i < 4; i++) {
for (int j = 0; j < 4; j++) {
Articles[i][j] = false;
}
// Welcome message
System.out.println("-------------------------");
System.out.println("Welcome to Delftstack.com.");
System.out.println("-------------------------\n");
// Starts program
Programstart();
}
}
public static void Programstart() {
// to read users' input
Scanner sc = new Scanner(System.in);
// user input
String Requested_Lanuguage;
String Requested_Article;
// Counters for articles array
int Count_Language = 0;
int Count_Artciles = 0;
// User to select their choice of Programming Language
System.out.print("Please type 1 for Java or 2 for Python: ");
// Language preference
Requested_Lanuguage = sc.nextLine();
switch (Requested_Lanuguage) {
case "1":
// User selects Java
System.out.println(">>> You have selected Java. \n");
break;
case "2":
// User selects Python
System.out.println(">>> You have selected Python. \n");
break;
default:
// User has not selected a valid Programming Language
System.out.println(">>> You have not selected a valid choice. Please try again. \n");
Programstart();
break;
}
// user to select their choice of article
System.out.print("Please type 1 for Web and 2 for App: ");
// Article preference
Requested_Article = sc.nextLine();
switch (Requested_Article) {
case "1":
// User selects Web Articles
System.out.println(">>> You have selected Web Articles. \n");
break;
case "2":
// User selects App Articles
System.out.println(">>> You have selected App Articles. \n");
break;
default:
// User has not selected a valid article
System.out.println(">>> You have not selected a choice. Please try again. \n");
Programstart();
break;
}
// Closes Scanner
sc.close();
}
}
위 코드의 출력은 다음과 같습니다.
-------------------------
Welcome to Delftstack.com.
-------------------------
Please type 1 for Java or 2 for Python: 1
>>> You have selected Java.
Please type 1 for Web and 2 for App: 1
>>> You have selected Web Articles.
Exception in thread "main" -------------------------
Welcome to Delftstack.com.
-------------------------
Please type 1 for Java or 2 for Python: java.util.NoSuchElementException: No line found
at java.base/java.util.Scanner.nextLine(Scanner.java:1651)
at delftstack.Example.Programstart(Example.java:45)
at delftstack.Example.main(Example.java:24)
경계 없이 nextLine()
메서드를 사용하기 때문에 오류가 발생합니다. 이 문제를 해결하려면 Requested_Article = sc.nextLine();
코드를 교체해야 합니다. 다음 코드로.
while (sc.hasNextLine()) {
Requested_Article = sc.nextLine();
// Switch condition here
}
해결책을 시도해 봅시다.
package delftstack;
import java.util.Scanner;
public class Example {
static boolean[][] Articles;
public static void main(String[] args) {
// This initiates all array values to be false
Articles = new boolean[4][4];
for (int i = 0; i < 4; i++) {
for (int j = 0; j < 4; j++) {
Articles[i][j] = false;
}
// Welcome message
System.out.println("-------------------------");
System.out.println("Welcome to Delftstack.com.");
System.out.println("-------------------------\n");
// Starts program
Programstart();
}
}
public static void Programstart() {
// to read users' input
Scanner sc = new Scanner(System.in);
// user input
String Requested_Lanuguage;
String Requested_Article;
// Counters for articles array
int Count_Language = 0;
int Count_Artciles = 0;
// User to select their choice of Programming Language
System.out.print("Please type 1 for Java or 2 for Python: ");
// Language preference
Requested_Lanuguage = sc.nextLine();
switch (Requested_Lanuguage) {
case "1":
// User selects Java
System.out.println(">>> You have selected Java. \n");
break;
case "2":
// User selects Python
System.out.println(">>> You have selected Python. \n");
break;
default:
// User has not selected a valid Programming Language
System.out.println(">>> You have not selected a valid choice. Please try again. \n");
Programstart();
break;
}
// user to select their choice of article
System.out.print("Please type 1 for Web and 2 for App: ");
// Article preference
while (sc.hasNextLine()) {
Requested_Article = sc.nextLine();
switch (Requested_Article) {
case "1":
// User selects Web Articles
System.out.println(">>> You have selected Web Articles. \n");
break;
case "2":
// User selects App Articles
System.out.println(">>> You have selected App Articles. \n");
break;
default:
// User has not selected a valid article
System.out.println(">>> You have not selected a choice. Please try again. \n");
Programstart();
break;
}
}
// Closes Scanner
sc.close();
}
}
위의 코드는 이제 Exception in thread "main" java.util.NoSuchElementException: No line found
를 발생시키지 않습니다. 출력을 참조하십시오.
-------------------------
Welcome to Delftstack.com.
-------------------------
Please type 1 for Java or 2 for Python: 1
>>> You have selected Java.
Please type 1 for Web and 2 for App: 1
>>> You have selected Web Articles.
1
>>> You have selected Web Articles.
>>> You have not selected a choice. Please try again.
Please type 1 for Java or 2 for Python:
Sheeraz is a Doctorate fellow in Computer Science at Northwestern Polytechnical University, Xian, China. He has 7 years of Software Development experience in AI, Web, Database, and Desktop technologies. He writes tutorials in Java, PHP, Python, GoLang, R, etc., to help beginners learn the field of Computer Science.
LinkedIn Facebook