The Stream Reduce Operation in Java
- What is Reduction in Java
-
Stream
reduce()
Operation in Java 8 -
Finding the Sum Using
reduce()
Operation in Java -
Finding the Sum Using
reduce()
and Method Reference in Java -
Finding Min and Max Using
reduce()
Operation in Java - Conclusion
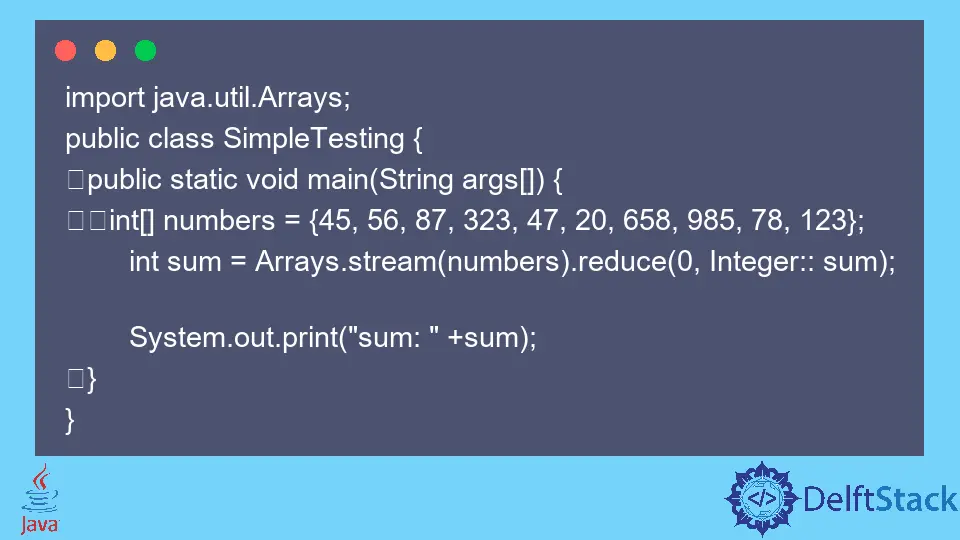
This tutorial will discuss the reduce()
operation detail and discuss some of its examples. Before discussing the reduce()
operation. Let us first discuss the reduction.
What is Reduction in Java
Many terminal operations (such as average, sum, min, max, and count) in the JDK combine the contents of a stream to output a single value. The reduction operation does the same and returns a single stream.
In addition, the JDK includes reduction operations that return a collection rather than a single value. Many reduction processes accomplish a specific job, such as calculating the average of values or categorizing items.
The JDK, on the other hand, has general-purpose reduction operations known as reducing and collecting.
Stream reduce()
Operation in Java 8
The reduce()
operation is a general-purpose reduction operation. The syntax of the reduce()
operation is:
T reduce(T identity, BinaryOperator<T> accumulator)
There are two arguments to the reduction operation:
identity
: Theidentity
element is both the reduction’s beginning value and the default outcome if the stream contains no elements.accumulator
: Theaccumulator
function accepts two arguments: a partial reduction result and the stream’s next element. It gives us a new partial result.
The reduce()
operation is a type of terminal operation.
Finding the Sum Using reduce()
Operation in Java
Let us calculate the sum of all the elements of an array using Stream API in Java. Look at the code below:
import java.util.Arrays;
public class SimpleTesting {
public static void main(String args[]) {
int[] numbers = {45, 56, 87, 323, 47, 20, 658, 985, 78, 123};
int sum = Arrays.stream(numbers).reduce(0, (a, b) -> a + b);
System.out.print("sum: " + sum);
}
}
Output:
sum: 2422
If we don’t use the reduce()
function, we will have to write the code below to get the sum of all the elements. See the example below.
import java.util.Arrays;
public class SimpleTesting {
public static void main(String args[]) {
int[] numbers = {45, 56, 87, 323, 47, 20, 658, 985, 78, 123};
int sum = 0;
for (int i : numbers) {
sum += i;
}
System.out.println("sum : " + sum);
}
}
Output:
sum : 2422
Finding the Sum Using reduce()
and Method Reference in Java
We can also pass the sum()
method reference Integer::sum
as follows to get the sum of all the elements. Look at the code below:
import java.util.Arrays;
public class SimpleTesting {
public static void main(String args[]) {
int[] numbers = {45, 56, 87, 323, 47, 20, 658, 985, 78, 123};
int sum = Arrays.stream(numbers).reduce(0, Integer::sum);
System.out.print("sum: " + sum);
}
}
Output:
sum: 2422
If the array is empty and we pass it to get the sum of elements, then it does not throw any exception instead gives a zero result. See the example below.
import java.util.Arrays;
public class SimpleTesting {
public static void main(String args[]) {
int[] numbers = {};
int sum = Arrays.stream(numbers).reduce(0, Integer::sum);
System.out.print("sum: " + sum);
}
}
Output:
sum: 0
As we can see, the identity element gets returned if the array is empty.
Finding Min and Max Using reduce()
Operation in Java
Generally, to find the min and max element in an array, we use a combination of for
loops and if-else
statements such as:
public class SimpleTesting {
public static void main(String args[]) {
int[] numbers = {45, 56, 87, 323, 47, 20, 658, 985, 78, 123};
// finding the min element
int min = numbers[0];
for (int i : numbers) {
if (min > i) {
min = i;
}
}
// finding the max element
int max = numbers[0];
for (int i : numbers) {
if (max < i) {
max = i;
}
}
// printing the max and min value
System.out.println("max: " + max);
System.out.println("min: " + min);
}
}
Output:
max: 985
min: 20
We can find the min and max element in an array using the reduce()
operation that makes our code more concise. See the example below.
import java.util.Arrays;
public class SimpleTesting {
public static void main(String args[]) {
int[] numbers = {45, 56, 87, 323, 47, 20, 658, 985, 78, 123};
// finding the min element
int min = Arrays.stream(numbers).reduce(Integer.MAX_VALUE, (a, b) -> a < b ? a : b);
// finding the max element
int max = Arrays.stream(numbers).reduce(Integer.MIN_VALUE, (a, b) -> a > b ? a : b);
// printing the max and min value
System.out.println("max: " + max);
System.out.println("min: " + min);
}
}
Output:
max: 985
min: 20
We can also use method references for the calculation of the min and max element from an array. Look at the code below:
import java.util.Arrays;
public class SimpleTesting {
public static void main(String args[]) {
int[] numbers = {45, 56, 87, 323, 47, 20, 658, 985, 78, 123};
// finding the min element
int min = Arrays.stream(numbers).reduce(Integer.MAX_VALUE, Integer::min);
// finding the max element
int max = Arrays.stream(numbers).reduce(Integer.MIN_VALUE, Integer::max);
// printing the max and min value
System.out.println("max: " + max);
System.out.println("min: " + min);
}
}
Output:
max: 985
min: 20
Conclusion
In this tutorial, we learned about the reduction operation. We then dived deep into the topic of the reduce()
operation. Lastly, we saw several examples of how we perform basic arithmetic functions concisely.