How to Convert OutputStream to String in Java
-
Introduction in Converting
OutputStream
toString
-
Convert
OutputStream
toString
in Java -
Best Practices in Converting
OutputStream
to String - Conclusion
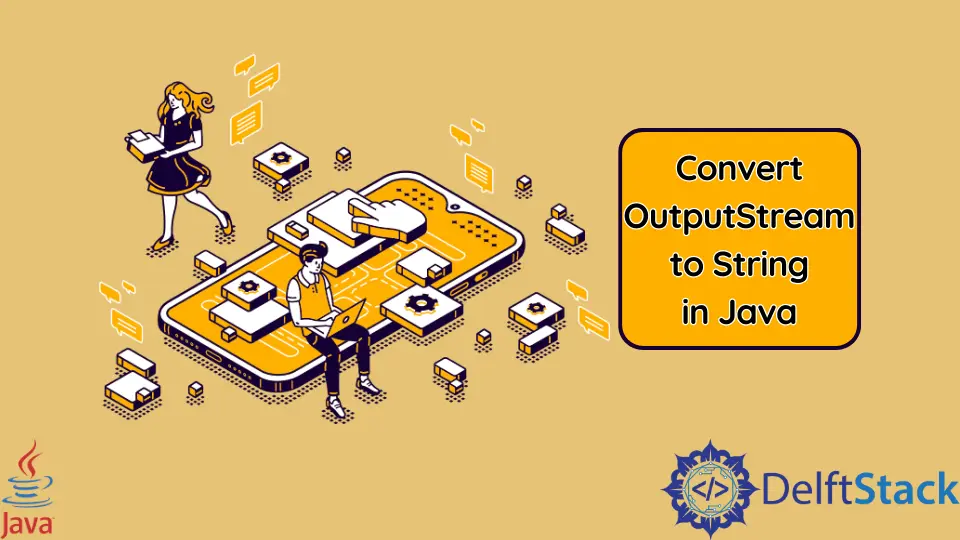
This tutorial demonstrates how to convert OutputStream
to String
in Java.
In Java, various methods, including utilizing ByteArrayOutputStream
with toString()
, the String
constructor, or type casting, provide effective ways to convert an OutputStream
to a String
and adhering to best practices such as character encoding consideration and proper stream handling ensures reliable and efficient code.
Introduction in Converting OutputStream
to String
Converting an OutputStream
to a String
in Java is important for several reasons. It allows developers to transform binary data, typically represented by an OutputStream
, into a human-readable and manipulable text format.
This conversion is particularly useful when dealing with data streams from sources like network connections or file operations, where the content needs interpretation or processing in a textual form. Additionally, converting to a String
facilitates easy integration with other parts of a program that operate with textual data, simplifying downstream operations such as parsing, logging, or displaying information to users.
This conversion process acts as a bridge between the binary nature of output streams and the text-based requirements of many Java applications, enhancing the overall flexibility and usability of the code.
Convert OutputStream
to String
in Java
ByteArrayOutputStream
and toString()
ByteArrayOutputStream
in Java provides a convenient in-memory storage for bytes. When converting an OutputStream
to a String
, using ByteArrayOutputStream
allows efficient accumulation of bytes.
The toString()
method of ByteArrayOutputStream
then simplifies the conversion by providing a direct way to obtain the content as a string. This approach is concise and avoids the need for additional libraries or complex code.
In comparison to other techniques, such as using StringWriter
or libraries like Apache Commons IO, the ByteArrayOutputStream
method is often simpler, offering a straightforward and native solution for converting output streams to strings in Java.
Code Example:
import java.io.ByteArrayOutputStream;
import java.io.IOException;
import java.io.OutputStream;
public class OutputStreamToStringExample {
public static void main(String[] args) {
// Create an example OutputStream
OutputStream outputStream = createExampleOutputStream();
// Convert the OutputStream to a String
String result = convertOutputStreamToString(outputStream);
// Display the original OutputStream and the converted String
System.out.println("Original OutputStream:");
System.out.println(outputStream.toString());
System.out.println("\nConverted String:");
System.out.println(result);
}
private static OutputStream createExampleOutputStream() {
// Create a ByteArrayOutputStream for demonstration
ByteArrayOutputStream byteArrayOutputStream = new ByteArrayOutputStream();
try {
byteArrayOutputStream.write("Hello! This is delftstack.com".getBytes());
} catch (IOException e) {
e.printStackTrace();
}
return byteArrayOutputStream;
}
private static String convertOutputStreamToString(OutputStream outputStream) {
// Use ByteArrayOutputStream's toString() method for conversion
return outputStream.toString();
}
}
The code example follows a simple and concise process for converting an OutputStream
to a String
in Java.
