How to Convert Stream to Array in Java
-
Using the
convertStreamToArray()
Method to Convert Stream to Array in Java -
Use the
toArray()
Method to Convert Stream to Array in Java -
Using the
mapToInt()
Method
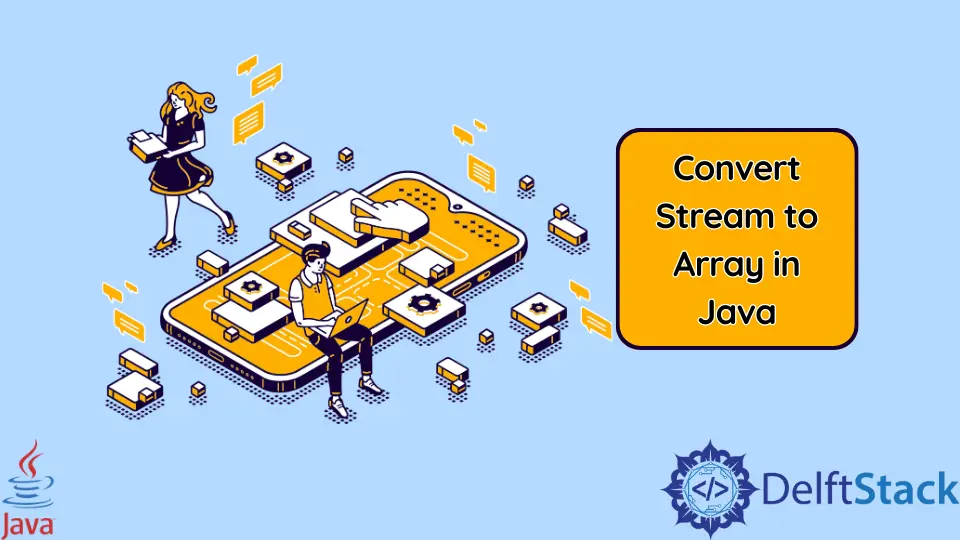
A stream is a collection of objects that supports many methods and can be customized to produce our desired results.
On the other hand, an array is a collection of the same data type variables declared with the same name. You can define an array either as the primitive data type or an object of a class.
If you define the array as a primitive data type, actual values will store in a separate memory location. Conversely, if you define the array as an object of a class, the actual Object will store in a heap segment.
Using the convertStreamToArray()
Method to Convert Stream to Array in Java
The stream comes with the convertStreamtoArray()
method that returns an array along with an element of the stream in an object array.
Algorithm
- Create a stream of integers first.
- Convert a stream of integers into an array using the method
Stream.toArray()
. - The returned array is of type
Object[]
. - Return that array object in the last.
Program
import java.util.*;
import java.util.stream.*;
public class sampleArticle {
// Function to convert Stream to Array
public static <D> Object[] convertStreamToArray(Stream<D> stream) {
return stream.toArray();
}
public static void main(String args[]) {
// Create a stream of integers
Stream<Integer> stream = Stream.of(2, 4, 6, 8, 10);
// Convert Stream to array
Object[] array = convertStreamToArray(stream);
// Print the array of stream
System.out.println("Array from Stream: " + Arrays.toString(array));
}
}
Output:
Array from Stream: [2,4,6,8,10]
Use the toArray()
Method to Convert Stream to Array in Java
Another pre-defined java method to convert Java stream into an array is toArray()
. This method returns an array that contains elements of the stream. It uses the supplied generator function to allocate space to the returned array and any other arrays required for the partitioned execution of a function.
Algorithm
- Create the stream.
- Using the method
toArray()
to convert the stream into an array. You need to pass theObject []: new
as the generator function to allocate space for the returned array to get this job done. - Array obtained via this process is of type
Object[]
. - Return the Object
Array[]
in the last.
Program
import java.util.*;
import java.util.stream.*;
public class sampleArticle {
// defining function that converts stream into an array
public static <D> Object[] convertStreamToArray(Stream<D> stream) {
return stream.toArray(Object[] ::new);
}
public static void main(String args[]) {
// Create a stream of integers
Stream<Integer> stream1 = Stream.of(2, 4, 6, 8, 10);
// Convert Stream to array
Object[] array = convertStreamToArray(stream1);
// Print the array of stream
System.out.println("Converted array from Stream: " + Arrays.toString(array));
}
}
Output:
Converted array from Stream: [2,4,6,8,10]
Using the mapToInt()
Method
Java 8 Stream comes with the mapToInt()
method that returns an integer stream containing elements of the given stream. It is an intermediary process. The intStream thus obtained is then converted into an integer array (int []
) using the toArray()
method.
Algorithm
- Create a stream of integers
- Convert stream of integers into an integer Stream (intStream) using the
Stream.mapToInt()
method. - Convert the resultant stream into an integer array (
int []
) using thetoArray()
method. - The array obtained through this method is of type integer.
- Return the integer array (
int []
) in the last.
Program
import java.util.*;
import java.util.stream.*;
public class sampleArticle {
// defining function that converts stream into an array
public static int[] convertStreamToArray(Stream<Integer> stream) {
return stream.mapToInt(Integer::intValue).toArray();
}
public static void main(String args[]) {
// Create a stream of integers
Stream<Integer> stream1 = Stream.of(2, 4, 6, 8, 10);
// Convert Stream to array
int[] array = convertStreamToArray(stream1);
// Print the array of stream
System.out.println("Converted array from Stream: " + Arrays.toString(array));
}
}
Output:
Converted array from Stream: [2,4,6,8,10]
Related Article - Java Stream
- How to Convert Iterable to Stream in Java
- How to Distinct by Property in Java 8 Stream
- How to Convert Stream Element to Map in Java
- BiFunction Interface in Java
- The Stream Reduce Operation in Java