How to Create An Empty Array in Java
- Understanding Empty and Null Arrays
- Method 1: Creating an Empty Array with Specified Size
- Method 2: Creating an Empty Array with No Size
- Method 3: Creating a Null Array
- Conclusion
- FAQ
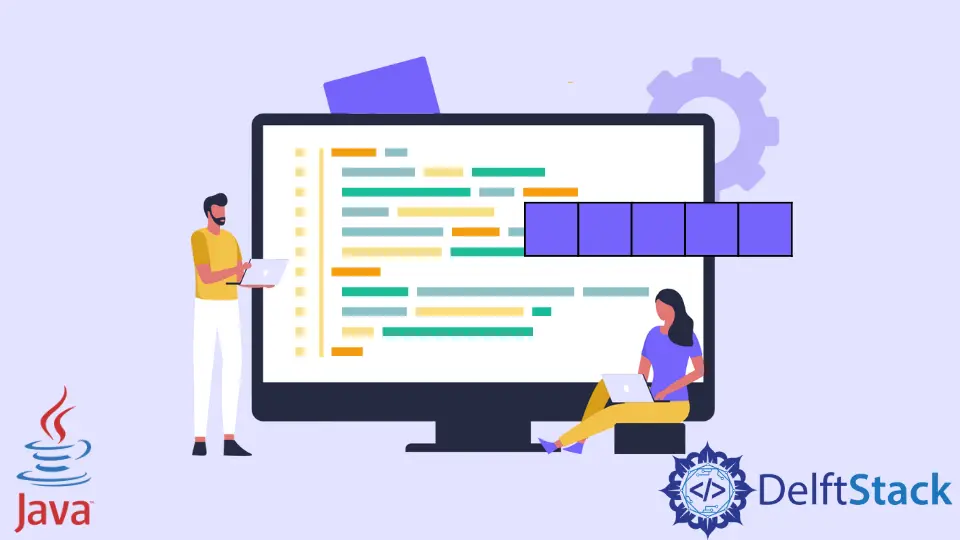
Creating an empty array in Java is a fundamental skill for any Java developer. Arrays are essential data structures that allow you to store multiple values in a single variable. Whether you are initializing a new project or working on an existing codebase, understanding how to create empty arrays can save you time and effort.
In this article, we’ll explore various methods to create both empty and null arrays in Java. By the end, you’ll have a solid grasp of how to manage arrays effectively in your Java applications. Let’s dive into the different approaches!
Understanding Empty and Null Arrays
Before we get into the methods of creating empty arrays, it’s important to understand the difference between an empty array and a null array. An empty array is an array that has been initialized but contains no elements. In contrast, a null array is a reference that points to no memory allocation. Knowing this distinction is crucial as it affects how you handle data in your applications.
Method 1: Creating an Empty Array with Specified Size
One of the most straightforward methods to create an empty array in Java is by defining its size during initialization. This method allows you to create an array that can hold a specific number of elements, but currently contains none.
public class Main {
public static void main(String[] args) {
int[] emptyArray = new int[5];
System.out.println("Length of the empty array: " + emptyArray.length);
}
}
Output:
Length of the empty array: 5
In this example, we create an integer array named emptyArray
with a size of 5. Although it is initialized, it does not contain any elements. The length of the array is 5, meaning it can hold five integers, but since we haven’t added any values yet, it is considered empty. This method is particularly useful when you know the number of elements you will eventually store in the array but want to initialize it without any data at the start.
Method 2: Creating an Empty Array with No Size
If you want to create an empty array without specifying its size, you can use the following approach. This method is beneficial when you don’t know beforehand how many elements you’ll need to store.
public class Main {
public static void main(String[] args) {
String[] emptyArray = new String[0];
System.out.println("Length of the empty array: " + emptyArray.length);
}
}
Output:
Length of the empty array: 0
Here, we create a String
array named emptyArray
with a size of zero. This means that the array is empty and cannot hold any elements. When you check the length of the array, it returns 0, indicating that there are no elements present. This method is particularly useful in scenarios where you might want to return an empty array from a method or initialize a collection without any data.
Method 3: Creating a Null Array
Creating a null array in Java is quite simple and is done by declaring the array without initializing it. This method is useful when you want to signify that the array has not been set up yet.
public class Main {
public static void main(String[] args) {
int[] nullArray = null;
System.out.println("Null array: " + nullArray);
}
}
Output:
Null array: null
In this example, we declare an integer array named nullArray
and do not initialize it. When we print the value of nullArray
, it outputs null
, indicating that the array reference does not point to any memory location. This can be useful in various scenarios, such as when you want to check whether an array has been set up or when you need to handle optional data.
Conclusion
Creating empty and null arrays in Java is a fundamental concept that every Java developer should master. Whether you choose to create an empty array with a specified size, an empty array with no size, or a null array, each method has its specific use cases. Understanding these differences will help you manage data more effectively in your Java applications. With the knowledge gained from this article, you can confidently implement arrays in your projects and enhance your coding skills.
FAQ
-
What is the difference between an empty array and a null array?
An empty array is initialized but contains no elements, while a null array is a reference that points to no memory allocation. -
Can you create an array without specifying its size?
Yes, you can create an empty array by specifying its size as zero, or by declaring it without initialization.
-
How do you check the length of an array in Java?
You can check the length of an array using the.length
property, which returns the number of elements that the array can hold. -
What happens if you try to access an element in a null array?
If you try to access an element in a null array, it will throw aNullPointerException
, indicating that you are trying to access a reference that does not point to any object. -
Is it possible to change the size of an array after it has been created?
No, arrays in Java have a fixed size once they are created. If you need a resizable array, consider using anArrayList
instead.