First, an example OutputStream
is created using the createExampleOutputStream
method, which initializes a ByteArrayOutputStream
and writes a string to it. Next, the convertOutputStreamToString
method takes the OutputStream
as input and uses the toString()
method of ByteArrayOutputStream
to perform the conversion, transforming the content of the stream into a string representation.
In the main
method, these methods are invoked, and the original OutputStream
is printed using its toString()
method, showcasing the content before conversion. Subsequently, the converted String
is displayed, illustrating the effectiveness of the custom conversion method.
This approach is both straightforward and efficient, providing a clear way to convert an OutputStream
to a String
in Java.
Output:
In the output, we observe the original content of the OutputStream
and the successfully converted String
, validating the effectiveness of the presented method.
ByteArrayOutputStream
and String
Constructor
ByteArrayOutputStream
and the String
constructor offer a straightforward method for converting an OutputStream
to a String
in Java. With ByteArrayOutputStream
, the stream’s content is accumulated in memory, and the resulting byte array can be converted into a string using the String
constructor.
This approach is simple, direct, and native to Java, eliminating the need for external libraries. In comparison to other methods, like using Scanner
or StringWriter
, the ByteArrayOutputStream
and String
constructor technique is often more concise, providing an efficient and built-in solution for the conversion process.
Code Example:
import java.io.ByteArrayOutputStream;
import java.io.IOException;
import java.io.OutputStream;
public class OutputStreamToStringExample {
public static void main(String[] args) {
// Create an example OutputStream
OutputStream outputStream = createExampleOutputStream();
// Convert the OutputStream to a String
String result = convertOutputStreamToString(outputStream);
// Display the original OutputStream and the converted String
System.out.println("Original OutputStream:");
System.out.println(outputStream.toString());
System.out.println("\nConverted String:");
System.out.println(result);
}
private static OutputStream createExampleOutputStream() {
// Create a ByteArrayOutputStream for demonstration
ByteArrayOutputStream byteArrayOutputStream = new ByteArrayOutputStream();
try {
byteArrayOutputStream.write("Hello! This is delftstack.com".getBytes());
} catch (IOException e) {
e.printStackTrace();
}
return byteArrayOutputStream;
}
private static String convertOutputStreamToString(OutputStream outputStream) {
// Use the String constructor to convert ByteArrayOutputStream to String
return new String(((ByteArrayOutputStream) outputStream).toByteArray());
}
}
In the code example, we follow a simple and concise process to convert an OutputStream
to a String
in Java.
First, the createExampleOutputStream
method initializes a ByteArrayOutputStream
and populates it with the bytes of the string Hello! This is delftstack.com
for demonstration. This serves as our sample OutputStream
.
Next, the magic happens in the convertOutputStreamToString
method, which takes an OutputStream
as a parameter and utilizes the String
constructor. This constructor creates a new String
using the byte array obtained from the ByteArrayOutputStream
.
In the main
method, we invoke these methods, printing the original OutputStream
using its toString()
method to reveal the content before conversion. Subsequently, we showcase the converted String
obtained through our custom conversion method.
This approach is not only straightforward but also efficient, providing a clear and concise way to convert an OutputStream
to a String
in Java.
Output:
The output demonstrates the original content of the OutputStream
and the successfully converted String
, affirming the effectiveness of the presented method.
ByteArrayOutputStream
and TypeCasting
ByteArrayOutputStream
and type casting offer a direct and concise method for converting an OutputStream
to a String
in Java. With ByteArrayOutputStream
, the stream’s content is accumulated in memory, and the resulting byte array can be cast directly to ByteArrayOutputStream
, simplifying the conversion.
This approach is native to Java and eliminates the need for external libraries. In comparison to other methods, such as using StringWriter
or external libraries like Apache Commons IO, the ByteArrayOutputStream
and type casting technique is often simpler, providing an efficient and built-in solution for the conversion process.
Code Example:
import java.io.ByteArrayOutputStream;
import java.io.IOException;
import java.io.OutputStream;
public class OutputStreamToStringExample {
public static void main(String[] args) {
// Create an example OutputStream
OutputStream outputStream = createExampleOutputStream();
// Convert the OutputStream to a String
String result = convertOutputStreamToString(outputStream);
// Display the original OutputStream and the converted String
System.out.println("Original OutputStream:");
System.out.println(outputStream.toString());
System.out.println("\nConverted String:");
System.out.println(result);
}
private static OutputStream createExampleOutputStream() {
// Create a ByteArrayOutputStream for demonstration
ByteArrayOutputStream byteArrayOutputStream = new ByteArrayOutputStream();
try {
byteArrayOutputStream.write("Hello! This is delftstack.com".getBytes());
} catch (IOException e) {
e.printStackTrace();
}
return byteArrayOutputStream;
}
private static String convertOutputStreamToString(OutputStream outputStream) {
// Use type casting to convert OutputStream to ByteArrayOutputStream
ByteArrayOutputStream byteArrayOutputStream = (ByteArrayOutputStream) outputStream;
return byteArrayOutputStream.toString();
}
}
In the provided code example, we utilize a simple and concise approach to convert an OutputStream
to a String
in Java.
