How to Declare an Array in Java
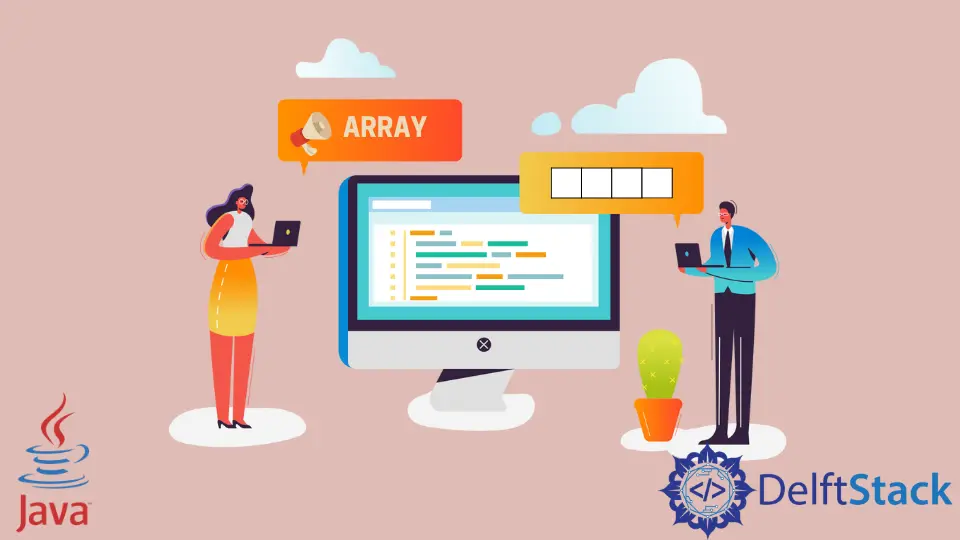
Declare an Array in Java
Below mentioned are few ways to declare a 1D array in the Java language. The detailed description is given after the given code.
import java.util.stream.IntStream;
public class DeclareAnArray {
public static void main(String[] args) {
int[] intArr1 = new int[3];
int[] intArr2 = {3, 4, 5};
int[] intArr3 = new int[] {3, 4, 5};
int intArr4[] = IntStream.range(0, 10).toArray();
int[] intArr5 = IntStream.of(2, 5, 3, 8, 1, 9, 7).toArray();
int[][] intArr6 = new int[5][2];
int intArr7[][] = new int[5][2];
int[] intArr8[] = new int[5][2];
int[][] intArr9 = {{1, 2}, {1, 2}, {1, 2}, {1, 2}, {1, 2}};
}
}
The description is given sequentially in order of statements in the code.
int[] intArr1 = new int[3];
is the simplest way to declare a one-dimensional array in the Java language. First, write the data type we want to make an array, as int
is the datatype. Now proceed to add square brackets []
after the datatype name, which means that we have declared an array of integers whose size is not defined. Give a variable name after datatype name as intArr1
. Moving towards the right, put a new
keyword after the =
operator. Keyword new
states instantiate an object and allocate the memory to it in the heap area. It proceeds with a constructor call of datatype given initially. Since the array is to be declared, the constructor takes the array size in square brackets. The =
operator assigns the object created to the reference variable. So an integer array of size 3
is declared with the variable as intArr1
.
int[] intArr2 = {3, 4, 5};
is another way of declaring and instantiating the values in one place. In this case, we give data type and variable name on the left side of the =
operator. To the right of it, we are directly providing values in curly braces {}
. In this case, the size of an array gets evaluated internally by the number of values declared. So an integer array of size 3
is created with values as 3,4, and 5
at index 0,1,2
respectively. Consider, we do not give any data to an array while instantiation, then the array is initialized with its default values.
int[] intArr3 = new int[]{3, 4, 5};
is in all ways similar to the second one but is quite lengthy. Here the declaration of an array is the same as the first way. The only difference is, we do not give size explicitly, as while defining values in {}
curly braces, the size gets calculated implicitly. So an array of integers with size 3
and initial values are also initialized.
The next method is int intArr4[] = IntStream.range(0, 10).toArray();
. In this way, we are using Java 8
and its functionalities to create and initialize an array of the desired type. The prototype of the declaration on the left-hand side and is different. It is another way to declare an array where square brackets are along with the variable name. So intArr4[]
is a valid name. Proceeding ahead, IntStream
is an interface given in the java.util.Stream
package. The method range
is a static factory method in the IntStream
interface and returns the IntStream
of integer values. It takes two values as parameters that define the inclusive lower bound and exclusive upper bound of an array. Lastly, the generated integer stream gets converted to an array using the toArray
function, and this is a terminal operator.
int [] intArr5 = IntStream.of(2, 5, 3, 8, 1, 9, 7).toArray();
represents one more way of declaring an array. And this says putting a space between datatype and square brackets is valid. In Java 8, we can use the of()
function to define a stream of integer values. The specified values are stored sequentially in the integer Stream. This stream is then collected to an array using the toArray
function.
Declare a 2D Array in Java
A two-dimensional array is a combination of rows and columns altogether in a single unit. The declaration of the 2D array includes the definition of rows and columns sequentially. The first value defines the number of rows, and the second value the number of columns.
int intArr7[][] = new int[5][2];
and int[] intArr8[] = new int[5][2];
are valid declarations of an array that has size 5 x 2, where 5 is the number of rows, and 2 is the number of columns.
int[][] intArr9={ {1,2}, {1,2}, {1,2}, {1,2}, {1,2} };
is a way of defining a 2D array where we don’t provide explicitly. The size is calculated internally by the number of parameters in curly braces. And the nesting of curly braces says values are to get inserted in row1 and proceeding by comma-separated values ahead.
Rashmi is a professional Software Developer with hands on over varied tech stack. She has been working on Java, Springboot, Microservices, Typescript, MySQL, Graphql and more. She loves to spread knowledge via her writings. She is keen taking up new things and adopt in her career.
LinkedIn