The createExampleOutputStream
method sets up a ByteArrayOutputStream
and populates it with the bytes corresponding to the string Hello! This is delftstack.com
. This serves as our sample OutputStream
.
Moving on to the convertOutputStreamToString
method, it takes an OutputStream
as input and uses type casting to convert it to a ByteArrayOutputStream
. This type casting is secure, assuming the actual type of the OutputStream
is indeed a ByteArrayOutputStream
, as demonstrated in the earlier createExampleOutputStream
method.
In the main
method, we invoke these methods to showcase the conversion process. Initially, we print the original OutputStream
using its toString()
method, revealing the content before any conversion.
Subsequently, we highlight the converted String
obtained through type casting. This technique, involving ByteArrayOutputStream
and type casting, offers a direct and efficient solution to convert an OutputStream
to a String
in Java.
Output:
The output demonstrates the original content of the OutputStream
and the successfully converted String
, affirming the effectiveness of the presented method utilizing type casting.
Best Practices in Converting OutputStream
to String
Consideration of Charset
When converting an OutputStream
to a String
in Java, it’s crucial to consider the character encoding used in the conversion. Always specify the appropriate charset to ensure accurate and reliable transformation.
Stream Closing
Ensure proper handling of resource management by closing the OutputStream
after conversion to prevent potential resource leaks. Utilize try-with-resources or explicitly close the stream in a final block.
Exception Handling
Implement robust exception handling to manage potential errors during the conversion process. IOExceptions
may occur, especially when dealing with IO operations, and handling them gracefully enhances the reliability of your code.
ByteArrayOutputStream
Size Initialization
If using ByteArrayOutputStream
, consider initializing it with an initial size if the approximate size of the data is known. This can help optimize memory usage and improve performance.
Validate InputStream
Source
When working with an OutputStream
obtained from an InputStream
, ensure that the source InputStream
is properly initialized and contains valid data. Validate the InputStream
before attempting the conversion.
Reusable Conversion Methods
Consider encapsulating the conversion logic into reusable methods or utility classes. This promotes code reusability and helps maintain a clean and modular codebase.
Use Standard Libraries:
Leverage standard libraries like Apache Commons IO or java.nio.charset.StandardCharsets for common conversion tasks. These libraries often provide efficient and well-tested methods for working with streams and strings.
Documentation
Document your code to provide clear information about the purpose and usage of the conversion methods. Include details about the expected input, output, and any potential exceptions that may be thrown.
Testing and Validation
Thoroughly test the conversion logic with various scenarios, including edge cases and unexpected inputs. Validate that the converted strings match the expected results.
Memory Considerations
Be mindful of memory usage, especially when dealing with large data sets. Evaluate the impact on memory consumption and optimize your code accordingly, considering the scalability of your application.
Performance Optimization
Explore performance optimization techniques based on your specific use case. For instance, if dealing with large files, consider using buffered streams or parallel processing to enhance conversion speed.
Cross-Platform Compatibility
Ensure that the charset used for conversion is compatible across different platforms. Consider using standard charsets to minimize compatibility issues between different systems.
Code Review
Conduct code reviews to get insights from peers regarding the efficiency, readability, and adherence to best practices in your conversion code. Collaborative feedback can lead to improvements and better maintainability.
Conclusion
Converting an OutputStream
to a String
in Java using techniques like ByteArrayOutputStream
and the toString()
method, String
constructor, or type casting offers straightforward and efficient solutions. These methods are invaluable when dealing with data streams, allowing seamless transitions between different data representations.
The provided examples highlight the simplicity and power of each approach, demonstrating their applicability in real-world scenarios.
When performing such conversions, it’s essential to follow best practices, including considering character encoding, closing streams appropriately, and implementing robust exception handling. These practices ensure reliability, maintainability, and optimal performance in Java code.
Sheeraz is a Doctorate fellow in Computer Science at Northwestern Polytechnical University, Xian, China. He has 7 years of Software Development experience in AI, Web, Database, and Desktop technologies. He writes tutorials in Java, PHP, Python, GoLang, R, etc., to help beginners learn the field of Computer Science.
LinkedIn Facebook